Ethernet for Nucleo and Disco board STM32F746 works with gcc and arm. IAC is untested
Dependents: STM32F746_iothub_client_sample_mqtt DISCO-F746NG_Ethernet Nucleo_F746ZG_Ethernet thethingsiO-DISCO_F746NG-mqtt ... more
TCPSocketServer.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketServer.h" 00019 00020 #include <cstring> 00021 00022 using std::memset; 00023 using std::memcpy; 00024 00025 TCPSocketServer::TCPSocketServer() { 00026 00027 } 00028 00029 int TCPSocketServer::bind(int port) { 00030 if (init_socket(SOCK_STREAM) < 0) 00031 return -1; 00032 00033 struct sockaddr_in localHost; 00034 memset(&localHost, 0, sizeof(localHost)); 00035 00036 localHost.sin_family = AF_INET; 00037 localHost.sin_port = htons(port); 00038 localHost.sin_addr.s_addr = INADDR_ANY; 00039 00040 if (lwip_bind(_sock_fd, (const struct sockaddr *) &localHost, sizeof(localHost)) < 0) { 00041 close(); 00042 return -1; 00043 } 00044 00045 return 0; 00046 } 00047 00048 int TCPSocketServer::listen(int max) { 00049 if (_sock_fd < 0) 00050 return -1; 00051 00052 if (lwip_listen(_sock_fd, max) < 0) { 00053 close(); 00054 return -1; 00055 } 00056 00057 return 0; 00058 } 00059 00060 int TCPSocketServer::accept(TCPSocketConnection& connection) { 00061 if (_sock_fd < 0) 00062 return -1; 00063 00064 if (!_blocking) { 00065 TimeInterval timeout(_timeout); 00066 if (wait_readable(timeout) != 0) 00067 return -1; 00068 } 00069 connection.reset_address(); 00070 socklen_t newSockRemoteHostLen = sizeof(connection._remoteHost); 00071 int fd = lwip_accept(_sock_fd, (struct sockaddr*) &connection._remoteHost, &newSockRemoteHostLen); 00072 if (fd < 0) 00073 return -1; //Accept failed 00074 connection._sock_fd = fd; 00075 connection._is_connected = true; 00076 connection.set_address(connection._remoteHost.sin_addr); 00077 return 0; 00078 }
Generated on Tue Jul 12 2022 18:14:55 by
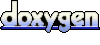