Ethernet for Nucleo and Disco board STM32F746 works with gcc and arm. IAC is untested
Dependents: STM32F746_iothub_client_sample_mqtt DISCO-F746NG_Ethernet Nucleo_F746ZG_Ethernet thethingsiO-DISCO_F746NG-mqtt ... more
netbuf.c
00001 /** 00002 * @file 00003 * Network buffer management 00004 * 00005 */ 00006 00007 /* 00008 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00009 * All rights reserved. 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. The name of the author may not be used to endorse or promote products 00020 * derived from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00023 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00024 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00025 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00026 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00027 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00028 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00029 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00030 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00031 * OF SUCH DAMAGE. 00032 * 00033 * This file is part of the lwIP TCP/IP stack. 00034 * 00035 * Author: Adam Dunkels <adam@sics.se> 00036 * 00037 */ 00038 00039 #include "lwip/opt.h" 00040 00041 #if LWIP_NETCONN /* don't build if not configured for use in lwipopts.h */ 00042 00043 #include "lwip/netbuf.h" 00044 #include "lwip/memp.h" 00045 00046 #include <string.h> 00047 00048 /** 00049 * Create (allocate) and initialize a new netbuf. 00050 * The netbuf doesn't yet contain a packet buffer! 00051 * 00052 * @return a pointer to a new netbuf 00053 * NULL on lack of memory 00054 */ 00055 struct 00056 netbuf *netbuf_new(void) 00057 { 00058 struct netbuf *buf; 00059 00060 buf = (struct netbuf *)memp_malloc(MEMP_NETBUF); 00061 if (buf != NULL) { 00062 buf->p = NULL; 00063 buf->ptr = NULL; 00064 ip_addr_set_any(&buf->addr); 00065 buf->port = 0; 00066 #if LWIP_NETBUF_RECVINFO || LWIP_CHECKSUM_ON_COPY 00067 #if LWIP_CHECKSUM_ON_COPY 00068 buf->flags = 0; 00069 #endif /* LWIP_CHECKSUM_ON_COPY */ 00070 buf->toport_chksum = 0; 00071 #if LWIP_NETBUF_RECVINFO 00072 ip_addr_set_any(&buf->toaddr); 00073 #endif /* LWIP_NETBUF_RECVINFO */ 00074 #endif /* LWIP_NETBUF_RECVINFO || LWIP_CHECKSUM_ON_COPY */ 00075 return buf; 00076 } else { 00077 return NULL; 00078 } 00079 } 00080 00081 /** 00082 * Deallocate a netbuf allocated by netbuf_new(). 00083 * 00084 * @param buf pointer to a netbuf allocated by netbuf_new() 00085 */ 00086 void 00087 netbuf_delete(struct netbuf *buf) 00088 { 00089 if (buf != NULL) { 00090 if (buf->p != NULL) { 00091 pbuf_free(buf->p); 00092 buf->p = buf->ptr = NULL; 00093 } 00094 memp_free(MEMP_NETBUF, buf); 00095 } 00096 } 00097 00098 /** 00099 * Allocate memory for a packet buffer for a given netbuf. 00100 * 00101 * @param buf the netbuf for which to allocate a packet buffer 00102 * @param size the size of the packet buffer to allocate 00103 * @return pointer to the allocated memory 00104 * NULL if no memory could be allocated 00105 */ 00106 void * 00107 netbuf_alloc(struct netbuf *buf, u16_t size) 00108 { 00109 LWIP_ERROR("netbuf_alloc: invalid buf", (buf != NULL), return NULL;); 00110 00111 /* Deallocate any previously allocated memory. */ 00112 if (buf->p != NULL) { 00113 pbuf_free(buf->p); 00114 } 00115 buf->p = pbuf_alloc(PBUF_TRANSPORT, size, PBUF_RAM); 00116 if (buf->p == NULL) { 00117 return NULL; 00118 } 00119 LWIP_ASSERT("check that first pbuf can hold size", 00120 (buf->p->len >= size)); 00121 buf->ptr = buf->p; 00122 return buf->p->payload; 00123 } 00124 00125 /** 00126 * Free the packet buffer included in a netbuf 00127 * 00128 * @param buf pointer to the netbuf which contains the packet buffer to free 00129 */ 00130 void 00131 netbuf_free(struct netbuf *buf) 00132 { 00133 LWIP_ERROR("netbuf_free: invalid buf", (buf != NULL), return;); 00134 if (buf->p != NULL) { 00135 pbuf_free(buf->p); 00136 } 00137 buf->p = buf->ptr = NULL; 00138 } 00139 00140 /** 00141 * Let a netbuf reference existing (non-volatile) data. 00142 * 00143 * @param buf netbuf which should reference the data 00144 * @param dataptr pointer to the data to reference 00145 * @param size size of the data 00146 * @return ERR_OK if data is referenced 00147 * ERR_MEM if data couldn't be referenced due to lack of memory 00148 */ 00149 err_t 00150 netbuf_ref(struct netbuf *buf, const void *dataptr, u16_t size) 00151 { 00152 LWIP_ERROR("netbuf_ref: invalid buf", (buf != NULL), return ERR_ARG;); 00153 if (buf->p != NULL) { 00154 pbuf_free(buf->p); 00155 } 00156 buf->p = pbuf_alloc(PBUF_TRANSPORT, 0, PBUF_REF); 00157 if (buf->p == NULL) { 00158 buf->ptr = NULL; 00159 return ERR_MEM; 00160 } 00161 buf->p->payload = (void*)dataptr; 00162 buf->p->len = buf->p->tot_len = size; 00163 buf->ptr = buf->p; 00164 return ERR_OK; 00165 } 00166 00167 /** 00168 * Chain one netbuf to another (@see pbuf_chain) 00169 * 00170 * @param head the first netbuf 00171 * @param tail netbuf to chain after head, freed by this function, may not be reference after returning 00172 */ 00173 void 00174 netbuf_chain(struct netbuf *head, struct netbuf *tail) 00175 { 00176 LWIP_ERROR("netbuf_ref: invalid head", (head != NULL), return;); 00177 LWIP_ERROR("netbuf_chain: invalid tail", (tail != NULL), return;); 00178 pbuf_cat(head->p, tail->p); 00179 head->ptr = head->p; 00180 memp_free(MEMP_NETBUF, tail); 00181 } 00182 00183 /** 00184 * Get the data pointer and length of the data inside a netbuf. 00185 * 00186 * @param buf netbuf to get the data from 00187 * @param dataptr pointer to a void pointer where to store the data pointer 00188 * @param len pointer to an u16_t where the length of the data is stored 00189 * @return ERR_OK if the information was retreived, 00190 * ERR_BUF on error. 00191 */ 00192 err_t 00193 netbuf_data(struct netbuf *buf, void **dataptr, u16_t *len) 00194 { 00195 LWIP_ERROR("netbuf_data: invalid buf", (buf != NULL), return ERR_ARG;); 00196 LWIP_ERROR("netbuf_data: invalid dataptr", (dataptr != NULL), return ERR_ARG;); 00197 LWIP_ERROR("netbuf_data: invalid len", (len != NULL), return ERR_ARG;); 00198 00199 if (buf->ptr == NULL) { 00200 return ERR_BUF; 00201 } 00202 *dataptr = buf->ptr->payload; 00203 *len = buf->ptr->len; 00204 return ERR_OK; 00205 } 00206 00207 /** 00208 * Move the current data pointer of a packet buffer contained in a netbuf 00209 * to the next part. 00210 * The packet buffer itself is not modified. 00211 * 00212 * @param buf the netbuf to modify 00213 * @return -1 if there is no next part 00214 * 1 if moved to the next part but now there is no next part 00215 * 0 if moved to the next part and there are still more parts 00216 */ 00217 s8_t 00218 netbuf_next(struct netbuf *buf) 00219 { 00220 LWIP_ERROR("netbuf_free: invalid buf", (buf != NULL), return -1;); 00221 if (buf->ptr->next == NULL) { 00222 return -1; 00223 } 00224 buf->ptr = buf->ptr->next; 00225 if (buf->ptr->next == NULL) { 00226 return 1; 00227 } 00228 return 0; 00229 } 00230 00231 /** 00232 * Move the current data pointer of a packet buffer contained in a netbuf 00233 * to the beginning of the packet. 00234 * The packet buffer itself is not modified. 00235 * 00236 * @param buf the netbuf to modify 00237 */ 00238 void 00239 netbuf_first(struct netbuf *buf) 00240 { 00241 LWIP_ERROR("netbuf_free: invalid buf", (buf != NULL), return;); 00242 buf->ptr = buf->p; 00243 } 00244 00245 #endif /* LWIP_NETCONN */
Generated on Tue Jul 12 2022 18:14:54 by
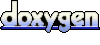