Library to help schedule events to run regularly in the main loop. This library does not have much documentation and is not really intended for public use yet.
Dependents: LineFollowing DeadReckoning
Pacer.h
00001 #pragma once 00002 00003 #include <mbed.h> 00004 00005 class Pacer 00006 { 00007 public: 00008 Pacer(int32_t pace_us) 00009 : pace_us(pace_us), last_time(0) 00010 { 00011 timer.start(); 00012 } 00013 00014 bool ready() 00015 { 00016 return (time() - last_time) >= pace_us; 00017 } 00018 00019 // This should generally only be called when ready() is true. 00020 void advance() 00021 { 00022 last_time += pace_us; 00023 } 00024 00025 void clear() 00026 { 00027 last_time = time(); 00028 } 00029 00030 bool pace() 00031 { 00032 if (ready()) 00033 { 00034 clear(); 00035 return true; 00036 } 00037 else 00038 { 00039 return false; 00040 } 00041 } 00042 00043 private: 00044 uint32_t time() 00045 { 00046 return (uint32_t)timer.read_us(); // Cast int32_t to uint32_t. 00047 } 00048 00049 uint32_t pace_us; 00050 uint32_t last_time; 00051 Timer timer; 00052 };
Generated on Sun Jul 17 2022 15:58:09 by
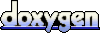