imu01c
LSM303D Class Reference
Tilt-compensated compass interface Library for the STMicro LSM303D 3-axis magnetometer, 3-axis acceleromter. More...
#include <LSM303D.h>
Public Member Functions | |
LSM303D (PinName sda, PinName scl) | |
Create a new interface for an LSM303D. | |
void | setOffset (float x, float y, float z) |
sets the x, y, and z offset corrections for hard iron calibration | |
void | setScale (float x, float y, float z) |
sets the scale factor for the x, y, and z axes | |
void | read () |
read the raw accelerometer and compass values calc Heading and Pitch | |
void | set_limits (int mode) |
Set Mag Limits. | |
void | frequency (int hz) |
sets the I2C bus frequency | |
Data Fields | |
vector | acc_raw |
ACC Raw readings with x,y and z axis. | |
vector | mag_raw |
MAG Raw readings with x,y and z axis. | |
vector | acc |
ACC Filtert readings with x,y and z axis. | |
vector | mag |
MAG Normal readings with x,y and z axis. | |
float | hdg |
Heading. | |
float | pitch |
Pitch. | |
vector | min |
MAG Minimal readings. | |
vector | max |
MAG Maximal readings. | |
vector | spreed |
MAG Min-Max estimated range. |
Detailed Description
Tilt-compensated compass interface Library for the STMicro LSM303D 3-axis magnetometer, 3-axis acceleromter.
Based on
Michael Shimniok http://bot-thoughts.com
test program by tosihisa and
Pololu sample library for LSM303DLH breakout by ryantm:
Copyright (c) 2011 Pololu Corporation. For more information, see
http://www.pololu.com/ http://forum.pololu.com/
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
#include "mbed.h" #include "LSM303D.h" Serial debug(USBTX,USBRX); LSM303D compass(p28, p27); int main() { int count; debug.baud(115200); debug.printf("LSM303D Test\x0d\x0a"); compass.setOffset(460, 610, -290); compass.setScale(0.93, 0.92, 1.00); while(1) { compass.read(); count++; if (count%10==0) debug.printf("Heading: %5.1f\n\r Pitch: %4.1f\n\r",compass.hdg, compass.pitch ); wait(0.050); } }
Definition at line 72 of file LSM303D.h.
Constructor & Destructor Documentation
LSM303D | ( | PinName | sda, |
PinName | scl | ||
) |
Create a new interface for an LSM303D.
- Parameters:
-
sda is the pin for the I2C SDA line scl is the pin for the I2C SCL line
Definition at line 88 of file LSM303D.cpp.
Member Function Documentation
void frequency | ( | int | hz ) |
sets the I2C bus frequency
- Parameters:
-
frequency is the I2C bus/clock frequency, either standard (100000) or fast (400000)
Definition at line 280 of file LSM303D.cpp.
void read | ( | ) |
read the raw accelerometer and compass values calc Heading and Pitch
Definition at line 186 of file LSM303D.cpp.
void set_limits | ( | int | mode ) |
Set Mag Limits.
Definition at line 254 of file LSM303D.cpp.
void setOffset | ( | float | x, |
float | y, | ||
float | z | ||
) |
sets the x, y, and z offset corrections for hard iron calibration
Calibration details here: http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/
If you gather raw magnetometer data and find, for example, x is offset by hard iron by -20 then pass +20 to this member function to correct for hard iron.
- Parameters:
-
x is the offset correction for the x axis y is the offset correction for the y axis z is the offset correction for the z axis
Definition at line 147 of file LSM303D.cpp.
void setScale | ( | float | x, |
float | y, | ||
float | z | ||
) |
sets the scale factor for the x, y, and z axes
Calibratio details here: http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/
Sensitivity of the three axes is never perfectly identical and this function can help to correct differences in sensitivity. You're supplying a multipler such that x, y and z will be normalized to the same max/min values
Definition at line 154 of file LSM303D.cpp.
Field Documentation
Generated on Tue Jul 12 2022 17:15:10 by
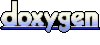