This library lets you control the addressable RGB LED strips from Pololu Robotics & Electronics.
Dependents: WoYaoChengGOng V2-WoYaoChengGOng STM32_MagneticLight tape_Led_Sample ... more
PololuLedStrip.h
00001 #include "mbed.h" 00002 00003 #ifndef _POLOLU_LED_STRIP_H 00004 #define _POLOLU_LED_STRIP_H 00005 00006 namespace Pololu 00007 { 00008 #ifndef _POLOLU_RGB_COLOR 00009 #define _POLOLU_RGB_COLOR 00010 00011 /** Represents an RGB color. */ 00012 typedef struct rgb_color 00013 { 00014 uint8_t red ; /*!< A number between 0 and 255 that represents the brightness of the red component. */ 00015 uint8_t green ; /*!< A number between 0 and 255 that represents the brightness of the green component. */ 00016 uint8_t blue ; /*!< A number between 0 and 255 that represents the brightness of the blue component. */ 00017 } rgb_color; 00018 #endif 00019 00020 extern "C" int led_strip_write_color(rgb_color *, volatile uint32_t * set, volatile uint32_t * clear, uint32_t mask); 00021 00022 /** This class lets you control the addressable RGB LED strips from Pololu</a>, 00023 or any other LED strip based on the TM1804 chip. */ 00024 class PololuLedStrip 00025 { 00026 gpio_t gpio; 00027 00028 public: 00029 00030 /** This constructor lets you make an led strip object by specifying the pin name. 00031 There are no restrictions on what pin you can choose. 00032 00033 Example: 00034 @code 00035 PololuLedStrip ledStrip(p8); 00036 @endcode 00037 */ 00038 PololuLedStrip(PinName pin); 00039 00040 /** Writes the specified series of colors to the LED strip. 00041 @param colors should be a pointer to an array of rgb_color structs. 00042 @param count should be the number of colors to write. 00043 00044 The first color in the array will be written to the LED closest to the data input connector. 00045 To update all the LEDs in the LED strip, count should be equal to or greater than the number of LEDs in the strip. 00046 If count is less than the number of LEDs in the strip, then some LEDs near the end of the strip will not be updated. 00047 00048 The colors are sent in series and each color takes about 45 microseconds to send. 00049 This function disables interrupts temporarily while it is running. 00050 This function waits for over 10 us at the end before returning to allow the colors to take effect. 00051 */ 00052 void write(rgb_color * colors, unsigned int count); 00053 00054 /** This option defaults to <code>false</code>. 00055 Setting this to true changes the behavior of the write function, making it enable interrupts 00056 after each color is sent, about every 60 microseconds. 00057 This allows your program to respond to interrupts faster, but makes it possible for an interrupt 00058 that takes longer than 8 microseconds to screw up the transmission of colors to the LED strip. 00059 00060 Example: 00061 @code 00062 PololuLedStrip::interruptFriendly = true; 00063 @endcode 00064 */ 00065 static bool interruptFriendly; 00066 00067 static void calculateDelays(); 00068 }; 00069 } 00070 00071 using namespace Pololu; 00072 00073 #endif
Generated on Tue Jul 12 2022 20:39:59 by
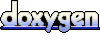