A set of data recording functions to locally store data in a circular buffer, with functions for offloading to an SD Card when convenient. dataRecorderr.h shows accessible functions to the main program - all direct SD operations are abstracted away by the library. When using this library, #include dataRecorder.h
Dependencies: sd-driver_compatible_with_MAX32630FTHR
Fork of CircularBufferSDCardLib by
dataRecorder.h
00001 #ifndef _DATARECORDER_H_ 00002 #define _DATARECORDER_H_ 00003 00004 #include "mbed.h" 00005 #include "platform/CircularBuffer.h" 00006 #include "sdCardReader.h" 00007 00008 00009 //converts number to string 00010 #include <sstream> 00011 #define SSTR( x ) static_cast< std::ostringstream & >(( std::ostringstream() << std::dec << x ) ).str() 00012 00013 // Define circular buffer size 00014 #define BUF_SIZE 10000 00015 00016 00017 /** Data Recorder is a class that stores data measurements in a ring buffer. 00018 It contains an SD Card Reader with a filesystem to store measurements when required **/ 00019 00020 class DataRecorder{ 00021 public: 00022 DataRecorder(int numDataFields); 00023 /** Get Information **/ 00024 // gets the number of values stored in the circular buffer 00025 int getDataQuantity(); 00026 int getTimeStampQuantity(); 00027 00028 // pops the last value off of the circular buffer 00029 uint16_t popLastDataPoint(); 00030 uint32_t popLastTimeStamp(); 00031 00032 /** Set Information **/ 00033 void logDistancePoint(uint16_t value); 00034 void logTimeStamp(uint32_t value); 00035 00036 /** Save logged data to SD card **/ 00037 void saveLoggedData(string filename); 00038 //saves buffer to sd card and clears it 00039 void saveLoggedDataAndClearBuffer(string filename); 00040 00041 int getTrialNumAndIncrement(); 00042 00043 00044 private: 00045 00046 void savePoint(uint16_t value); 00047 // input values are converted to char arrays 00048 // iterate over char array to add values to buffer 00049 // buffer contents it turned into a uint8_t array and written to 00050 // sd card 00051 00052 /* Buffers for Data Storage */ 00053 CircularBuffer<uint16_t, BUF_SIZE> m_buf_data; 00054 CircularBuffer<uint32_t, BUF_SIZE> m_buf_timestamp; 00055 00056 // Count for buffers 00057 int m_data_quantity; 00058 int m_time_quantity; 00059 00060 /* SD Card Interface Object */ 00061 SDCardReader* m_saveBuddy; 00062 00063 int m_numDataFields; 00064 00065 00066 00067 00068 //specialized buffers 00069 //CircularBuffer<uint16_t, BUF_SIZE> buf_distance; 00070 //CircularBuffer<uint16_t, BUF_SIZE> buf_kalmanAngle; 00071 //CircularBuffer<uint32_t, BUF_SIZE> buf_timestamp; 00072 00073 /*CircularBuffer<char, BUF_SIZE> buf_accX; 00074 CircularBuffer<char, BUF_SIZE> buf_accY; 00075 CircularBuffer<char, BUF_SIZE> buf_accZ; 00076 CircularBuffer<char, BUF_SIZE> buf_gyroX; 00077 CircularBuffer<char, BUF_SIZE> buf_gyroY; 00078 CircularBuffer<char, BUF_SIZE> buf_gyroZ;*/ 00079 00080 //sdcard blocks 00081 //uint8_t blockDistance[4096]; 00082 //int blockDistance_Index; 00083 00084 // clear pops all elements out of buffer. 00085 /*CircularBuffer<char, BUF_SIZE> buf; 00086 char data_stream[];// = "DataToBeAddedToBuffer"; 00087 uint32_t bytes_written;// = 0; 00088 int m; 00089 const uint8_t separator = ' ';*/ 00090 00091 }; 00092 00093 00094 #endif
Generated on Fri Jul 15 2022 02:01:26 by
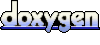