A library to manipulate 2D arrays, and output them to an LED Dot Matrix Display. The display must be wired up using a shift register combined with a nor latch.
LEDMatrix.h
00001 #include "mbed.h" 00002 #include "Matrix.h" 00003 00004 template <int WIDTH, int HEIGHT> class LEDMatrix : public Matrix<bool> { 00005 private: 00006 //Array of row pins 00007 DigitalOut* _rows; 00008 00009 //Pointers to column control pins 00010 DigitalOut* _nextCol; 00011 DigitalOut* _firstCol; 00012 int _usPerColumn; 00013 00014 public: 00015 LEDMatrix(DigitalOut rows[HEIGHT], DigitalOut* nextCol, DigitalOut* firstCol, int usPerFrame) : Matrix<bool>(WIDTH, HEIGHT), _rows(rows), _nextCol(nextCol), _firstCol(firstCol) { 00016 clear(); 00017 _usPerColumn = usPerFrame / WIDTH; 00018 } 00019 00020 void redraw() { 00021 //Pulse firstCol 00022 *_firstCol = 1; 00023 wait_us(10); 00024 *_firstCol = 0; 00025 00026 //Account for reverse column ordering 00027 for (int col = WIDTH - 1; col >= 0; col--) { 00028 //Pulse nextCol 00029 *_nextCol = 1; 00030 wait_us(10); 00031 *_nextCol = 0; 00032 00033 //Output a column of data to the row pins. 00034 for(int row = 0; row < HEIGHT; row++) { 00035 _rows[row] = (*this)(col, row); 00036 } 00037 00038 //Wait 00039 wait_us(_usPerColumn); 00040 00041 //Clear the column, ready for the next iteration 00042 for(int i = 0; i < HEIGHT; i++) { 00043 _rows[i] = false; 00044 } 00045 } 00046 } 00047 };
Generated on Tue Jul 19 2022 21:46:55 by
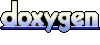