Updated many functions and bugs from the previous version. This program can emulate the keyboard and mouse on another computer using serial input [PC1-USB-MBED-USB-PC2] Control using serial input to keyboard/mouse output like a regular keyboard and mouse.
Fork of KeyboardMouseSerialV2 by
main.cpp
00001 #include "mbed.h" 00002 #include "USBMouseKeyboard.h" 00003 00004 USBMouseKeyboard key_mouse; 00005 Serial pc(USBTX, USBRX); 00006 00007 #define NFIELDS (6) 00008 char* pFields[NFIELDS]; 00009 00010 void ParseCommands(char* Buffer, char** pFields, uint32_t numFields, char* delimiter) { 00011 char* pString = Buffer; 00012 char* pField; 00013 00014 for (uint32_t i=0; i<numFields; i++) { 00015 pField = strtok(pString, delimiter); 00016 if (pField != NULL) { 00017 pFields[i] = pField; 00018 } else { 00019 pFields[i] = ""; 00020 } 00021 pString = NULL; //parse next 00022 } 00023 //Connection to device atempted 00024 if (strcmp("DRCmbed", pFields[0]) == 0) { 00025 pc.printf("mbeddevice\r\n");//Validate correct port query (For Ext Application Port Chcking) 00026 } 00027 //////////////////////////////// 00028 00029 if (strcmp("Vector", pFields[0]) == 0) { 00030 key_mouse.move(atoi(pFields[1]),atoi(pFields[2])); 00031 pc.printf("Vector Issued\r\n"); 00032 } 00033 00034 if (strcmp("Click", pFields[0]) == 0) { 00035 if (strcmp("LPress", pFields[1]) == 0) { 00036 key_mouse.press(MOUSE_LEFT); 00037 } 00038 if (strcmp("LRelease", pFields[1]) == 0) { 00039 key_mouse.release(MOUSE_LEFT); 00040 } 00041 if (strcmp("LClick", pFields[1]) == 0) { 00042 key_mouse.click(MOUSE_LEFT); 00043 } 00044 if (strcmp("LDClick", pFields[1]) == 0) { 00045 key_mouse.doubleClick(); 00046 } 00047 if (strcmp("RPress", pFields[1]) == 0) { 00048 key_mouse.press(MOUSE_RIGHT); 00049 } 00050 if (strcmp("RRelease", pFields[1]) == 0) { 00051 key_mouse.release(MOUSE_RIGHT); 00052 } 00053 if (strcmp("RClick", pFields[1]) == 0) { 00054 key_mouse.click(MOUSE_RIGHT); 00055 } 00056 pc.printf("Click Issued\r\n"); 00057 } 00058 00059 if (strcmp("Type", pFields[0]) == 0) { 00060 key_mouse.printf(pFields[1]); 00061 pc.printf("Type Issued\r\n"); 00062 } 00063 00064 if (strcmp("Keyboard", pFields[0]) == 0) { 00065 if (strcmp("Return", pFields[1]) == 0) { 00066 key_mouse.printf("\n"); 00067 } 00068 if (strcmp("Backspace", pFields[1]) == 0) { 00069 key_mouse.printf("\b"); 00070 } 00071 if (strcmp("Tab", pFields[1]) == 0) { 00072 key_mouse.printf("\t"); 00073 } 00074 if (strcmp("Capslock", pFields[1]) == 0) { 00075 key_mouse.keyCode(KEY_CAPS_LOCK); 00076 } 00077 if (strcmp("FKey1", pFields[1]) == 0) { 00078 key_mouse.keyCode(KEY_F1); 00079 } 00080 if (strcmp("FKey2", pFields[1]) == 0) { 00081 key_mouse.keyCode(KEY_F2); 00082 } 00083 if (strcmp("FKey3", pFields[1]) == 0) { 00084 key_mouse.keyCode(KEY_F3); 00085 } 00086 if (strcmp("FKey4", pFields[1]) == 0) { 00087 key_mouse.keyCode(KEY_F4); 00088 } 00089 if (strcmp("FKey5", pFields[1]) == 0) { 00090 key_mouse.keyCode(KEY_F5); 00091 } 00092 if (strcmp("FKey6", pFields[1]) == 0) { 00093 key_mouse.keyCode(KEY_F6); 00094 } 00095 if (strcmp("FKey7", pFields[1]) == 0) { 00096 key_mouse.keyCode(KEY_F7); 00097 } 00098 if (strcmp("FKey8", pFields[1]) == 0) { 00099 key_mouse.keyCode(KEY_F8); 00100 } 00101 if (strcmp("FKey9", pFields[1]) == 0) { 00102 key_mouse.keyCode(KEY_F9); 00103 } 00104 if (strcmp("FKey10", pFields[1]) == 0) { 00105 key_mouse.keyCode(KEY_F10); 00106 } 00107 if (strcmp("FKey11", pFields[1]) == 0) { 00108 key_mouse.keyCode(KEY_F11); 00109 } 00110 if (strcmp("FKey12", pFields[1]) == 0) { 00111 key_mouse.keyCode(KEY_F12); 00112 } 00113 pc.printf("Keyboard Issued\r\n"); 00114 } 00115 00116 if (strcmp("Volume", pFields[0]) == 0) { 00117 if (strcmp("Up", pFields[1]) == 0) { 00118 key_mouse.mediaControl(KEY_VOLUME_UP); 00119 } 00120 if (strcmp("Down", pFields[1]) == 0) { 00121 key_mouse.mediaControl(KEY_VOLUME_DOWN); 00122 } 00123 pc.printf("Volume Issued\r\n"); 00124 } 00125 00126 if (strcmp("Scroll", pFields[0]) == 0) { 00127 key_mouse.scroll(atoi(pFields[1])); 00128 pc.printf("Scroll Issued\r\n"); 00129 } 00130 } 00131 00132 int main(int argc, char* argv[]) { 00133 pc.baud(9600); 00134 char buf[256]; 00135 00136 //List of avalable commands 00137 //DRCmbed <-- Sends an auto response back to the computer host computer (Ext app com port validation event) 00138 //Type,Hello World <--Needs fix for spaces but can handle text the length how big the buffer is -1 = 255 bufferable characters 00139 //Vector,1000,6000 <--Vectors can be anything you specify 00140 //Click,LPress <--Holds the left mouse click down 00141 //Click,LClick <--Clicks the left mouse button 00142 //Click,LDClick <--Makes a left double click 00143 //Click,LRelease <--Releases the left mouse button 00144 //Click,RPress <--Holds the right mouse click down 00145 //Click,RRelease <--Releases the left mouse button 00146 //Click,RClick <--Clicks the right mouse button 00147 //Click,RDClick <--Makes a right double click 00148 //Scroll,10 <--Value can be anything you specify 00149 //Scroll,-10 <--Value can be anything you specify 00150 //Volume,Up <--Turns the system volume up 1 notch 00151 //Volume,Down <--Turns the sstem volume down 1 notch 00152 //Keyboard,Tab <--Emulates the system tab key 00153 //Keyboard,Capslock <--Emulates the system capslock key 00154 //Keyboard,Backspace <--Emulates the system backspace key 00155 //Keyboard,Return <--Emulates the system enter key 00156 //Keyboard,FKey1 <--Emulates the system F keys 00157 //Keyboard,FKey2 00158 //Keyboard,FKey3 00159 //Keyboard,FKey4 00160 //Keyboard,FKey5 00161 //Keyboard,FKey6 00162 //Keyboard,FKey7 00163 //Keyboard,FKey8 00164 //Keyboard,FKey9 00165 //Keyboard,FKey10 00166 //Keyboard,FKey11 00167 //Keyboard,FKey12 00168 while (1) { 00169 pc.scanf("%s", buf); 00170 ParseCommands(buf, pFields, NFIELDS, ","); 00171 } 00172 }
Generated on Sun Jul 24 2022 17:11:04 by
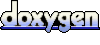