A set of data recording functions to locally store data in a circular buffer, with functions for offloading to an SD Card when convenient. dataRecorderr.h shows accessible functions to the main program - all direct SD operations are abstracted away by the library. When using this library, #include dataRecorder.h
Dependencies: sd-driver_compatible_with_MAX32630FTHR
Fork of CircularBufferSDCardLib by
sdCardReader.cpp
00001 #include "sdCardReader.h" 00002 00003 00004 //helpers 00005 int getSize(int* p){ 00006 printf("reg p %i \n",sizeof(p)); 00007 printf("star p %i \n",sizeof(*p)); 00008 00009 return (sizeof(p)/sizeof(*p)); 00010 } 00011 00012 int getSize(uint16_t* p){ 00013 return (sizeof(p)/sizeof(*p)); 00014 } 00015 00016 //private essential methods 00017 // Try to mount the filesystem 00018 void SDCardReader::mountFileSystem(){ 00019 printf("Mounting the filesystem... "); 00020 fflush(stdout); 00021 int err = m_fs->mount(this); 00022 printf("%s\n", (err ? "Fail :(" : "OK")); 00023 if (err) { 00024 // Reformat if we can't mount the filesystem 00025 // this should only happen on the first boot 00026 printf("No filesystem found, formatting... "); 00027 fflush(stdout); 00028 err = m_fs->reformat(this); 00029 printf("%s\n", (err ? "Fail :(" : "OK")); 00030 if (err) { 00031 error("error: %s (%d)\n", strerror(-err), err); 00032 } 00033 } 00034 } 00035 00036 void SDCardReader::unmountFileSystem(){ 00037 printf("Unmounting... "); 00038 fflush(stdout); 00039 int err = m_fs->unmount(); 00040 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00041 if (err < 0) { 00042 error("error: %s (%d)\n", strerror(-err), err); 00043 } 00044 } 00045 00046 00047 FILE* SDCardReader::openFile(string filename){ 00048 printf("Opening \"/fs/%s\"... ",filename.c_str()); 00049 fflush(stdout); 00050 FILE *f = fopen(filename.c_str(), "r+"); 00051 printf("%s\n", (!f ? "Fail :(" : "OK")); 00052 if (!f) { 00053 // Create the file if it doesn't exist 00054 printf("No file found, creating a new file... "); 00055 fflush(stdout); 00056 f = fopen(filename.c_str(), "w+"); 00057 printf("%s\n", (!f ? "Fail :(" : "OK")); 00058 if (!f) { 00059 error("error: %s (%d)\n", strerror(errno), -errno); 00060 } 00061 } 00062 return f; 00063 } 00064 00065 void SDCardReader::write_uint16_t(uint16_t in_data, bool endline, FILE* fileToUse){ 00066 FILE *f = fileToUse; 00067 printf("%s\n", (!f ? "Fail :(" : "OK")); 00068 00069 int16_t data = (int16_t)in_data; 00070 00071 //printf("\rWriting 16 bit value: % " PRIu16 " ", data); 00072 printf("\rWriting 16 bit value %i ", static_cast<int>(data)); 00073 fflush(stdout); 00074 00075 int err = 0; 00076 //write and check for error 00077 if (endline){ 00078 err = fprintf(f,"%i\n",static_cast<int>(data)); 00079 //err = fprintf(f,"%" PRIu16 "\n",data); 00080 }else{ 00081 err = fprintf(f,"%i " ,static_cast<int>(data)); 00082 } 00083 00084 if (err < 0) { 00085 printf("Fail :(\n"); 00086 error("error: %s (%d)\n", strerror(errno), -errno); 00087 } 00088 } 00089 00090 00091 void SDCardReader::write_uint32_t(uint32_t data, bool endline, FILE* fileToUse){ 00092 FILE *f = fileToUse; 00093 printf("%s\n", (!f ? "Fail :(" : "OK")); 00094 00095 //printf("\rWriting 32 bit value: %" PRIu32 " ", data); 00096 printf("\rWriting 32 bit value %i ", static_cast<int>(data)); 00097 fflush(stdout); 00098 00099 int err = 0; 00100 //write and check for error 00101 if (endline){ 00102 //err = fprintf(f,"%" PRIu32 "\n",data); 00103 err = fprintf(f,"%i\n",static_cast<int>(data)); 00104 }else{ 00105 err = fprintf(f,"%i ",static_cast<int>(data)); 00106 } 00107 00108 if (err < 0) { 00109 printf("Fail :(\n"); 00110 error("error: %s (%d)\n", strerror(errno), -errno); 00111 } 00112 } 00113 00114 00115 void SDCardReader::closeFile(FILE* fileToClose){ 00116 // Close the file which also flushes any cached writes 00117 printf("Closing file..."); 00118 fflush(stdout); 00119 00120 int err = 0; 00121 err = fclose(fileToClose); 00122 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00123 if (err < 0) { 00124 error("error: %s (%d)\n", strerror(errno), -errno); 00125 } 00126 } 00127 00128 int SDCardReader::fullWriteProcedure(string filename,vector<int> indexArr, vector<uint32_t> timeArr, vector<vector <uint16_t> > allData){ 00129 mountFileSystem(); 00130 FILE* theFile = openFile(filename); 00131 00132 printf("The size of indexArr is %i \n",indexArr.size()); 00133 00134 for (unsigned int i = 0; i< indexArr.size(); i++){ 00135 writeDataPoint(theFile,indexArr[i],timeArr[i], allData[i]); 00136 } 00137 00138 closeFile(theFile); 00139 unmountFileSystem(); 00140 00141 return 1; 00142 } 00143 00144 //use circular buffers instead of strings 00145 //write as 1st column, index. 2nd column timestamp. 3rd column data1. 4th data2. etc. 00146 //pass in array of circular buffers 00147 int SDCardReader::writeDataPoint(FILE* theFile, int index, uint32_t timestamp, vector<uint16_t> data){ 00148 // FILE* theFile = openFile(filename); 00149 00150 //write index 00151 write_uint16_t(index, false, theFile); 00152 //write timestamp 00153 write_uint32_t(timestamp, false, theFile); 00154 00155 //write data 00156 for (unsigned int i = 0; i < data.size()-1; i++) { 00157 write_uint16_t(data[i], false, theFile); 00158 } 00159 write_uint16_t(data[data.size()-1], true, theFile); 00160 return 0; 00161 } 00162 00163 int SDCardReader::eraseData(){ 00164 printf("Initializing the block device... "); 00165 fflush(stdout); 00166 int err = this->init(); 00167 printf("%s\n", (err ? "Fail :(" : "OK")); 00168 if (err) { 00169 error("error: %s (%d)\n", strerror(-err), err); 00170 } 00171 00172 printf("Erasing the block device... "); 00173 fflush(stdout); 00174 err = this->erase(0, this->size()); 00175 printf("%s\n", (err ? "Fail :(" : "OK")); 00176 if (err) { 00177 error("error: %s (%d)\n", strerror(-err), err); 00178 } 00179 00180 printf("Deinitializing the block device... "); 00181 fflush(stdout); 00182 err = this->deinit(); 00183 printf("%s\n", (err ? "Fail :(" : "OK")); 00184 if (err) { 00185 error("error: %s (%d)\n", strerror(-err), err); 00186 } 00187 00188 return err; 00189 } 00190 00191 //Methods for reading sdcard files. Mostly to read off trial numbers 00192 int SDCardReader::readAndIncrement(string filename){ 00193 mountFileSystem(); 00194 00195 FILE* theFile = openFile(filename); 00196 // Get current stream position 00197 long pos = ftell(theFile); 00198 // Parse out the number 00199 int32_t number; 00200 int32_t presentNumber; 00201 00202 fscanf(theFile, "%d", &number); 00203 presentNumber = number; 00204 number += 1; 00205 //read lone value as int, return it 00206 // Seek to beginning of number 00207 fseek(theFile, pos, SEEK_SET); 00208 // Store number 00209 fprintf(theFile, "%d\n", number); 00210 // Flush between write and read on same file 00211 fflush(theFile); 00212 00213 closeFile(theFile); 00214 unmountFileSystem(); 00215 return (int)presentNumber; 00216 } 00217
Generated on Fri Jul 15 2022 02:01:26 by
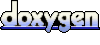