
EasyCAT test with SM synchronization - EtherCAT slave example
Dependencies: mbed EasyCAT_lib
main.cpp
00001 //******************************************************************************************** 00002 // * 00003 // AB&T Tecnologie Informatiche - Ivrea Italy * 00004 // http://www.bausano.net * 00005 // https://www.ethercat.org/en/products/791FFAA126AD43859920EA64384AD4FD.htm * 00006 // * 00007 //******************************************************************************************** 00008 // * 00009 // This software is distributed as an example, in the hope that it could be useful, * 00010 // WITHOUT ANY WARRANTY, even the implied warranty of FITNESS FOR A PARTICULAR PURPOSE * 00011 // * 00012 //******************************************************************************************** 00013 00014 00015 // revision 2 - moved "DigitalOut Led(LED1)" 00016 00017 00018 //- EasyCAT shield application basic example 170912 00019 //- using SM syncronization for mbed boards 00020 // 00021 //- Derived from the example project TestEasyCAT_SM_sync.ino for the AB&T EasyCAT Arduino shield 00022 // 00023 //- In this example we poll the interrupt pin coming from the LAN9252, so not a real interrupt, 00024 //- to synchronize the EasyCAT and the Master activities 00025 00026 00027 //- Tested with the STM32 NUCLEO-F767ZI board 00028 00029 00030 00031 #include "mbed.h" 00032 #include "EasyCAT.h" // EasyCAT library to interface the LAN9252 00033 00034 00035 void Application (void); 00036 void SafeOut(void); 00037 00038 00039 EasyCAT EASYCAT(SM_SYNC); // EasyCAT instantiation 00040 // In order to use the SM Synchronization we have to set 00041 // the "SM_SYNC" parameter in the constructor 00042 00043 // The constructor allow us to choose the pin used for the EasyCAT SPI chip select 00044 // Without any parameter pin 9 will be used 00045 00046 // for EasyCAT board REV_A we can choose between: 00047 // D8, D9, D10 00048 // 00049 // for EasyCAT board REV_B we can choose between: 00050 // D8, D9, D10, A5, D6, D7 00051 00052 // example: 00053 //EasyCAT EASYCAT(D8, SM_SYNC); // pin D8 will be used as SPI chip select 00054 00055 00056 // The chip select chosen by the firmware must match the setting on the board 00057 00058 // On board REV_A the chip select is set soldering 00059 // a 0 ohm resistor in the appropriate position 00060 00061 // On board REV_B the chip select is set 00062 // througt a bank of jumpers 00063 00064 00065 00066 //---- pins declaration ------------------------------------------------------------------------------ 00067 00068 00069 00070 AnalogIn Ana0(A0); // analog input 0 00071 AnalogIn Ana1(A1); // analog input 1 00072 00073 00074 DigitalOut Out_0(A2); // four bits output 00075 DigitalOut Out_1(A3); // 00076 DigitalOut Out_2(A4); // 00077 DigitalOut Out_3(A5); // 00078 00079 DigitalIn In_0(D3); // four bits input 00080 DigitalIn In_1(D5); // 00081 DigitalIn In_2(D6); // 00082 DigitalIn In_3(D7); // 00083 00084 00085 00086 00087 DigitalIn InterruptPin(D2); // This pin receive the SM interrupt 00088 // generated by the LAN9252 00089 // We poll it, so it is not used as an interrupt 00090 00091 00092 00093 //---- global variables --------------------------------------------------------------------------- 00094 00095 00096 UWORD ContaUp; // used for sawthoot test generation 00097 UWORD ContaDown; // 00098 00099 unsigned long Millis = 0; 00100 unsigned long PreviousSaw = 0; 00101 unsigned long PreviousCycle = 0; 00102 unsigned long PreviousMillis = 0; 00103 00104 00105 //---- declarations for Arduino "millis()" emulation ----------------------- 00106 00107 static Ticker uS_Tick; 00108 static volatile uint32_t MillisVal = 0; 00109 00110 void InitMillis(void); 00111 void mS_Tick(void); 00112 00113 inline static uint32_t millis (void) 00114 { 00115 return MillisVal; 00116 }; 00117 00118 00119 00120 //--------------------------------------------------------------------------------------------- 00121 00122 int main(void) 00123 { 00124 00125 printf ("\nEasyCAT - Generic EtherCAT slave\n"); // print the banner 00126 00127 InitMillis(); // init Arduino "millis()" emulation 00128 00129 ContaDown.Word = 0x0000; 00130 ContaUp.Word = 0x0000; 00131 //---- initialize the EasyCAT board ----- 00132 00133 if (EASYCAT.Init() == true) // initialization 00134 { // succesfully completed 00135 printf ("initialized\n"); // 00136 } 00137 00138 else // initialization failed 00139 { // the EasyCAT board was not recognized 00140 printf ("initialization failed\n"); // 00141 // The most common reason is that the SPI 00142 // chip select choosen on the board doesn't 00143 // match the one choosen by the firmware 00144 00145 DigitalOut Led(LED1); // 00146 00147 while (1) // stay in loop for ever 00148 { // with the led blinking 00149 Led = 1; // 00150 wait_ms(125); // 00151 Led = 0; // 00152 wait_ms(125); // 00153 } // 00154 } 00155 00156 00157 while (1) 00158 { 00159 00160 #define WatchDogTime 100 00161 00162 00163 Millis = millis(); // if there is no INT signal from the LAN9252 for more 00164 if (Millis - PreviousMillis >= WatchDogTime) // than 100mS we put the output in a safe state 00165 { // 00166 PreviousMillis = Millis; // 00167 // 00168 SafeOut(); // 00169 } // 00170 00171 00172 if (InterruptPin == 0) // If the INT signal is detected LOW 00173 { // 00174 00175 EASYCAT.MainTask(); // We execute the EtherCAT task 00176 // 00177 Application(); // and the user application 00178 00179 PreviousMillis = millis(); // refresh the watchdog timer 00180 } 00181 } 00182 } 00183 00184 00185 00186 00187 00188 00189 00190 00191 //---- user application ------------------------------------------------------------------------------ 00192 00193 void Application (void) 00194 00195 { 00196 float Analog; 00197 // --- analog inputs management --- 00198 // 00199 Analog = Ana0.read(); // read analog input 0 00200 Analog = Analog * 255; // normalize it on 8 bits 00201 EASYCAT.BufferIn.Byte[0] = (uint8_t)Analog; // and put the result into 00202 // input Byte 0 00203 00204 Analog = Ana1.read(); // read analog input 1 00205 Analog = Analog * 255; // normalize it on 8 bits 00206 EASYCAT.BufferIn.Byte[1] = (uint8_t)Analog; // and put the result into 00207 // input Byte 1 00208 00209 00210 // --- four output bits management ---- 00211 // 00212 if (EASYCAT.BufferOut.Byte[0] & 0b00000001) // the four output bits are mapped to the 00213 Out_0 = 1; // lower nibble of output Byte 0 00214 else // 00215 Out_0 = 0; // 00216 // 00217 if (EASYCAT.BufferOut.Byte[0] & (1<<0)) // 00218 Out_0 = 1; // 00219 else // 00220 Out_0 = 0; // 00221 // 00222 if (EASYCAT.BufferOut.Byte[0] & (1<<1)) // 00223 Out_1 = 1; // 00224 else // 00225 Out_1 = 0; // 00226 // 00227 if (EASYCAT.BufferOut.Byte[0] & (1<<2)) // 00228 Out_2 = 1; // 00229 else // 00230 Out_2 = 0; // 00231 // 00232 if (EASYCAT.BufferOut.Byte[0] & (1<<3)) // 00233 Out_3 = 1; // 00234 else // 00235 Out_3 = 0; // 00236 00237 //--- four input bits management --- 00238 // 00239 EASYCAT.BufferIn.Byte[6] = 0x00; // the four input pins are mapped to the 00240 if (!In_0) // lower nibble of input Byte 6 00241 EASYCAT.BufferIn.Byte[6] |= 0b00000001; // 00242 if (!In_1) // 00243 EASYCAT.BufferIn.Byte[6] |= 0b00000010; // 00244 if (!In_2) // 00245 EASYCAT.BufferIn.Byte[6] |= 0b00000100; // 00246 if (!In_3) // 00247 EASYCAT.BufferIn.Byte[6] |= 0b00001000; // 00248 00249 00250 // --- test sawtooth generation --- 00251 // 00252 Millis = millis(); // each 100 mS 00253 00254 if (Millis - PreviousSaw >= 100) // 00255 { // 00256 PreviousSaw = Millis; // 00257 // 00258 ContaUp.Word++; // we increment the variable ContaUp 00259 ContaDown.Word--; // and decrement ContaDown 00260 } // 00261 00262 // we use these variables to create sawtooth, 00263 // with different slopes and periods, for 00264 // test pourpose, in input Bytes 2,3,4,5,30,31 00265 00266 EASYCAT.BufferIn.Byte[2] = ContaUp.Byte[0]; // slow rising slope 00267 EASYCAT.BufferIn.Byte[3] = ContaUp.Byte[1]; // extremly slow rising slope 00268 00269 EASYCAT.BufferIn.Byte[4] = ContaDown.Byte[0]; // slow falling slope 00270 EASYCAT.BufferIn.Byte[5] = ContaDown.Byte[1]; // extremly slow falling slope 00271 00272 00273 EASYCAT.BufferIn.Byte[30] = ContaUp.Byte[0] << 2; // medium speed rising slope 00274 EASYCAT.BufferIn.Byte[31] = ContaDown.Byte[0] << 2; // medium speed falling slope 00275 } 00276 00277 00278 //------------------------------------------------------------------------------------------------ 00279 00280 void SafeOut(void) 00281 00282 { 00283 Out_0 = 0; 00284 Out_1 = 0; 00285 Out_2 = 0; 00286 Out_3 = 0; 00287 } 00288 00289 00290 //--- functions for Arduino "millis()" emulation ------------------------------------- 00291 00292 00293 void InitMillis(void) 00294 { 00295 uS_Tick.attach (&mS_Tick, 0.001); 00296 } 00297 00298 void mS_Tick(void) 00299 { 00300 MillisVal++; 00301 } 00302 00303
Generated on Thu Jul 14 2022 07:29:47 by
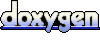