A set of data recording functions to locally store data in a circular buffer, with functions for offloading to an SD Card when convenient. dataRecorderr.h shows accessible functions to the main program - all direct SD operations are abstracted away by the library. When using this library, #include dataRecorder.h
Dependencies: sd-driver_compatible_with_MAX32630FTHR
Fork of CircularBufferSDCardLib by
exampleMain.cpp
00001 /* 00002 00003 #include "mbed.h" 00004 #include "dataRecorder.h" 00005 00006 DataRecorder* boba = new DataRecorder(); 00007 00008 InterruptIn irq(PF_2); 00009 DigitalOut led1(PE_0); 00010 00011 00012 int main() { 00013 00014 printf("We are in! \n"); 00015 00016 uint16_t a = 123; 00017 uint16_t b = 2034; 00018 uint16_t c = 1040; 00019 uint16_t d = 8; 00020 uint16_t e = 980; 00021 00022 uint32_t ta = 2121; 00023 uint32_t tb = 8181; 00024 uint32_t tc = 6262; 00025 uint32_t td = 3535; 00026 uint32_t te = 1212; 00027 00028 boba->logDistancePoint(a); 00029 boba->logDistancePoint(b); 00030 boba->logDistancePoint(c); 00031 boba->logDistancePoint(d); 00032 boba->logDistancePoint(e); 00033 00034 boba->logTimeStamp(ta); 00035 boba->logTimeStamp(tb); 00036 boba->logTimeStamp(tc); 00037 boba->logTimeStamp(td); 00038 boba->logTimeStamp(te); 00039 00040 00041 printf("about to save\n"); 00042 00043 boba->saveLoggedDataAndClearBuffer("/fs/SUPERDATAYO.txt"); 00044 00045 printf("saved\n"); 00046 00047 led1 = !led1; 00048 } 00049 00050 */
Generated on Fri Jul 15 2022 02:01:26 by
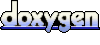