R1 code for micro:bit based train controller code, requires second micro:bit running rx code to operate - see https://meanderingpi.wordpress.com/ for more information
Fork of nrf51-sdk by
Peer Data Storage
[Peer_manager]
An internal module of Peer_manager. More...
Data Structures | |
struct | pds_evt_t |
Events that can come from the peer_data_storage module. More... | |
Typedefs | |
typedef void(* | pds_evt_handler_t )(pds_evt_t const *p_event) |
Event handler for events from the peer_data_storage module. | |
Enumerations | |
enum | pds_evt_id_t { PDS_EVT_STORED, PDS_EVT_CLEARED, PDS_EVT_PEER_ID_CLEAR, PDS_EVT_ERROR_STORE, PDS_EVT_ERROR_CLEAR, PDS_EVT_ERROR_PEER_ID_CLEAR, PDS_EVT_COMPRESSED } |
The types of events that can come from the peer_data_storage module. More... | |
Functions | |
ret_code_t | pds_register (pds_evt_handler_t evt_handler) |
Function for registering for events from the peer database. | |
ret_code_t | pds_init (pds_evt_handler_t evt_handler) |
Function for initializing Peer Data storage and registering a callback for its events. | |
ret_code_t | pds_peer_data_read_ptr_get (pm_peer_id_t peer_id, pm_peer_data_id_t data_id, pm_peer_data_flash_t *p_data, pm_store_token_t *p_token) |
Function for retrieving a direct pointer to peer data in persistent storage. | |
ret_code_t | pds_peer_data_lock (pm_store_token_t store_token) |
Function to lock the flash data (to defer compression from invalidating data) | |
ret_code_t | pds_peer_data_verify (pm_store_token_t store_token) |
Function to verify flash data integrity. | |
ret_code_t | pds_peer_data_read (pm_peer_id_t peer_id, pm_peer_data_id_t data_id, pm_peer_data_t *p_data, fds_length_t *p_len_words) |
Function for retrieving peer data from persistent storage by making a copy. | |
ret_code_t | pds_peer_data_write_prepare (pm_peer_data_const_t const *p_peer_data, pm_prepare_token_t *p_prepare_token) |
Function for preparing persistent storage for a write. | |
ret_code_t | pds_peer_data_write_prepare_cancel (pm_prepare_token_t prepare_token) |
Function for undoing a previous call to pds_peer_data_write_prepare. | |
ret_code_t | pds_peer_data_write_prepared (pm_peer_id_t peer_id, pm_peer_data_const_t const *p_peer_data, pm_prepare_token_t prepare_token, pm_store_token_t *p_store_token) |
Function for writing prepared (reserved) peer data to persistent storage. | |
ret_code_t | pds_peer_data_write (pm_peer_id_t peer_id, pm_peer_data_const_t const *p_peer_data, pm_store_token_t *p_store_token) |
Function for writing peer data to persistent storage. | |
ret_code_t | pds_peer_data_update (pm_peer_id_t peer_id, pm_peer_data_const_t const *peer_data, pm_store_token_t old_token, pm_store_token_t *p_store_token) |
Function for updating currently stored peer data to a new version. | |
ret_code_t | pds_peer_data_clear (pm_peer_id_t peer_id, pm_peer_data_id_t data_id) |
Function for clearing peer data from persistent storage. | |
pm_peer_id_t | pds_peer_id_allocate (void) |
Function for claiming an unused peer ID. | |
ret_code_t | pds_peer_id_free (pm_peer_id_t peer_id) |
Function for freeing a peer ID and clearing all data associated with it in persistent storage. | |
bool | pds_peer_id_is_allocated (pm_peer_id_t peer_id) |
Function for finding out whether a peer ID is in use. | |
pm_peer_id_t | pds_next_peer_id_get (pm_peer_id_t prev_peer_id) |
Function for getting the next peer ID in the sequence of all used peer IDs. | |
uint32_t | pds_n_peers (void) |
Function for querying the number of valid peer IDs available. |
Detailed Description
An internal module of Peer_manager.
This module provides a Peer Manager-specific API to the persistent storage.
Typedef Documentation
typedef void(* pds_evt_handler_t)(pds_evt_t const *p_event) |
Event handler for events from the peer_data_storage module.
- Parameters:
-
[in] event The event that has happened. [in] peer_id The id of the peer the event pertains to. [in] flags The data the event pertains to.
Definition at line 97 of file peer_data_storage.h.
Enumeration Type Documentation
enum pds_evt_id_t |
The types of events that can come from the peer_data_storage module.
- Enumerator:
Definition at line 68 of file peer_data_storage.h.
Function Documentation
ret_code_t pds_init | ( | pds_evt_handler_t | evt_handler ) |
Function for initializing Peer Data storage and registering a callback for its events.
- Parameters:
-
[in] evt_handler Event handler to register.
- Return values:
-
NRF_SUCCESS Registration successful. NRF_ERROR_NO_MEM No more event handlers can be registered. NRF_ERROR_NULL evt_handler was NULL. NRF_ERROR_INVALID_STATE FDS has not completed initialization.
uint32_t pds_n_peers | ( | void | ) |
Function for querying the number of valid peer IDs available.
I.E the number of peers in persistent storage.
- Returns:
- The number of valid peer IDs, or 0 if module is not initialized.
Definition at line 679 of file peer_data_storage.c.
pm_peer_id_t pds_next_peer_id_get | ( | pm_peer_id_t | prev_peer_id ) |
Function for getting the next peer ID in the sequence of all used peer IDs.
Can be used to loop through all used peer IDs.
- Note:
- PM_PEER_ID_INVALID is considered to be before the first and after the last ordinary peer ID.
- Parameters:
-
[in] prev_peer_id The previous peer ID.
- Returns:
- The next peer ID.
- The first ordinary peer ID if prev_peer_id was PM_PEER_ID_INVALID.
- Return values:
-
PM_PEER_ID_INVALID if prev_peer_id was the last ordinary peer ID or the module is not initialized.
Definition at line 667 of file peer_data_storage.c.
ret_code_t pds_peer_data_clear | ( | pm_peer_id_t | peer_id, |
pm_peer_data_id_t | data_id | ||
) |
Function for clearing peer data from persistent storage.
Clearing happens asynchronously. Expect a PDS_EVT_CLEARED or PDS_EVT_ERROR_CLEAR event.
- Parameters:
-
[in] peer_id The id of the peer the data pertains to. [in] data_id Which data to clear.
- Return values:
-
NRF_SUCCESS The clear was initiated successfully. NRF_ERROR_INVALID_PARAM Data ID or was invalid. NRF_ERROR_NOT_FOUND Nothing to clear for this peer ID. NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 602 of file peer_data_storage.c.
ret_code_t pds_peer_data_lock | ( | pm_store_token_t | store_token ) |
Function to lock the flash data (to defer compression from invalidating data)
- Parameters:
-
[in] store_token The token representing the item to lock
Definition at line 413 of file peer_data_storage.c.
ret_code_t pds_peer_data_read | ( | pm_peer_id_t | peer_id, |
pm_peer_data_id_t | data_id, | ||
pm_peer_data_t * | p_data, | ||
fds_length_t * | p_len_words | ||
) |
Function for retrieving peer data from persistent storage by making a copy.
- Parameters:
-
[in] peer_id The id of the peer whose data to read. [in] data_id Which piece of data to read. [out] p_data Pointer to the peer data. [in,out] p_len_words Length available to copy to (in words). If set to NULL, then no copy will be made and the length will be reflected in p_len_words after the call returns.
- Return values:
-
NRF_SUCCESS The read was successful. NRF_ERROR_INVALID_PARAM Invalid data_id. NRF_ERROR_NULL data contained a NULL pointer. NRF_ERROR_NOT_FOUND The requested data was not found in persistent storage. NRF_ERROR_NO_MEM The length of stored data too large to copy out NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 435 of file peer_data_storage.c.
ret_code_t pds_peer_data_read_ptr_get | ( | pm_peer_id_t | peer_id, |
pm_peer_data_id_t | data_id, | ||
pm_peer_data_flash_t * | p_data, | ||
pm_store_token_t * | p_token | ||
) |
Function for retrieving a direct pointer to peer data in persistent storage.
- Parameters:
-
[in] peer_id The id of the peer whose data to read. [in] data_id Which data to get. [out] p_data The peer data pointer. [out] p_token Token that can be used to lock data in flash and check data validity.
- Return values:
-
NRF_SUCCESS The pointer was successfully retrieved. NRF_ERROR_INVALID_PARAM Invalid data_id. NRF_ERROR_NULL p_data was NULL. NRF_ERROR_NOT_FOUND The requested data was not found in persistent storage. NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 369 of file peer_data_storage.c.
ret_code_t pds_peer_data_update | ( | pm_peer_id_t | peer_id, |
pm_peer_data_const_t const * | peer_data, | ||
pm_store_token_t | old_token, | ||
pm_store_token_t * | p_store_token | ||
) |
Function for updating currently stored peer data to a new version.
Updating happens asynchronously. Expect a PDS_EVT_STORED or PDS_EVT_ERROR_STORE for the store token and a PDS_EVT_ERROR_CLEAR or PDS_EVT_ERROR_CLEAR for the old token
- Parameters:
-
[in] peer_id The peer which the data is associated to. [in] peer_data New data. [in] old_token Store token for the old data. [out] p_store_token Store token for the new data.
- Return values:
-
NRF_SUCESS The update was initiated successfully NRF_ERROR_NOT_FOUND The old store token was invalid. NRF_ERROR_NULL Data contained a NULL pointer. NRF_ERROR_NO_MEM No space available in persistent storage. NRF_ERROR_BUSY FDS or underlying modules are busy and can't take any more requests NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 569 of file peer_data_storage.c.
ret_code_t pds_peer_data_verify | ( | pm_store_token_t | store_token ) |
Function to verify flash data integrity.
- Parameters:
-
[in] store_token The token representing the item to lock
- Return values:
-
NRF_SUCCESS The data integrity is valid. NRF_ERROR_NULL The token is invalid. NRF_ERROR_INVALID_DATA The data integrity is not valid. NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 424 of file peer_data_storage.c.
ret_code_t pds_peer_data_write | ( | pm_peer_id_t | peer_id, |
pm_peer_data_const_t const * | p_peer_data, | ||
pm_store_token_t * | p_store_token | ||
) |
Function for writing peer data to persistent storage.
Writing happens asynchronously. Expect a PDS_EVT_STORED or PDS_EVT_ERROR_STORE event.
- Parameters:
-
[in] peer_id The id of the peer the data pertains to. [in] p_peer_data The peer data. [out] p_store_token A token identifying this particular store operation. The token can be used to identify events pertaining to this operation.
- Return values:
-
NRF_SUCCESS The write was initiated successfully. NRF_ERROR_INVALID_PARAM Invalid data ID or store_flags. NRF_ERROR_NULL Data contained a NULL pointer. NRF_ERROR_NO_MEM No space available in persistent storage. This can only happen if p_prepare_token is NULL. NRF_ERROR_BUSY FDS or underlying modules are busy and can't take any more requests NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 536 of file peer_data_storage.c.
ret_code_t pds_peer_data_write_prepare | ( | pm_peer_data_const_t const * | p_peer_data, |
pm_prepare_token_t * | p_prepare_token | ||
) |
Function for preparing persistent storage for a write.
If this call succeeds, space is reserved in persistent storage, so the write will fit.
- Note:
- If space has already been prepared for this peer_id/data_id pair, no new space will be reserved, unless the previous reservation had too small size.
- Parameters:
-
[in] p_peer_data Data to prepare for. The data needs not be ready, but length and type values must. [out] p_prepare_token A token identifying the prepared memory area.
- Return values:
-
NRF_SUCCESS The call was successful. NRF_ERROR_INVALID_PARAM Invalid data ID. NRF_ERROR_INVALID_LENGTH Data length above the maximum allowed. NRF_ERROR_NO_MEM No space available in persistent storage. NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 473 of file peer_data_storage.c.
ret_code_t pds_peer_data_write_prepare_cancel | ( | pm_prepare_token_t | prepare_token ) |
Function for undoing a previous call to pds_peer_data_write_prepare.
- Parameters:
-
[in] prepare_token A token identifying the prepared memory area to cancel.
- Return values:
-
NRF_SUCCESS The call was successful. NRF_ERROR_NOT_FOUND Invalid peer ID and/or prepare token. NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 488 of file peer_data_storage.c.
ret_code_t pds_peer_data_write_prepared | ( | pm_peer_id_t | peer_id, |
pm_peer_data_const_t const * | p_peer_data, | ||
pm_prepare_token_t | prepare_token, | ||
pm_store_token_t * | p_store_token | ||
) |
Function for writing prepared (reserved) peer data to persistent storage.
Writing happens asynchronously. Expect a PDS_EVT_STORED or PDS_EVT_ERROR_STORE event.
- Parameters:
-
[in] peer_id The id of the peer the data pertains to. [in] p_peer_data The peer data. [in] prepare_token A token identifying the prepared memory area to write into. If the prepare token is invalid, e.g. PDS_PREPARE_TOKEN_INVALID, the prepare/write sequence will happen atomically. [out] p_store_token A token identifying this particular store operation. The token can be used to identify events pertaining to this operation.
- Return values:
-
NRF_SUCCESS The write was initiated successfully. NRF_ERROR_INVALID_PARAM Invalid data ID or store_flags. NRF_ERROR_INVALID_LENGTH Length of data longer than in prepare call. NRF_ERROR_NULL data contained a NULL pointer. NRF_ERROR_NO_MEM No space available in persistent storage. This can only happen if p_prepare_token is NULL. NRF_ERROR_BUSY FDS or underlying modules are busy and can't take any more requests NRF_ERROR_INVALID_STATE Module is not initialized.
Definition at line 500 of file peer_data_storage.c.
pm_peer_id_t pds_peer_id_allocate | ( | void | ) |
Function for claiming an unused peer ID.
- Returns:
- The first unused peer ID.
- Return values:
-
PM_PEER_ID_INVALID If no peer ID is available or module is not initialized.
Definition at line 628 of file peer_data_storage.c.
ret_code_t pds_peer_id_free | ( | pm_peer_id_t | peer_id ) |
Function for freeing a peer ID and clearing all data associated with it in persistent storage.
- Parameters:
-
[in] peer_id Peer ID to free.
- Return values:
-
NRF_SUCCESS The clear was initiated successfully NRF_ERROR_BUSY Another peer_id clear was already requested or fds queue full
Definition at line 639 of file peer_data_storage.c.
bool pds_peer_id_is_allocated | ( | pm_peer_id_t | peer_id ) |
Function for finding out whether a peer ID is in use.
- Parameters:
-
[in] peer_id The peer ID to inquire about.
- Return values:
-
true peer_id is in use. false peer_id is free, or the module is not initialized.
Definition at line 655 of file peer_data_storage.c.
ret_code_t pds_register | ( | pds_evt_handler_t | evt_handler ) |
Function for registering for events from the peer database.
- Note:
- This function will initialize the module if it is not already initialized.
- Parameters:
-
[in] evt_handler Event handler to register.
- Return values:
-
NRF_SUCCESS Registration successful. NRF_ERROR_NO_MEM No more event handlers can be registered. NRF_ERROR_NULL evt_handler was NULL. NRF_ERROR_INVALID_PARAM Unexpected return code from pm_buffer_init. NRF_ERROR_INVALID_STATE FDS has not been initalized.
Definition at line 332 of file peer_data_storage.c.
Generated on Tue Jul 12 2022 19:00:13 by
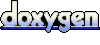