
A Bitmap library created a while back. Probably still works...
Embed:
(wiki syntax)
Show/hide line numbers
BitmapFile.cpp
00001 #include "BitmapFile.h" 00002 00003 00004 BitmapFile::BitmapFile(char* fname) : m_pFile(NULL) 00005 { 00006 m_fileName = fname; 00007 Initialize(); 00008 } 00009 00010 BitmapFile::~BitmapFile() 00011 { 00012 delete[] m_fileName; 00013 } 00014 00015 bool BitmapFile::Initialize() 00016 { 00017 bool success = true; 00018 open(); 00019 fread(&BMPHeader,sizeof(BMPHeader),1,m_pFile); 00020 success = (BMPHeader.b == 'B' && BMPHeader.m == 'M'); 00021 00022 fread(&m_headerlength,sizeof(m_headerlength),1,m_pFile); 00023 fread(&DIBHeader,m_headerlength,1,m_pFile); 00024 00025 /*Debugging code 00026 00027 Serial pc2(USBTX,USBRX); 00028 00029 pc2.printf("\n\rFile = %s", m_fileName); 00030 00031 pc2.printf("\n\rBMPHeader - Size = %d:\n\r",sizeof(BMPHeader)); 00032 pc2.printf("\tbm:\t\t%c%c\n\r",BMPHeader.b,BMPHeader.m); 00033 pc2.printf("\tfilesize:\t%d\n\r",BMPHeader.filesize); 00034 pc2.printf("\treserved:\t%d,%d\n\r",BMPHeader.reserved1,BMPHeader.reserved2); 00035 pc2.printf("\toffset:\t\t%d\n\r",BMPHeader.offset); 00036 00037 pc2.printf("\n\rDIBHeader - Size = %d:\n\r",sizeof(DIBHeader)); 00038 pc2.printf("\theaderLength:\t%d\n\r",DIBHeader.headerLength); 00039 pc2.printf("\theight:\t\t\t%d\n\r",DIBHeader.height); 00040 pc2.printf("\twidth:\t\t%d\n\r",DIBHeader.width); 00041 pc2.printf("\tcplanes:\t\t%d\n\r",DIBHeader.cplanes); 00042 pc2.printf("\tcolordepth:\t\t%d\n\r",DIBHeader.colordepth); 00043 pc2.printf("\tcompression:\t%d\n\r",DIBHeader.compression); 00044 pc2.printf("\tdatasize:\t\t%d\n\r",DIBHeader.datasize); 00045 pc2.printf("\tvres:\t\t%d\n\r",DIBHeader.vres); 00046 pc2.printf("\thres:\t\t%d\n\r",DIBHeader.hres); 00047 00048 00049 */ 00050 00051 m_rowsize = 4*((getColorDepth()*getWidth()+31)/32); 00052 00053 close(); 00054 return success; 00055 } 00056 00057 void BitmapFile::open() 00058 { 00059 if(m_pFile==NULL) 00060 { 00061 m_pFile = fopen(m_fileName, "r"); 00062 } 00063 } 00064 00065 void BitmapFile::close() 00066 { 00067 if(m_pFile!=NULL) 00068 { 00069 fclose(m_pFile); 00070 m_pFile = NULL; 00071 } 00072 } 00073 00074 /**********************************************************/ 00075 /*BMP Header Gets */ 00076 /**********************************************************/ 00077 00078 int BitmapFile::getFileSize() 00079 { 00080 return BMPHeader.filesize; 00081 } 00082 00083 int BitmapFile::getReserved1() 00084 { 00085 return BMPHeader.reserved1; 00086 } 00087 00088 int BitmapFile::getReserved2() 00089 { 00090 return BMPHeader.reserved2; 00091 } 00092 00093 int BitmapFile::getOffset() 00094 { 00095 return BMPHeader.offset; 00096 } 00097 00098 /**********************************************************/ 00099 /*DIB Header Gets */ 00100 /**********************************************************/ 00101 00102 int BitmapFile::getHeaderType() 00103 { 00104 return m_headerlength; 00105 } 00106 00107 int BitmapFile::getHeight() 00108 { 00109 return DIBHeader.height; 00110 } 00111 00112 int BitmapFile::getWidth() 00113 { 00114 return DIBHeader.width; 00115 } 00116 00117 int BitmapFile::getCPlanes() 00118 { 00119 return DIBHeader.cplanes; 00120 } 00121 00122 int BitmapFile::getColorDepth() 00123 { 00124 return DIBHeader.colordepth; 00125 } 00126 00127 int BitmapFile::getCompression() 00128 { 00129 return DIBHeader.compression; 00130 } 00131 00132 int BitmapFile::getDataSize() 00133 { 00134 return DIBHeader.datasize; 00135 } 00136 00137 int BitmapFile::getHRes() 00138 { 00139 return DIBHeader.hres; 00140 } 00141 00142 int BitmapFile::getVRes() 00143 { 00144 return DIBHeader.vres; 00145 } 00146 00147 int BitmapFile::getNumPaletteColors() 00148 { 00149 return DIBHeader.numpalettecolors; 00150 } 00151 00152 int BitmapFile::getImportantColors() 00153 { 00154 return DIBHeader.importantcolors; 00155 } 00156 00157 /**********************************************************/ 00158 /*Data Gets */ 00159 /**********************************************************/ 00160 00161 int BitmapFile::getRowSize() 00162 { 00163 return m_rowsize; 00164 } 00165 00166 int BitmapFile::getPixel(int row, int col, bool closefile) 00167 { 00168 int color = -1; 00169 if(row>=0 && row < getHeight() && col>=0 && col< getWidth()) 00170 { 00171 if(getColorDepth() == 24) 00172 { 00173 open(); 00174 color = 0; //make sure the last byte is 00 00175 00176 int index = getOffset(); 00177 index += col*3; 00178 index += row*4*ceil(getWidth()*3/4.0); 00179 fseek(m_pFile, index, SEEK_SET); 00180 00181 fread (&color, 3,1,m_pFile); 00182 00183 if(closefile) 00184 { 00185 close(); 00186 } 00187 } 00188 } 00189 return color; 00190 } 00191 00192 int *BitmapFile::getRow(int row, bool closefile) 00193 { 00194 open(); 00195 int *colors = new int[getWidth()]; 00196 int index = getOffset() + m_rowsize*row; 00197 fseek(m_pFile, index, SEEK_SET); 00198 if(getColorDepth() == 24) 00199 { 00200 for(int i=0; i<getWidth(); i++) 00201 { 00202 fread(&colors[i],3,1,m_pFile); 00203 } 00204 } 00205 else if(getColorDepth() == 1) 00206 { 00207 char *temp = new char[m_rowsize]; 00208 for(int i=0; i<m_rowsize; i++) 00209 { 00210 fread(&temp[i],sizeof(char),1,m_pFile); 00211 } 00212 for(int i=0; i<getWidth(); i++) 00213 { 00214 int byte = i / 8; 00215 int bit = i % 8; 00216 colors[i] = ((temp[byte] << bit) & 0x80) ? 0xFFFFFF : 0x000000; 00217 } 00218 delete [] temp; 00219 } 00220 if(closefile) 00221 { 00222 close(); 00223 } 00224 return colors; 00225 } 00226 00227 int *BitmapFile::getRowBW(int row, bool closefile) 00228 { 00229 open(); 00230 int *colors = new int[getWidth()]; 00231 int index = getOffset() + m_rowsize*row; 00232 fseek(m_pFile, index, SEEK_SET); 00233 if(getColorDepth() == 24) 00234 { 00235 for(int i=0; i<getWidth(); i++) 00236 { 00237 char temp[3]; 00238 fread(temp,sizeof(char),3,m_pFile); 00239 int average = (temp[0]+temp[1]+temp[2])/3; 00240 colors[i] = average>128 ? 0xFFFFFF : 0x000000; 00241 } 00242 } 00243 else if(getColorDepth() == 1) 00244 { 00245 delete [] colors; 00246 colors = getRow(row, closefile); 00247 } 00248 if(closefile) 00249 { 00250 close(); 00251 } 00252 return colors; 00253 } 00254 00255 char *BitmapFile::getRowBitstream(int row, bool closefile) 00256 { 00257 open(); 00258 int bitsperrow = (getWidth()+7)/8; 00259 char *data = new char[bitsperrow]; 00260 for(int i = 0; i<bitsperrow; i++) 00261 { 00262 data[i] = 0; 00263 } 00264 int index = getOffset() + m_rowsize*row; 00265 fseek(m_pFile, index, SEEK_SET); 00266 00267 if(getColorDepth() == 24) 00268 { 00269 for(int i=0; i<getWidth(); i++) 00270 { 00271 char temp[3]; 00272 fread(temp,sizeof(char),3,m_pFile); 00273 int average = (temp[0]+temp[1]+temp[2])/3; 00274 int val = average<128?0:1; 00275 data[i/8] |= (val*0x80) >> (i%8); 00276 } 00277 } 00278 else if(getColorDepth() == 1) 00279 { 00280 fread(data,sizeof(char),bitsperrow,m_pFile); 00281 } 00282 00283 if(closefile) 00284 { 00285 close(); 00286 } 00287 return data; 00288 }
Generated on Wed Jul 20 2022 11:12:02 by
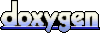