mbed.org implementation of the abstract SmartREST library for the Cumulocity Platform SmartREST protocol.
Dependents: MbedSmartRestMain MbedSmartRestMain
AbstractDataSink.h
00001 /* 00002 * AbstractDataSink.h 00003 * 00004 * Created on: Nov 1, 2013 00005 * * Authors: Vincent Wochnik <v.wochnik@gmail.com> 00006 * 00007 * Copyright (c) 2013 Cumulocity GmbH 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining 00010 * a copy of this software and associated documentation files (the 00011 * "Software"), to deal in the Software without restriction, including 00012 * without limitation the rights to use, copy, modify, merge, publish, 00013 * distribute, sublicense, and/or sell copies of the Software, and to 00014 * permit persons to whom the Software is furnished to do so, subject to 00015 * the following conditions: 00016 * 00017 * The above copyright notice and this permission notice shall be 00018 * included in all copies or substantial portions of the Software. 00019 * 00020 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, 00021 * EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00022 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00023 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE 00024 * LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION 00025 * OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION 00026 * WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00027 */ 00028 00029 /** 00030 * @file AbstractDataSink.h 00031 * An abstraction for a data sink which can be used to send data 00032 * over a connection. 00033 * 00034 * Following write actions are supported: 00035 * - write a character 00036 * - write a buffer of certain length 00037 * - write a character string 00038 * - write an unsigned number 00039 */ 00040 00041 #ifndef ABSTRACTDATASINK_H 00042 #define ABSTRACTDATASINK_H 00043 00044 #include "config.h" 00045 #include <stddef.h> 00046 00047 /* 00048 * The AbstractDataSink class provides a way to write data to a 00049 * connection. 00050 */ 00051 class AbstractDataSink 00052 { 00053 public: 00054 virtual ~AbstractDataSink() { }; 00055 00056 /** 00057 * Writes a single character. 00058 * @param c the character to write 00059 * @return the number of characters written, in this case 1 or 0 00060 */ 00061 virtual size_t write(char) = 0; 00062 00063 /** 00064 * Writes a buffer. 00065 * @param buf the buffer to write 00066 * @param length the length of the buffer in bytes 00067 * @return the number of characters written 00068 */ 00069 virtual size_t write(void*, size_t) = 0; 00070 00071 /** 00072 * Writes a character string. 00073 * @param str the string to write 00074 * @return the number of characters written 00075 */ 00076 virtual size_t write(const char*) = 0; 00077 00078 /** 00079 * Writes a number with base 10. 00080 * @param number the number to write 00081 * @return the number of digits written 00082 */ 00083 virtual size_t write(unsigned long) = 0; 00084 }; 00085 00086 #endif
Generated on Tue Jul 12 2022 15:21:50 by
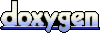