Ethernet for Nucleo and Disco board STM32F746 works with gcc and arm. IAC is untested
Dependents: STM32F746_iothub_client_sample_mqtt DISCO-F746NG_Ethernet Nucleo_F746ZG_Ethernet thethingsiO-DISCO_F746NG-mqtt ... more
api_msg.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_API_MSG_H__ 00033 #define __LWIP_API_MSG_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #if LWIP_NETCONN /* don't build if not configured for use in lwipopts.h */ 00038 00039 #include <stddef.h> /* for size_t */ 00040 00041 #include "lwip/ip_addr.h" 00042 #include "lwip/err.h" 00043 #include "lwip/sys.h" 00044 #include "lwip/igmp.h" 00045 #include "lwip/api.h" 00046 00047 #ifdef __cplusplus 00048 extern "C" { 00049 #endif 00050 00051 /* For the netconn API, these values are use as a bitmask! */ 00052 #define NETCONN_SHUT_RD 1 00053 #define NETCONN_SHUT_WR 2 00054 #define NETCONN_SHUT_RDWR (NETCONN_SHUT_RD | NETCONN_SHUT_WR) 00055 00056 /* IP addresses and port numbers are expected to be in 00057 * the same byte order as in the corresponding pcb. 00058 */ 00059 /** This struct includes everything that is necessary to execute a function 00060 for a netconn in another thread context (mainly used to process netconns 00061 in the tcpip_thread context to be thread safe). */ 00062 struct api_msg_msg { 00063 /** The netconn which to process - always needed: it includes the semaphore 00064 which is used to block the application thread until the function finished. */ 00065 struct netconn *conn; 00066 /** The return value of the function executed in tcpip_thread. */ 00067 err_t err; 00068 /** Depending on the executed function, one of these union members is used */ 00069 union { 00070 /** used for do_send */ 00071 struct netbuf *b; 00072 /** used for do_newconn */ 00073 struct { 00074 u8_t proto; 00075 } n; 00076 /** used for do_bind and do_connect */ 00077 struct { 00078 ip_addr_t *ipaddr; 00079 u16_t port; 00080 } bc; 00081 /** used for do_getaddr */ 00082 struct { 00083 ip_addr_t *ipaddr; 00084 u16_t *port; 00085 u8_t local; 00086 } ad; 00087 /** used for do_write */ 00088 struct { 00089 const void *dataptr; 00090 size_t len; 00091 u8_t apiflags; 00092 #if LWIP_SO_SNDTIMEO 00093 u32_t time_started; 00094 #endif /* LWIP_SO_SNDTIMEO */ 00095 } w; 00096 /** used for do_recv */ 00097 struct { 00098 u32_t len; 00099 } r; 00100 /** used for do_close (/shutdown) */ 00101 struct { 00102 u8_t shut; 00103 } sd; 00104 #if LWIP_IGMP 00105 /** used for do_join_leave_group */ 00106 struct { 00107 ip_addr_t *multiaddr; 00108 ip_addr_t *netif_addr; 00109 enum netconn_igmp join_or_leave; 00110 } jl; 00111 #endif /* LWIP_IGMP */ 00112 #if TCP_LISTEN_BACKLOG 00113 struct { 00114 u8_t backlog; 00115 } lb; 00116 #endif /* TCP_LISTEN_BACKLOG */ 00117 } msg; 00118 }; 00119 00120 /** This struct contains a function to execute in another thread context and 00121 a struct api_msg_msg that serves as an argument for this function. 00122 This is passed to tcpip_apimsg to execute functions in tcpip_thread context. */ 00123 struct api_msg { 00124 /** function to execute in tcpip_thread context */ 00125 void (* function)(struct api_msg_msg *msg); 00126 /** arguments for this function */ 00127 struct api_msg_msg msg; 00128 }; 00129 00130 #if LWIP_DNS 00131 /** As do_gethostbyname requires more arguments but doesn't require a netconn, 00132 it has its own struct (to avoid struct api_msg getting bigger than necessary). 00133 do_gethostbyname must be called using tcpip_callback instead of tcpip_apimsg 00134 (see netconn_gethostbyname). */ 00135 struct dns_api_msg { 00136 /** Hostname to query or dotted IP address string */ 00137 const char *name; 00138 /** Rhe resolved address is stored here */ 00139 ip_addr_t *addr; 00140 /** This semaphore is posted when the name is resolved, the application thread 00141 should wait on it. */ 00142 sys_sem_t *sem; 00143 /** Errors are given back here */ 00144 err_t *err; 00145 }; 00146 #endif /* LWIP_DNS */ 00147 00148 void do_newconn ( struct api_msg_msg *msg); 00149 void do_delconn ( struct api_msg_msg *msg); 00150 void do_bind ( struct api_msg_msg *msg); 00151 void do_connect ( struct api_msg_msg *msg); 00152 void do_disconnect ( struct api_msg_msg *msg); 00153 void do_listen ( struct api_msg_msg *msg); 00154 void do_send ( struct api_msg_msg *msg); 00155 void do_recv ( struct api_msg_msg *msg); 00156 void do_write ( struct api_msg_msg *msg); 00157 void do_getaddr ( struct api_msg_msg *msg); 00158 void do_close ( struct api_msg_msg *msg); 00159 void do_shutdown ( struct api_msg_msg *msg); 00160 #if LWIP_IGMP 00161 void do_join_leave_group( struct api_msg_msg *msg); 00162 #endif /* LWIP_IGMP */ 00163 00164 #if LWIP_DNS 00165 void do_gethostbyname(void *arg); 00166 #endif /* LWIP_DNS */ 00167 00168 struct netconn* netconn_alloc(enum netconn_type t, netconn_callback callback); 00169 void netconn_free(struct netconn *conn); 00170 00171 #ifdef __cplusplus 00172 } 00173 #endif 00174 00175 #endif /* LWIP_NETCONN */ 00176 00177 #endif /* __LWIP_API_MSG_H__ */
Generated on Tue Jul 12 2022 18:14:54 by
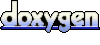