Patched for Audio example - Add status check when DFSDM's filter and channel de-init.
Dependents: DISCO_F413ZH-AUDIO-demo
st7789h2.c
00001 /** 00002 ****************************************************************************** 00003 * @file st7789h2.c 00004 * @author MCD Application Team 00005 * @version V1.1.1 00006 * @date 29-December-2016 00007 * @brief This file includes the LCD driver for st7789h2 LCD. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "st7789h2.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup Components 00046 * @{ 00047 */ 00048 00049 /** @defgroup ST7789H2 00050 * @brief This file provides a set of functions needed to drive the 00051 * FRIDA FRD154BP2901 LCD. 00052 * @{ 00053 */ 00054 00055 /** @defgroup ST7789H2_Private_TypesDefinitions ST7789H2 Private TypesDefinitions 00056 * @{ 00057 */ 00058 typedef struct { 00059 uint8_t red; 00060 uint8_t green; 00061 uint8_t blue; 00062 } ST7789H2_Rgb888; 00063 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup ST7789H2_Private_Defines ST7789H2 Private Defines 00069 * @{ 00070 */ 00071 00072 /** 00073 * @} 00074 */ 00075 00076 /** @defgroup ST7789H2_Private_Macros ST7789H2 Private Macros 00077 * @{ 00078 */ 00079 00080 /** 00081 * @} 00082 */ 00083 00084 /** @defgroup ST7789H2_Private_Variables ST7789H2 Private Variables 00085 * @{ 00086 */ 00087 LCD_DrvTypeDef ST7789H2_drv = 00088 { 00089 ST7789H2_Init, 00090 ST7789H2_ReadID, 00091 ST7789H2_DisplayOn, 00092 ST7789H2_DisplayOff, 00093 ST7789H2_SetCursor, 00094 ST7789H2_WritePixel, 00095 ST7789H2_ReadPixel, 00096 ST7789H2_SetDisplayWindow, 00097 ST7789H2_DrawHLine, 00098 ST7789H2_DrawVLine, 00099 ST7789H2_GetLcdPixelWidth, 00100 ST7789H2_GetLcdPixelHeight, 00101 ST7789H2_DrawBitmap, 00102 ST7789H2_DrawRGBImage, 00103 }; 00104 00105 static uint16_t WindowsXstart = 0; 00106 static uint16_t WindowsYstart = 0; 00107 static uint16_t WindowsXend = ST7789H2_LCD_PIXEL_WIDTH-1; 00108 static uint16_t WindowsYend = ST7789H2_LCD_PIXEL_HEIGHT-1; 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup ST7789H2_Private_FunctionPrototypes ST7789H2 Private FunctionPrototypes 00114 * @{ 00115 */ 00116 static ST7789H2_Rgb888 ST7789H2_ReadPixel_rgb888(uint16_t Xpos, uint16_t Ypos); 00117 static void ST7789H2_DrawRGBHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint8_t *pdata); 00118 00119 /** 00120 * @} 00121 */ 00122 00123 /** @defgroup ST7789H2_Private_Functions ST7789H2 Private Functions 00124 * @{ 00125 */ 00126 00127 /** 00128 * @brief Initialize the st7789h2 LCD Component. 00129 * @param None 00130 * @retval None 00131 */ 00132 void ST7789H2_Init(void) 00133 { 00134 uint8_t parameter[14]; 00135 00136 /* Initialize st7789h2 low level bus layer ----------------------------------*/ 00137 LCD_IO_Init(); 00138 /* Sleep In Command */ 00139 ST7789H2_WriteReg(ST7789H2_SLEEP_IN, (uint8_t*)NULL, 0); 00140 /* Wait for 10ms */ 00141 LCD_IO_Delay(10); 00142 00143 /* SW Reset Command */ 00144 ST7789H2_WriteReg(0x01, (uint8_t*)NULL, 0); 00145 /* Wait for 200ms */ 00146 LCD_IO_Delay(200); 00147 00148 /* Sleep Out Command */ 00149 ST7789H2_WriteReg(ST7789H2_SLEEP_OUT, (uint8_t*)NULL, 0); 00150 /* Wait for 120ms */ 00151 LCD_IO_Delay(120); 00152 00153 /* Normal display for Driver Down side */ 00154 parameter[0] = 0x00; 00155 ST7789H2_WriteReg(ST7789H2_NORMAL_DISPLAY, parameter, 1); 00156 00157 /* Color mode 16bits/pixel */ 00158 parameter[0] = 0x05; 00159 ST7789H2_WriteReg(ST7789H2_COLOR_MODE, parameter, 1); 00160 00161 /* Display inversion On */ 00162 ST7789H2_WriteReg(ST7789H2_DISPLAY_INVERSION, (uint8_t*)NULL, 0); 00163 00164 /* Set Column address CASET */ 00165 parameter[0] = 0x00; 00166 parameter[1] = 0x00; 00167 parameter[2] = 0x00; 00168 parameter[3] = 0xEF; 00169 ST7789H2_WriteReg(ST7789H2_CASET, parameter, 4); 00170 /* Set Row address RASET */ 00171 parameter[0] = 0x00; 00172 parameter[1] = 0x00; 00173 parameter[2] = 0x00; 00174 parameter[3] = 0xEF; 00175 ST7789H2_WriteReg(ST7789H2_RASET, parameter, 4); 00176 00177 /*--------------- ST7789H2 Frame rate setting -------------------------------*/ 00178 /* PORCH control setting */ 00179 parameter[0] = 0x0C; 00180 parameter[1] = 0x0C; 00181 parameter[2] = 0x00; 00182 parameter[3] = 0x33; 00183 parameter[4] = 0x33; 00184 ST7789H2_WriteReg(ST7789H2_PORCH_CTRL, parameter, 5); 00185 00186 /* GATE control setting */ 00187 parameter[0] = 0x35; 00188 ST7789H2_WriteReg(ST7789H2_GATE_CTRL, parameter, 1); 00189 00190 /*--------------- ST7789H2 Power setting ------------------------------------*/ 00191 /* VCOM setting */ 00192 parameter[0] = 0x1F; 00193 ST7789H2_WriteReg(ST7789H2_VCOM_SET, parameter, 1); 00194 00195 /* LCM Control setting */ 00196 parameter[0] = 0x2C; 00197 ST7789H2_WriteReg(ST7789H2_LCM_CTRL, parameter, 1); 00198 00199 /* VDV and VRH Command Enable */ 00200 parameter[0] = 0x01; 00201 parameter[1] = 0xC3; 00202 ST7789H2_WriteReg(ST7789H2_VDV_VRH_EN, parameter, 2); 00203 00204 /* VDV Set */ 00205 parameter[0] = 0x20; 00206 ST7789H2_WriteReg(ST7789H2_VDV_SET, parameter, 1); 00207 00208 /* Frame Rate Control in normal mode */ 00209 parameter[0] = 0x0F; 00210 ST7789H2_WriteReg(ST7789H2_FR_CTRL, parameter, 1); 00211 00212 /* Power Control */ 00213 parameter[0] = 0xA4; 00214 parameter[1] = 0xA1; 00215 ST7789H2_WriteReg(ST7789H2_POWER_CTRL, parameter, 1); 00216 00217 /*--------------- ST7789H2 Gamma setting ------------------------------------*/ 00218 /* Positive Voltage Gamma Control */ 00219 parameter[0] = 0xD0; 00220 parameter[1] = 0x08; 00221 parameter[2] = 0x11; 00222 parameter[3] = 0x08; 00223 parameter[4] = 0x0C; 00224 parameter[5] = 0x15; 00225 parameter[6] = 0x39; 00226 parameter[7] = 0x33; 00227 parameter[8] = 0x50; 00228 parameter[9] = 0x36; 00229 parameter[10] = 0x13; 00230 parameter[11] = 0x14; 00231 parameter[12] = 0x29; 00232 parameter[13] = 0x2D; 00233 ST7789H2_WriteReg(ST7789H2_PV_GAMMA_CTRL, parameter, 14); 00234 00235 /* Negative Voltage Gamma Control */ 00236 parameter[0] = 0xD0; 00237 parameter[1] = 0x08; 00238 parameter[2] = 0x10; 00239 parameter[3] = 0x08; 00240 parameter[4] = 0x06; 00241 parameter[5] = 0x06; 00242 parameter[6] = 0x39; 00243 parameter[7] = 0x44; 00244 parameter[8] = 0x51; 00245 parameter[9] = 0x0B; 00246 parameter[10] = 0x16; 00247 parameter[11] = 0x14; 00248 parameter[12] = 0x2F; 00249 parameter[13] = 0x31; 00250 ST7789H2_WriteReg(ST7789H2_NV_GAMMA_CTRL, parameter, 14); 00251 00252 /* Display ON command */ 00253 ST7789H2_DisplayOn(); 00254 00255 /* Tearing Effect Line On: Option (00h:VSYNC Interface OFF, 01h:VSYNC Interface ON) */ 00256 parameter[0] = 0x00; 00257 ST7789H2_WriteReg(ST7789H2_TEARING_EFFECT, parameter, 1); 00258 00259 } 00260 00261 /** 00262 * @brief Set the Display Orientation. 00263 * @param orientation: ST7789H2_ORIENTATION_PORTRAIT, ST7789H2_ORIENTATION_LANDSCAPE 00264 * or ST7789H2_ORIENTATION_LANDSCAPE_ROT180 00265 * @retval None 00266 */ 00267 void ST7789H2_SetOrientation(uint32_t orientation) 00268 { 00269 uint8_t parameter[6]; 00270 00271 if(orientation == ST7789H2_ORIENTATION_LANDSCAPE) 00272 { 00273 parameter[0] = 0x00; 00274 } 00275 else if(orientation == ST7789H2_ORIENTATION_LANDSCAPE_ROT180) 00276 { 00277 /* Vertical Scrolling Definition */ 00278 /* TFA describes the Top Fixed Area */ 00279 parameter[0] = 0x00; 00280 parameter[1] = 0x00; 00281 /* VSA describes the height of the Vertical Scrolling Area */ 00282 parameter[2] = 0x01; 00283 parameter[3] = 0xF0; 00284 /* BFA describes the Bottom Fixed Area */ 00285 parameter[4] = 0x00; 00286 parameter[5] = 0x00; 00287 ST7789H2_WriteReg(ST7789H2_VSCRDEF, parameter, 6); 00288 00289 /* Vertical Scroll Start Address of RAM */ 00290 /* GRAM row nbr (320) - Display row nbr (240) = 80 = 0x50 */ 00291 parameter[0] = 0x00; 00292 parameter[1] = 0x50; 00293 ST7789H2_WriteReg(ST7789H2_VSCSAD, parameter, 2); 00294 00295 parameter[0] = 0xC0; 00296 } 00297 else 00298 { 00299 parameter[0] = 0x60; 00300 } 00301 ST7789H2_WriteReg(ST7789H2_NORMAL_DISPLAY, parameter, 1); 00302 } 00303 00304 /** 00305 * @brief Enables the Display. 00306 * @param None 00307 * @retval None 00308 */ 00309 void ST7789H2_DisplayOn(void) 00310 { 00311 /* Display ON command */ 00312 ST7789H2_WriteReg(ST7789H2_DISPLAY_ON, (uint8_t*)NULL, 0); 00313 00314 /* Sleep Out command */ 00315 ST7789H2_WriteReg(ST7789H2_SLEEP_OUT, (uint8_t*)NULL, 0); 00316 } 00317 00318 /** 00319 * @brief Disables the Display. 00320 * @param None 00321 * @retval None 00322 */ 00323 void ST7789H2_DisplayOff(void) 00324 { 00325 uint8_t parameter[1]; 00326 parameter[0] = 0xFE; 00327 /* Display OFF command */ 00328 ST7789H2_WriteReg(ST7789H2_DISPLAY_OFF, parameter, 1); 00329 /* Sleep In Command */ 00330 ST7789H2_WriteReg(ST7789H2_SLEEP_IN, (uint8_t*)NULL, 0); 00331 /* Wait for 10ms */ 00332 LCD_IO_Delay(10); 00333 } 00334 00335 /** 00336 * @brief Get the LCD pixel Width. 00337 * @param None 00338 * @retval The Lcd Pixel Width 00339 */ 00340 uint16_t ST7789H2_GetLcdPixelWidth(void) 00341 { 00342 return (uint16_t)ST7789H2_LCD_PIXEL_WIDTH; 00343 } 00344 00345 /** 00346 * @brief Get the LCD pixel Height. 00347 * @param None 00348 * @retval The Lcd Pixel Height 00349 */ 00350 uint16_t ST7789H2_GetLcdPixelHeight(void) 00351 { 00352 return (uint16_t)ST7789H2_LCD_PIXEL_HEIGHT; 00353 } 00354 00355 /** 00356 * @brief Get the st7789h2 ID. 00357 * @param None 00358 * @retval The st7789h2 ID 00359 */ 00360 uint16_t ST7789H2_ReadID(void) 00361 { 00362 LCD_IO_Init(); 00363 00364 return ST7789H2_ReadReg(ST7789H2_LCD_ID); 00365 } 00366 00367 /** 00368 * @brief Set Cursor position. 00369 * @param Xpos: specifies the X position. 00370 * @param Ypos: specifies the Y position. 00371 * @retval None 00372 */ 00373 void ST7789H2_SetCursor(uint16_t Xpos, uint16_t Ypos) 00374 { 00375 uint8_t parameter[4]; 00376 /* CASET: Comumn Addrses Set */ 00377 parameter[0] = 0x00; 00378 parameter[1] = 0x00 + Xpos; 00379 parameter[2] = 0x00; 00380 parameter[3] = 0xEF + Xpos; 00381 ST7789H2_WriteReg(ST7789H2_CASET, parameter, 4); 00382 /* RASET: Row Addrses Set */ 00383 parameter[0] = 0x00; 00384 parameter[1] = 0x00 + Ypos; 00385 parameter[2] = 0x00; 00386 parameter[3] = 0xEF + Ypos; 00387 ST7789H2_WriteReg(ST7789H2_RASET, parameter, 4); 00388 } 00389 00390 /** 00391 * @brief Write pixel. 00392 * @param Xpos: specifies the X position. 00393 * @param Ypos: specifies the Y position. 00394 * @param RGBCode: the RGB pixel color in RGB565 format 00395 * @retval None 00396 */ 00397 void ST7789H2_WritePixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00398 { 00399 /* Set Cursor */ 00400 ST7789H2_SetCursor(Xpos, Ypos); 00401 00402 /* Prepare to write to LCD RAM */ 00403 ST7789H2_WriteReg(ST7789H2_WRITE_RAM, (uint8_t*)NULL, 0); /* RAM write data command */ 00404 00405 /* Write RAM data */ 00406 LCD_IO_WriteData(RGBCode); 00407 } 00408 00409 /** 00410 * @brief Read pixel. 00411 * @param Xpos: specifies the X position. 00412 * @param Ypos: specifies the Y position. 00413 * @retval The RGB pixel color in RGB565 format 00414 */ 00415 uint16_t ST7789H2_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00416 { 00417 ST7789H2_Rgb888 rgb888; 00418 uint8_t r, g, b; 00419 uint16_t rgb565; 00420 00421 /* Set Cursor */ 00422 ST7789H2_SetCursor(Xpos, Ypos); 00423 00424 /* Read RGB888 data from LCD RAM */ 00425 rgb888 = ST7789H2_ReadPixel_rgb888(Xpos, Ypos); 00426 00427 /* Convert RGB888 to RGB565 */ 00428 r = ((rgb888.red & 0xF8) >> 3); /* Extract the red component 5 most significant bits */ 00429 g = ((rgb888.green & 0xFC) >> 2); /* Extract the green component 6 most significant bits */ 00430 b = ((rgb888.blue & 0xF8) >> 3); /* Extract the blue component 5 most significant bits */ 00431 00432 rgb565 = ((uint16_t)(r) << 11) + ((uint16_t)(g) << 5) + ((uint16_t)(b) << 0); 00433 00434 return (rgb565); 00435 } 00436 00437 /** 00438 * @brief Writes to the selected LCD register. 00439 * @param Command: command value (or register address as named in st7789h2 doc). 00440 * @param Parameters: pointer on parameters value (if command uses one or several parameters). 00441 * @param NbParameters: number of command parameters (0 if no parameter) 00442 * @retval None 00443 */ 00444 void ST7789H2_WriteReg(uint8_t Command, uint8_t *Parameters, uint8_t NbParameters) 00445 { 00446 uint8_t i; 00447 00448 /* Send command */ 00449 LCD_IO_WriteReg(Command); 00450 00451 /* Send command's parameters if any */ 00452 for (i=0; i<NbParameters; i++) 00453 { 00454 LCD_IO_WriteData(Parameters[i]); 00455 } 00456 } 00457 00458 /** 00459 * @brief Reads the selected LCD Register. 00460 * @param Command: command value (or register address as named in st7789h2 doc). 00461 * @retval Register Value. 00462 */ 00463 uint8_t ST7789H2_ReadReg(uint8_t Command) 00464 { 00465 /* Send command */ 00466 LCD_IO_WriteReg(Command); 00467 00468 /* Read dummy data */ 00469 LCD_IO_ReadData(); 00470 00471 /* Read register value */ 00472 return (LCD_IO_ReadData()); 00473 } 00474 00475 /** 00476 * @brief Sets a display window 00477 * @param Xpos: specifies the X bottom left position. 00478 * @param Ypos: specifies the Y bottom left position. 00479 * @param Height: display window height. 00480 * @param Width: display window width. 00481 * @retval None 00482 */ 00483 void ST7789H2_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00484 { 00485 if (Xpos < ST7789H2_LCD_PIXEL_WIDTH) 00486 { 00487 WindowsXstart = Xpos; 00488 } 00489 else 00490 { 00491 WindowsXstart = 0; 00492 } 00493 00494 if (Ypos < ST7789H2_LCD_PIXEL_HEIGHT) 00495 { 00496 WindowsYstart = Ypos; 00497 } 00498 else 00499 { 00500 WindowsYstart = 0; 00501 } 00502 00503 if (Width + Xpos <= ST7789H2_LCD_PIXEL_WIDTH) 00504 { 00505 WindowsXend = Width + Xpos - 1; 00506 } 00507 else 00508 { 00509 WindowsXend = ST7789H2_LCD_PIXEL_WIDTH - 1; 00510 } 00511 00512 if (Height + Ypos <= ST7789H2_LCD_PIXEL_HEIGHT) 00513 { 00514 WindowsYend = Height + Ypos - 1; 00515 } 00516 else 00517 { 00518 WindowsYend = ST7789H2_LCD_PIXEL_HEIGHT-1; 00519 } 00520 } 00521 00522 /** 00523 * @brief Draw vertical line. 00524 * @param RGBCode: Specifies the RGB color in RGB565 format 00525 * @param Xpos: specifies the X position. 00526 * @param Ypos: specifies the Y position. 00527 * @param Length: specifies the Line length. 00528 * @retval None 00529 */ 00530 void ST7789H2_DrawHLine(uint16_t RGBCode, uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00531 { 00532 uint16_t counter = 0; 00533 00534 /* Set Cursor */ 00535 ST7789H2_SetCursor(Xpos, Ypos); 00536 00537 /* Prepare to write to LCD RAM */ 00538 ST7789H2_WriteReg(ST7789H2_WRITE_RAM, (uint8_t*)NULL, 0); /* RAM write data command */ 00539 00540 /* Sent a complete line */ 00541 for(counter = 0; counter < Length; counter++) 00542 { 00543 LCD_IO_WriteData(RGBCode); 00544 } 00545 } 00546 00547 /** 00548 * @brief Draw vertical line. 00549 * @param RGBCode: Specifies the RGB color 00550 * @param Xpos: specifies the X position. 00551 * @param Ypos: specifies the Y position. 00552 * @param Length: specifies the Line length. 00553 * @retval None 00554 */ 00555 void ST7789H2_DrawVLine(uint16_t RGBCode, uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00556 { 00557 uint16_t counter = 0; 00558 00559 /* Set Cursor */ 00560 ST7789H2_SetCursor(Xpos, Ypos); 00561 00562 /* Prepare to write to LCD RAM */ 00563 ST7789H2_WriteReg(ST7789H2_WRITE_RAM, (uint8_t*)NULL, 0); /* RAM write data command */ 00564 00565 /* Fill a complete vertical line */ 00566 for(counter = 0; counter < Length; counter++) 00567 { 00568 ST7789H2_WritePixel(Xpos, Ypos + counter, RGBCode); 00569 } 00570 } 00571 00572 /** 00573 * @brief Displays a bitmap picture. 00574 * @param BmpAddress: Bmp picture address. 00575 * @param Xpos: Bmp X position in the LCD 00576 * @param Ypos: Bmp Y position in the LCD 00577 * @retval None 00578 */ 00579 void ST7789H2_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp) 00580 { 00581 uint32_t index = 0, size = 0; 00582 uint32_t posY; 00583 uint32_t nb_line = 0; 00584 uint16_t Xsize = WindowsXend - WindowsXstart + 1; 00585 uint16_t Ysize = WindowsYend - WindowsYstart + 1; 00586 00587 /* Read bitmap size */ 00588 size = *(volatile uint16_t *) (pbmp + 2); 00589 size |= (*(volatile uint16_t *) (pbmp + 4)) << 16; 00590 /* Get bitmap data address offset */ 00591 index = *(volatile uint16_t *) (pbmp + 10); 00592 index |= (*(volatile uint16_t *) (pbmp + 12)) << 16; 00593 size = (size - index)/2; 00594 pbmp += index; 00595 00596 for (posY = (Ypos + Ysize); posY > Ypos; posY--) /* In BMP files the line order is inverted */ 00597 { 00598 /* Set Cursor */ 00599 ST7789H2_SetCursor(Xpos, posY - 1); 00600 00601 /* Draw one line of the picture */ 00602 ST7789H2_DrawRGBHLine(Xpos, posY - 1, Xsize, (pbmp + (nb_line * Xsize * 2))); 00603 nb_line++; 00604 } 00605 } 00606 00607 /** 00608 * @brief Displays picture. 00609 * @param pdata: picture address. 00610 * @param Xpos: Image X position in the LCD 00611 * @param Ypos: Image Y position in the LCD 00612 * @param Xsize: Image X size in the LCD 00613 * @param Ysize: Image Y size in the LCD 00614 * @retval None 00615 */ 00616 void ST7789H2_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pdata) 00617 { 00618 uint32_t posY; 00619 uint32_t nb_line = 0; 00620 00621 for (posY = Ypos; posY < (Ypos + Ysize); posY ++) 00622 { 00623 /* Set Cursor */ 00624 ST7789H2_SetCursor(Xpos, posY); 00625 00626 /* Draw one line of the picture */ 00627 ST7789H2_DrawRGBHLine(Xpos, posY, Xsize, (pdata + (nb_line * Xsize * 2))); 00628 nb_line++; 00629 } 00630 } 00631 00632 00633 /****************************************************************************** 00634 Static Functions 00635 *******************************************************************************/ 00636 00637 /** 00638 * @brief Read pixel from LCD RAM in RGB888 format 00639 * @param Xpos: specifies the X position. 00640 * @param Ypos: specifies the Y position. 00641 * @retval Each RGB pixel color components in a structure 00642 */ 00643 static ST7789H2_Rgb888 ST7789H2_ReadPixel_rgb888(uint16_t Xpos, uint16_t Ypos) 00644 { 00645 ST7789H2_Rgb888 rgb888; 00646 uint16_t rgb888_part1, rgb888_part2; 00647 00648 /* In LCD RAM, pixels are 24 bits packed and read with 16 bits access 00649 * Here is the pixels components arrangement in memory : 00650 * bits: 15 14 13 12 11 10 09 08 | 07 06 05 04 03 02 01 00 00651 * address 0 : red pixel 0 X X | green pixel 0 X X 00652 * address 1 : blue pixel 0 X X | red pixel 1 X X 00653 * address 2 : green pixel 1 X X | blue pixel 1 X X 00654 */ 00655 00656 /* Set Cursor */ 00657 ST7789H2_SetCursor(Xpos, Ypos); 00658 /* Prepare to read LCD RAM */ 00659 ST7789H2_WriteReg(ST7789H2_READ_RAM, (uint8_t*)NULL, 0); /* RAM read data command */ 00660 /* Dummy read */ 00661 LCD_IO_ReadData(); 00662 /* Read first part of the RGB888 data */ 00663 rgb888_part1 = LCD_IO_ReadData(); 00664 /* Read first part of the RGB888 data */ 00665 rgb888_part2 = LCD_IO_ReadData(); 00666 00667 /* red component */ 00668 rgb888.red = (rgb888_part1 & 0xFC00) >> 8; 00669 /* green component */ 00670 rgb888.green = (rgb888_part1 & 0x00FC) >> 0; 00671 /* blue component */ 00672 rgb888.blue = (rgb888_part2 & 0xFC00) >> 8; 00673 00674 return rgb888; 00675 } 00676 00677 00678 /** 00679 * @brief Displays a single picture line. 00680 * @param pdata: picture address. 00681 * @param Xpos: Image X position in the LCD 00682 * @param Ypos: Image Y position in the LCD 00683 * @param Xsize: Image X size in the LCD 00684 * @retval None 00685 */ 00686 static void ST7789H2_DrawRGBHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint8_t *pdata) 00687 { 00688 uint32_t i = 0; 00689 uint32_t posX; 00690 uint16_t *rgb565 = (uint16_t*)pdata; 00691 00692 /* Prepare to write to LCD RAM */ 00693 ST7789H2_WriteReg(ST7789H2_WRITE_RAM, (uint8_t*)NULL, 0); /* RAM write data command */ 00694 00695 for (posX = Xpos; posX < (Xsize + Xpos); posX++) 00696 { 00697 if ((posX >= WindowsXstart) && (Ypos >= WindowsYstart) && /* Check we are in the defined window */ 00698 (posX <= WindowsXend) && (Ypos <= WindowsYend)) 00699 { 00700 if (posX != (Xsize + Xpos)) /* When writing last pixel when size is odd, the third part is not written */ 00701 { 00702 LCD_IO_WriteData(rgb565[i]); 00703 } 00704 i++; 00705 } 00706 } 00707 } 00708 00709 /** 00710 * @} 00711 */ 00712 00713 /** 00714 * @} 00715 */ 00716 00717 /** 00718 * @} 00719 */ 00720 00721 /** 00722 * @} 00723 */ 00724 00725 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Jul 14 2022 12:00:02 by
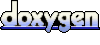