
An floppy drive audio generator using dsp on live audio
Dependencies: Terminal asyncADC mbed-dsp mbed
moppy.cpp
00001 #include "mbed.h" 00002 #include "moppy.h" 00003 00004 #define SILENCE_ALL 250 00005 #define RESET_ALL 251 00006 #define RESET_COMPLETE 252 00007 #define ERROR_STATE 253 00008 #define RESERVED 254 00009 #define SYNC 255 00010 00011 void Moppy::consumeAndCheck(int event) { 00012 while(expectedSyncs-->0) { 00013 if(str_buffer[expectedSyncs]!=0) 00014 error("Moppy Flush Error"); 00015 } 00016 } 00017 00018 Moppy::Moppy(PinName tx, PinName rx, int baud) : serial(tx,rx,baud) { 00019 00020 wait_ms(50); 00021 00022 //Synchronise 00023 serial.putc(SYNC); 00024 if(serial.getc()!=SYNC) 00025 error("Moppy Initialisation Error"); 00026 00027 //Reset all 00028 serial.putc(RESET_ALL); 00029 if(serial.getc()!=RESET_ALL) 00030 error("Failed to reset all drives"); 00031 serial.putc(RESET_COMPLETE); 00032 if(serial.getc()!=RESET_COMPLETE) 00033 error("Failed to reset all drives"); 00034 00035 //Count 00036 serial.putc(249); 00037 driveCount = (249-serial.getc())*2; 00038 serial.putc(SYNC); 00039 if(serial.getc()!=SYNC) 00040 error("Moppy Initialisation Error"); 00041 00042 str_buffer = (char*)calloc(driveCount/2, sizeof(uint8_t)*6); 00043 00044 freqs = (uint16_t*)calloc(driveCount, sizeof(uint16_t)); 00045 00046 pair_changed = (bool*)calloc(driveCount/2, sizeof(bool)); 00047 00048 expectedSyncs = 0; 00049 read_callback.attach(this, &Moppy::consumeAndCheck); 00050 00051 } 00052 00053 void Moppy::setFrequency(int drive, int frequency) { 00054 00055 freqs[drive] = frequency; 00056 pair_changed[drive>>1] = true; 00057 00058 } 00059 00060 void Moppy::flush() { 00061 00062 //build message 00063 int i=driveCount>>1; 00064 int j=0; 00065 while(i-->0) { 00066 if(pair_changed[i]) { 00067 int freq1 = freqs[i<<1]; 00068 int freq2 = freqs[(i<<1)+1]; 00069 00070 str_buffer[j++] = i; 00071 str_buffer[j++] = freq1/250; 00072 str_buffer[j++] = freq1%250; 00073 str_buffer[j++] = freq2/250; 00074 str_buffer[j++] = freq2%250; 00075 str_buffer[j++] = SYNC; 00076 00077 pair_changed[i] = false; //reset changed flag 00078 } 00079 } 00080 00081 //send message asynchronously 00082 if(j>0) { 00083 00084 for(int i=0; i<j; i++) { 00085 serial.putc(str_buffer[i]); 00086 if(str_buffer[i]==SYNC) 00087 serial.getc(); 00088 } 00089 00090 // serial.write((uint8_t*)str_buffer, j, NULL); 00091 // expectedSyncs = j/6; 00092 // serial.read((uint8_t*)str_buffer, expectedSyncs, read_callback); 00093 } 00094 00095 } 00096 00097 bool Moppy::silence() { 00098 serial.putc(250); 00099 return serial.getc()==250; 00100 }
Generated on Thu Jul 21 2022 02:01:04 by
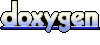