A set of data recording functions to locally store data in a circular buffer, with functions for offloading to an SD Card when convenient. dataRecorderr.h shows accessible functions to the main program - all direct SD operations are abstracted away by the library. When using this library, #include dataRecorder.h
Dependencies: sd-driver_compatible_with_MAX32630FTHR
Fork of CircularBufferSDCardLib by
dataRecorder.cpp
00001 #include "dataRecorder.h" 00002 00003 /* CONSTRUCTOR*/ 00004 DataRecorder::DataRecorder(int numDataFields){ 00005 //Initialize SDCard Reader Class 00006 m_saveBuddy = new SDCardReader(); 00007 00008 //Initialize data quantity as 0 00009 m_data_quantity = 0; 00010 00011 //initialize num fields 00012 m_numDataFields = numDataFields; 00013 } 00014 00015 00016 /* PUBLIC METHODS */ 00017 00018 int DataRecorder::getDataQuantity(){ 00019 return m_data_quantity; 00020 } 00021 00022 int DataRecorder::getTimeStampQuantity(){ 00023 return m_time_quantity; 00024 } 00025 00026 00027 uint16_t DataRecorder::popLastDataPoint(){ 00028 //printf("pop data\n"); 00029 00030 m_data_quantity--; 00031 if (m_data_quantity<0){ 00032 m_data_quantity=0; 00033 } 00034 uint16_t poppedData; 00035 if(!m_buf_data.empty()){ 00036 m_buf_data.pop(poppedData); 00037 return poppedData; 00038 }else{ 00039 return (uint16_t)0; 00040 } 00041 00042 } 00043 00044 uint32_t DataRecorder::popLastTimeStamp(){ 00045 //printf("pop time\n"); 00046 m_time_quantity--; 00047 if (m_time_quantity<0){ 00048 m_time_quantity=0; 00049 } 00050 00051 uint32_t poppedData; 00052 if(!m_buf_timestamp.empty()){ 00053 m_buf_timestamp.pop(poppedData); 00054 return poppedData; 00055 }else{ 00056 return (uint16_t)0; 00057 } 00058 00059 } 00060 00061 void DataRecorder::logDistancePoint(uint16_t value){ 00062 //printf("push distance\n"); 00063 m_data_quantity++; 00064 if (m_data_quantity>BUF_SIZE){ 00065 m_data_quantity=BUF_SIZE; 00066 } 00067 m_buf_data.push(value); 00068 } 00069 00070 void DataRecorder::logTimeStamp(uint32_t value){ 00071 //printf("push time\n"); 00072 m_time_quantity++; 00073 if (m_time_quantity>BUF_SIZE){ 00074 m_time_quantity=BUF_SIZE; 00075 } 00076 m_buf_timestamp.push(value); 00077 } 00078 00079 //helpers 00080 int getoSize(int* p){ 00081 printf("reg p %i \n",sizeof(p)); 00082 printf("star p %i \n",sizeof(*p)); 00083 00084 return (sizeof(p)/sizeof(*p)); 00085 } 00086 00087 int getoSize(uint16_t* p){ 00088 return (sizeof(p)/sizeof(*p)); 00089 } 00090 00091 00092 /** Save logged data to SD card **/ 00093 void DataRecorder::saveLoggedData(string filename){ 00094 //iterate over buffer and use the sd card commands 00095 00096 printf("before logging, data quantity is %i\n",m_data_quantity); 00097 00098 int numPoints = m_data_quantity/m_numDataFields; //add check for timestamps 00099 00100 printf("numPoints %i \n ",numPoints); 00101 00102 vector<int> indexArr(numPoints); 00103 //int indexArr[numPoints]; 00104 00105 //index setting 00106 for (int i = numPoints-1; i >=0 ; i--){ 00107 indexArr[i] = i; 00108 } 00109 00110 vector<uint32_t> timeArr(numPoints); 00111 vector<uint16_t> dataEntry(m_numDataFields); //created before loop to see if prevent data collision 00112 vector<vector <uint16_t> > allDataArr(numPoints); 00113 00114 //uint32_t timeArr[numPoints]; 00115 //uint16_t* allDataArr[numPoints]; 00116 00117 for (int i = 0; i < numPoints; i++){ 00118 //time aggregation 00119 timeArr[i] = popLastTimeStamp(); 00120 00121 //data aggregation 00122 // vector<uint16_t> dataEntry(m_numDataFields); 00123 for (int j=0;j<m_numDataFields;j++){ 00124 dataEntry[j] = popLastDataPoint(); 00125 printf("%i\n",dataEntry[j]); 00126 } 00127 allDataArr[i] = dataEntry; 00128 // printf("%i\n",static_cast<int>(dataEntry[0])); 00129 } 00130 00131 printf("Before launch! size test %i \n",indexArr.size()); 00132 00133 m_saveBuddy->fullWriteProcedure(filename,indexArr,timeArr,allDataArr); 00134 } 00135 //saves buffer to sd card and clears it 00136 void DataRecorder::saveLoggedDataAndClearBuffer(string filename){ 00137 saveLoggedData(filename); 00138 // then eraseBuffers(); 00139 } 00140 00141 int DataRecorder::getTrialNumAndIncrement(){ 00142 return m_saveBuddy->readAndIncrement("/fs/trialNum.txt"); 00143 }
Generated on Fri Jul 15 2022 02:01:26 by
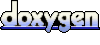