corrected version (with typedef struct IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE_DATA* IOTHUB_CLIENT_LL_UPLOADTOBLOB_HANDLE;) included in the sources
Dependents: STM32F746_iothub_client_sample_mqtt
Fork of iothub_client by
iothub_message.h
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 /** @file iothub_message.h 00005 * @brief The @c IoTHub_Message component encapsulates one message that 00006 * can be transferred by an IoT hub client. 00007 */ 00008 00009 #ifndef IOTHUB_MESSAGE_H 00010 #define IOTHUB_MESSAGE_H 00011 00012 #include "azure_c_shared_utility/macro_utils.h" 00013 #include "azure_c_shared_utility/map.h" 00014 00015 #ifdef __cplusplus 00016 #include <cstddef> 00017 #include <ctime> 00018 #include <cstdint> 00019 extern "C" 00020 { 00021 #else 00022 #include <stddef.h> 00023 #include <time.h> 00024 #include <stdint.h> 00025 #endif 00026 00027 #define IOTHUB_MESSAGE_RESULT_VALUES \ 00028 IOTHUB_MESSAGE_OK, \ 00029 IOTHUB_MESSAGE_INVALID_ARG, \ 00030 IOTHUB_MESSAGE_INVALID_TYPE, \ 00031 IOTHUB_MESSAGE_ERROR \ 00032 00033 /** @brief Enumeration specifying the status of calls to various 00034 * APIs in this module. 00035 */ 00036 DEFINE_ENUM(IOTHUB_MESSAGE_RESULT, IOTHUB_MESSAGE_RESULT_VALUES); 00037 00038 #define IOTHUBMESSAGE_CONTENT_TYPE_VALUES \ 00039 IOTHUBMESSAGE_BYTEARRAY, \ 00040 IOTHUBMESSAGE_STRING, \ 00041 IOTHUBMESSAGE_UNKNOWN \ 00042 00043 /** @brief Enumeration specifying the content type of the a given 00044 * message. 00045 */ 00046 DEFINE_ENUM(IOTHUBMESSAGE_CONTENT_TYPE, IOTHUBMESSAGE_CONTENT_TYPE_VALUES); 00047 00048 typedef void* IOTHUB_MESSAGE_HANDLE; 00049 00050 /** 00051 * @brief Creates a new IoT hub message from a byte array. The type of the 00052 * message will be set to @c IOTHUBMESSAGE_BYTEARRAY. 00053 * 00054 * @param byteArray The byte array from which the message is to be created. 00055 * @param size The size of the byte array. 00056 * 00057 * @return A valid @c IOTHUB_MESSAGE_HANDLE if the message was successfully 00058 * created or @c NULL in case an error occurs. 00059 */ 00060 extern IOTHUB_MESSAGE_HANDLE IoTHubMessage_CreateFromByteArray(const unsigned char* byteArray, size_t size); 00061 00062 /** 00063 * @brief Creates a new IoT hub message from a null terminated string. The 00064 * type of the message will be set to @c IOTHUBMESSAGE_STRING. 00065 * 00066 * @param source The null terminated string from which the message is to be 00067 * created. 00068 * 00069 * @return A valid @c IOTHUB_MESSAGE_HANDLE if the message was successfully 00070 * created or @c NULL in case an error occurs. 00071 */ 00072 extern IOTHUB_MESSAGE_HANDLE IoTHubMessage_CreateFromString(const char* source); 00073 00074 /** 00075 * @brief Creates a new IoT hub message with the content identical to that 00076 * of the @p iotHubMessageHandle parameter. 00077 * 00078 * @param iotHubMessageHandle Handle to the message that is to be cloned. 00079 * 00080 * @return A valid @c IOTHUB_MESSAGE_HANDLE if the message was successfully 00081 * cloned or @c NULL in case an error occurs. 00082 */ 00083 extern IOTHUB_MESSAGE_HANDLE IoTHubMessage_Clone(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00084 00085 /** 00086 * @brief Fetches a pointer and size for the data associated with the IoT 00087 * hub message handle. If the content type of the message is not 00088 * @c IOTHUBMESSAGE_BYTEARRAY then the function returns 00089 * @c IOTHUB_MESSAGE_INVALID_ARG. 00090 * 00091 * @param iotHubMessageHandle Handle to the message. 00092 * @param buffer Pointer to the memory location where the 00093 * pointer to the buffer will be written. 00094 * @param size The size of the buffer will be written to 00095 * this address. 00096 * 00097 * @return Returns IOTHUB_MESSAGE_OK if the byte array was fetched successfully 00098 * or an error code otherwise. 00099 */ 00100 extern IOTHUB_MESSAGE_RESULT IoTHubMessage_GetByteArray(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const unsigned char** buffer, size_t* size); 00101 00102 /** 00103 * @brief Returns the null terminated string stored in the message. 00104 * If the content type of the message is not @c IOTHUBMESSAGE_STRING 00105 * then the function returns @c NULL. 00106 * 00107 * @param iotHubMessageHandle Handle to the message. 00108 * 00109 * @return @c NULL if an error occurs or a pointer to the stored null 00110 * terminated string otherwise. 00111 */ 00112 extern const char* IoTHubMessage_GetString(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00113 00114 /** 00115 * @brief Returns the content type of the message given by parameter 00116 * @c iotHubMessageHandle. 00117 * 00118 * @param iotHubMessageHandle Handle to the message. 00119 * 00120 * @return An @c IOTHUBMESSAGE_CONTENT_TYPE value. 00121 */ 00122 extern IOTHUBMESSAGE_CONTENT_TYPE IoTHubMessage_GetContentType(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00123 00124 /** 00125 * @brief Gets a handle to the message's properties map. 00126 * 00127 * @param iotHubMessageHandle Handle to the message. 00128 * 00129 * @return A @c MAP_HANDLE pointing to the properties map for this message. 00130 */ 00131 extern MAP_HANDLE IoTHubMessage_Properties(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00132 00133 /** 00134 * @brief Gets the MessageId from the IOTHUB_MESSAGE_HANDLE. 00135 * 00136 * @param iotHubMessageHandle Handle to the message. 00137 * 00138 * @return A const char* pointing to the Message Id. 00139 */ 00140 extern const char* IoTHubMessage_GetMessageId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00141 00142 /** 00143 * @brief Sets the MessageId for the IOTHUB_MESSAGE_HANDLE. 00144 * 00145 * @param iotHubMessageHandle Handle to the message. 00146 * @param messageId Pointer to the memory location of the messageId 00147 * 00148 * @return Returns IOTHUB_MESSAGE_OK if the messageId was set successfully 00149 * or an error code otherwise. 00150 */ 00151 extern IOTHUB_MESSAGE_RESULT IoTHubMessage_SetMessageId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const char* messageId); 00152 00153 /** 00154 * @brief Gets the CorrelationId from the IOTHUB_MESSAGE_HANDLE. 00155 * 00156 * @param iotHubMessageHandle Handle to the message. 00157 * 00158 * @return A const char* pointing to the Correlation Id. 00159 */ 00160 extern const char* IoTHubMessage_GetCorrelationId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00161 00162 /** 00163 * @brief Sets the CorrelationId for the IOTHUB_MESSAGE_HANDLE. 00164 * 00165 * @param iotHubMessageHandle Handle to the message. 00166 * @param correlationId Pointer to the memory location of the messageId 00167 * 00168 * @return Returns IOTHUB_MESSAGE_OK if the messageId was set successfully 00169 * or an error code otherwise. 00170 */ 00171 extern IOTHUB_MESSAGE_RESULT IoTHubMessage_SetCorrelationId(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle, const char* correlationId); 00172 00173 /** 00174 * @brief Frees all resources associated with the given message handle. 00175 * 00176 * @param iotHubMessageHandle Handle to the message. 00177 */ 00178 extern void IoTHubMessage_Destroy(IOTHUB_MESSAGE_HANDLE iotHubMessageHandle); 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* IOTHUB_MESSAGE_H */
Generated on Tue Jul 12 2022 19:44:54 by
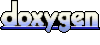