PinDetect rework, removed the continuous sampling, using just irq, timers and pin state sequence.
Fork of PinDetect by
PinDetect Class Reference
PinDetectMod is a rework of PinDetect, . More...
#include <PinDetectMod.h>
Public Member Functions | |
PinDetect (PinName p, PinMode m, int assertvalue, unsigned int debounce_us=5000, unsigned int held_us=2000000) | |
PinDetect constructor. | |
~PinDetect () | |
PinDetect destructor. | |
void | disable () |
Disable. | |
void | enable () |
Enable. | |
void | setDebounceTime (unsigned int dbtime) |
Set debounce time. | |
void | setHeldTime (unsigned int htime) |
Set time until held assumed. | |
void | attach_asserted (void(*function)(void)) |
Attach a callback function. | |
template<typename T > | |
void | attach_asserted (T *object, void(T::*member)(void)) |
Attach a callback object/method. | |
void | attach_deasserted (void(*function)(void)) |
Attach a callback function. | |
template<typename T > | |
void | attach_deasserted (T *object, void(T::*member)(void)) |
Attach a callback object/method. | |
void | attach_asserted_held (void(*function)(void)) |
Attach a callback function. | |
template<typename T > | |
void | attach_asserted_held (T *object, void(T::*member)(void)) |
Attach a callback object/method. | |
void | attach_deasserted_held (void(*function)(void)) |
Attach a callback function. | |
template<typename T > | |
void | attach_deasserted_held (T *object, void(T::*member)(void)) |
Attach a callback object/method. | |
operator int () | |
operator int() | |
unsigned int | state () |
Get the current pin state. | |
void | statereset () |
Reset pinstate to S_IDLE and reset debounce/held timers. | |
unsigned int | getbouncein () |
Get the total bounces for deasserted->asserted. | |
unsigned int | getbounceout () |
Get the total bounces for asserted->deasserted. |
Detailed Description
PinDetectMod is a rework of PinDetect, .
Unlike the original PinDetect which samples pin at specified rate, here is all about interrupts and timers, because for some application the continuous sampling of a mostly idle pin is an overhead. Pin state is evaluated in sequence deasserted->ringing->asserted->(->held_asserted)->ringing->deasserted callback function can be attached to these state changes: deasserted->asserted (e.g. as soon as button is pressed) asserted->deasserted (e.g quick button pulse) asserted->held_asserted (e.g. as soon as the button is evaluated to be held) held_asserted->deasserted (e.g. long button pulse)
Only callbacks that have been attached will be called by the library.
Example:
#include "mbed.h" #include "PinDetectMod.h" DigitalOut led1( LED1 ); PinDetect pin(p30, PullUp, 0); void keyPressed( void ) { backligh_on(); } void keyQuickPulsed( void ) { increasevalue(); } void keyPressedHeld( void ) { beep(); } void keyLongPulsed( void ) { setup_menu(); } int main() { pin.attach_asserted( &keyPressed ); pin.attach_deasserted( &keyQuickPulsed ); pin.attach_asserted_held( &keyPressedHeld ); pin.attach_deasserted_held( &keyLongPulsed ); while( 1 ) { led1 = !led1; wait( 0.2 ); } }
Definition at line 101 of file PinDetectMod.h.
Constructor & Destructor Documentation
PinDetect | ( | PinName | p, |
PinMode | m, | ||
int | assertvalue, | ||
unsigned int | debounce_us = 5000 , |
||
unsigned int | held_us = 2000000 |
||
) |
PinDetect constructor.
- See also:
- http://mbed.org/handbook/DigitalIn
- Parameters:
-
p PinName is a valid pin that supports DigitalIn m PinMode (PullUp, PullDown, PullNone....) assertvalue pin state to be considered as asserted (0 or 1) debounce_us debounce time in microseconds, default 5000 held_us time in microseconds for the state to be considered held_asserted, default 2000000
Definition at line 116 of file PinDetectMod.h.
~PinDetect | ( | ) |
PinDetect destructor.
Definition at line 139 of file PinDetectMod.h.
Member Function Documentation
void attach_asserted | ( | void(*)(void) | function ) |
Attach a callback function.
DigitalOut led1( LED1 ); PinDetect pin(p30, PullUp, 0); void myCallback( void ) { led1 = 1; }; main() { pin.attach_asserted( &myCallback ); }
Call this function when a pin is asserted.
- Parameters:
-
function A C function pointer
Definition at line 192 of file PinDetectMod.h.
void attach_asserted | ( | T * | object, |
void(T::*)(void) | member | ||
) |
Attach a callback object/method.
class Bar { public: void myCallback( void ) { led1 = 1; } }; DigitalOut led1( LED1 ); PinDetect pin(p30, PullUp, 0); Bar bar; main() { pin.attach_asserted( &bar, &Bar::myCallback ); }
Call this function when a pin is asserted.
- Parameters:
-
object An object that conatins the callback method. method The method within the object to call.
Definition at line 220 of file PinDetectMod.h.
void attach_asserted_held | ( | void(*)(void) | function ) |
Attach a callback function.
DigitalOut led2( LED2 ); PinDetect pin(p30, PullUp, 0); void myCallback( void ) { led2 = 1; }; main() { pin.attach_asserted_held( &myCallback ); }
Call this function when a pin is asserted and held.
- Parameters:
-
function A C function pointer
Definition at line 296 of file PinDetectMod.h.
void attach_asserted_held | ( | T * | object, |
void(T::*)(void) | member | ||
) |
Attach a callback object/method.
class Bar { public: void myCallback( void ) { led2 = 0; } }; DigitalOut led2( LED2 ); PinDetect pin(p30, PullUp, 0); Bar bar; main() { pin.attach_asserted_held( &bar, &Bar::myCallback ); }
Call this function when a pin is asserted and held.
- Parameters:
-
object An object that conatins the callback method. method The method within the object to call.
Definition at line 324 of file PinDetectMod.h.
void attach_deasserted | ( | T * | object, |
void(T::*)(void) | member | ||
) |
Attach a callback object/method.
class Bar { public: void myCallback( void ) { led1 = 0; } }; DigitalOut led1( LED1 ); PinDetect pin(p30, PullUp, 0); Bar bar; main() { pin.attach_deasserted( &bar, &Bar::myCallback ); }
Call this function when a pin is deasserted (short pulse).
- Parameters:
-
object An object that conatins the callback method. method The method within the object to call.
Definition at line 272 of file PinDetectMod.h.
void attach_deasserted | ( | void(*)(void) | function ) |
Attach a callback function.
DigitalOut led1( LED1 ); PinDetect pin(p30, PullUp, 0); void myCallback( void ) { led1 = 0; }; main() { pin.attach_deasserted( &myCallback ); }
Call this function when a pin is deasserted (short pulse).
- Parameters:
-
function A C function pointer
Definition at line 244 of file PinDetectMod.h.
void attach_deasserted_held | ( | void(*)(void) | function ) |
Attach a callback function.
DigitalOut led3( LED3 ); PinDetect pin(p30, PullUp, 0); void myCallback( void ) { led3 = 1; }; main() { pin.attach_deasserted_held( &myCallback ); }
Call this function when a pin is deasserted after being held.
- Parameters:
-
function A C function pointer
Definition at line 348 of file PinDetectMod.h.
void attach_deasserted_held | ( | T * | object, |
void(T::*)(void) | member | ||
) |
Attach a callback object/method.
class Bar { public: void myCallback( void ) { led3 = 0; } }; DigitalOut led3( LED3 ); PinDetect pin(p30, PullUp, 0); Bar bar; main() { pin.attach_deasserted_held( &bar, &Bar::myCallback ); }
Call this function when a pin is deasserted after being held.
- Parameters:
-
object An object that conatins the callback method. method The method within the object to call.
Definition at line 376 of file PinDetectMod.h.
void disable | ( | ) |
Disable.
Definition at line 146 of file PinDetectMod.h.
void enable | ( | ) |
Enable.
Definition at line 153 of file PinDetectMod.h.
unsigned int getbouncein | ( | ) |
Get the total bounces for deasserted->asserted.
- Returns:
- bounces so far
Definition at line 408 of file PinDetectMod.h.
unsigned int getbounceout | ( | ) |
Get the total bounces for asserted->deasserted.
- Returns:
- bounces so far
Definition at line 416 of file PinDetectMod.h.
operator int | ( | ) |
operator int()
Read the value of the pin being sampled.
Definition at line 384 of file PinDetectMod.h.
void setDebounceTime | ( | unsigned int | dbtime ) |
void setHeldTime | ( | unsigned int | htime ) |
Set time until held assumed.
- Parameters:
-
htime microseconds
Definition at line 169 of file PinDetectMod.h.
unsigned int state | ( | ) |
Get the current pin state.
- Returns:
- pinstate bitmask S_HELD|S_ASSERTED|S_RINGING, 0=S_IDLE
Definition at line 390 of file PinDetectMod.h.
void statereset | ( | ) |
Reset pinstate to S_IDLE and reset debounce/held timers.
Definition at line 397 of file PinDetectMod.h.
Generated on Wed Jul 13 2022 10:41:18 by
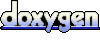