
Example program to test AES-GCM functionality. Used for a workshop
aesni.h File Reference
AES-NI for hardware AES acceleration on some Intel processors. More...
Go to the source code of this file.
Functions | |
int | aesni_supports (unsigned int what) |
AES-NI features detection routine. | |
int | aesni_crypt_ecb (aes_context *ctx, int mode, const unsigned char input[16], unsigned char output[16]) |
AES-NI AES-ECB block en(de)cryption. | |
void | aesni_gcm_mult (unsigned char c[16], const unsigned char a[16], const unsigned char b[16]) |
GCM multiplication: c = a * b in GF(2^128) | |
void | aesni_inverse_key (unsigned char *invkey, const unsigned char *fwdkey, int nr) |
Compute decryption round keys from encryption round keys. | |
int | aesni_setkey_enc (unsigned char *rk, const unsigned char *key, size_t bits) |
Perform key expansion (for encryption) |
Detailed Description
AES-NI for hardware AES acceleration on some Intel processors.
Copyright (C) 2013, Brainspark B.V.
This file is part of PolarSSL (http://www.polarssl.org) Lead Maintainer: Paul Bakker <polarssl_maintainer at polarssl.org>
All rights reserved.
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file aesni.h.
Function Documentation
int aesni_crypt_ecb | ( | aes_context * | ctx, |
int | mode, | ||
const unsigned char | input[16], | ||
unsigned char | output[16] | ||
) |
void aesni_gcm_mult | ( | unsigned char | c[16], |
const unsigned char | a[16], | ||
const unsigned char | b[16] | ||
) |
void aesni_inverse_key | ( | unsigned char * | invkey, |
const unsigned char * | fwdkey, | ||
int | nr | ||
) |
int aesni_setkey_enc | ( | unsigned char * | rk, |
const unsigned char * | key, | ||
size_t | bits | ||
) |
Generated on Tue Jul 12 2022 19:40:21 by
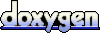