Buffered Serial Port Driver for RTOS
Dependents: nucleo_cannonball PiballNeoController
SerialDriver Class Reference
RTOS compatible buffered Serial port driver. More...
#include <SerialDriver.h>
Public Member Functions | |
SerialDriver (PinName txPin, PinName rxPin, int txBufferLength_=256, int rxBufferLength_=256, unsigned char *txBuffer_=NULL, unsigned char *rxBuffer_=NULL) | |
Prepares ring buffer and irq. | |
int | putc (int c, unsigned int timeoutMs=osWaitForever) |
Put a byte to the TX buffer. | |
int | getc (unsigned int timeoutMs=osWaitForever) |
Get a byte from the RX buffer. | |
int | write (const unsigned char *buffer, const unsigned int length, bool block=true) |
write a buck of bytes | |
int | read (unsigned char *buffer, const unsigned int length, bool block=true) |
read a buck of bytes | |
int | puts (const char *str, bool block=true) |
Write a string (without terminating null) | |
int | printf (const char *format,...) |
Print a formatted string. | |
bool | isTxBufferFull () |
Checks if TX buffer is full. | |
bool | isRxBufferFull () |
Checks if RX buffer is full. | |
bool | isTxBufferEmtpy () |
Checks if TX buffer is empty. | |
bool | isRxBufferEmpty () |
Checks if RX buffer is empty. | |
bool | writeable () |
Checks if TX buffer is writeable (= not full). | |
bool | readable () |
Checks if RX buffer is readable (= not empty). | |
int | getNumTxDrops () |
Returns number of dropped bytes that did not fit into TX buffer. | |
int | getNumRxDrops () |
Returns number of dropped bytes that did not fit into RX buffer. |
Detailed Description
RTOS compatible buffered Serial port driver.
- Based on RawSerial.
- Can use external buffers.
- ISR driven, ring buffered IO operation
- Can Replace mbed RawSerial
- IO operations are idle waiting, don't waste time in RTOS :D
- Do not use attach methods for TxIrq or RxIrq! They are already in use.
Definition at line 32 of file SerialDriver.h.
Constructor & Destructor Documentation
SerialDriver | ( | PinName | txPin, |
PinName | rxPin, | ||
int | txBufferLength_ = 256 , |
||
int | rxBufferLength_ = 256 , |
||
unsigned char * | txBuffer_ = NULL , |
||
unsigned char * | rxBuffer_ = NULL |
||
) |
Prepares ring buffer and irq.
If no buffer was set, the buffer gets allocated.
- Parameters:
-
txPin TX PinName, e.g. USBTX rxPin RX PinName, e.g. USBRX txBufferLength_ size of TX buffer. Must be > 1! rxBufferLength_ size of RX buffer. Must be > 1! txBuffer_ TX buffer, if NULL, the buffer will be allocated rxBuffer_ RX buffer, if NULL, the buffer will be allocated
Definition at line 3 of file SerialDriver.cpp.
Member Function Documentation
int getc | ( | unsigned int | timeoutMs = osWaitForever ) |
Get a byte from the RX buffer.
If the RX buffer is empty, it waits the defined timeout.
- Parameters:
-
timeoutMs give RX buffer time to get readable.
- Returns:
- next byte from RX buffer or -1 after timeout.
Definition at line 113 of file SerialDriver.cpp.
int getNumRxDrops | ( | ) |
Returns number of dropped bytes that did not fit into RX buffer.
- Returns:
- number of dropped rx bytes
Definition at line 183 of file SerialDriver.h.
int getNumTxDrops | ( | ) |
Returns number of dropped bytes that did not fit into TX buffer.
- Returns:
- number of dropped tx bytes
Definition at line 179 of file SerialDriver.h.
bool isRxBufferEmpty | ( | ) |
Checks if RX buffer is empty.
- Returns:
- true - RX buffer is empty, false - else
Definition at line 161 of file SerialDriver.h.
bool isRxBufferFull | ( | ) |
Checks if RX buffer is full.
- Returns:
- true - RX buffer is full, false - else
Definition at line 153 of file SerialDriver.h.
bool isTxBufferEmtpy | ( | ) |
Checks if TX buffer is empty.
- Returns:
- true - TX buffer is empty, false - else
Definition at line 157 of file SerialDriver.h.
bool isTxBufferFull | ( | ) |
Checks if TX buffer is full.
- Returns:
- true - TX buffer is full, false - else
Definition at line 149 of file SerialDriver.h.
int printf | ( | const char * | format, |
... | |||
) |
Print a formatted string.
Idle blocking! Dynamically allocates needed buffer.
- Parameters:
-
format null terminated format string
- Returns:
- written chars (without terminating null)
Definition at line 211 of file SerialDriver.cpp.
int putc | ( | int | c, |
unsigned int | timeoutMs = osWaitForever |
||
) |
Put a byte to the TX buffer.
If the TX buffer is full, it waits the defined timeout. Drops the byte, if TX buffer is still full after timeout.
- Parameters:
-
c The byte to write timeoutMs give TX buffer time to get writeable.
- Returns:
- 1 - success, 0 - if TX Buffer was full all the time
Definition at line 50 of file SerialDriver.cpp.
int puts | ( | const char * | str, |
bool | block = true |
||
) |
Write a string (without terminating null)
For compatibility with mbed RawSerial
- Parameters:
-
str null terminated string block idle wait for every putc to complete
- Returns:
- written chars (without terminating null)
Definition at line 204 of file SerialDriver.cpp.
int read | ( | unsigned char * | buffer, |
const unsigned int | length, | ||
bool | block = true |
||
) |
read a buck of bytes
No timeout! To block, or not to block. That is the question.
- Parameters:
-
buffer buck of bytes length read how much bytes? block idle wait for every getc to complete
- Returns:
- read bytes. For non block read it could be < length!
Definition at line 188 of file SerialDriver.cpp.
bool readable | ( | ) |
Checks if RX buffer is readable (= not empty).
For compatibility with mbed Serial.
- Returns:
- true - RX buffer is readable, false - else
Definition at line 174 of file SerialDriver.h.
int write | ( | const unsigned char * | buffer, |
const unsigned int | length, | ||
bool | block = true |
||
) |
write a buck of bytes
No timeout! To block, or not to block. That is the question.
- Parameters:
-
buffer buck of bytes length write how much bytes? block idle wait for every putc to complete
- Returns:
- written bytes. For non block write it could be < length!
Definition at line 178 of file SerialDriver.cpp.
bool writeable | ( | ) |
Checks if TX buffer is writeable (= not full).
For compatibility with mbed Serial.
- Returns:
- true - TX buffer is writeable, false - else
Definition at line 168 of file SerialDriver.h.
Generated on Tue Jul 12 2022 15:12:29 by
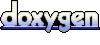