Buffered Serial Port Driver for RTOS
Dependents: nucleo_cannonball PiballNeoController
Example_Bridge.cpp
00001 /// @file Example_Bridge.cpp 00002 /// @brief Forwarding every byte from USB to uart and vice versa 00003 /// 00004 /// - Uses SerialDriver with USBTX and USBRX. 00005 /// - Uses SerialDriver with p13 and p14. 00006 /// 00007 /// - LED1 indicates forwarding pc to uart works 00008 /// - LED2 indicates forwarding uart to pc works 00009 /// - LED4 indicates a parallel thread running 00010 /// 00011 /// - connect p13 with p14 to get a hardware null modem ;) 00012 00013 #if 0 00014 00015 #include "SerialDriver.h" 00016 00017 SerialDriver pc(USBTX, USBRX); 00018 SerialDriver uart(p13, p14); 00019 DigitalOut led1(LED1), led2(LED2), led4(LED4); 00020 00021 // This thread forwards from pc to uart 00022 void forwardPc(void const * argument) 00023 { 00024 // Sometimes You're the Hammer, 00025 while(1) 00026 { 00027 uart.putc(pc.getc()); 00028 led1= !led1; 00029 } 00030 } 00031 00032 // This thread forwards from uart to pc 00033 void forwardUart(void const * argument) 00034 { 00035 // Sometimes You're the Nail 00036 while(1) 00037 { 00038 pc.putc(uart.getc()); 00039 led2= !led2; 00040 } 00041 } 00042 00043 int main() 00044 { 00045 // setup serial ports 00046 pc.baud(9600); 00047 uart.baud(38400); 00048 00049 // Start the forwarding threads 00050 Thread forwardPcThread(&forwardPc); 00051 Thread forwardUartThread(&forwardUart); 00052 00053 // Do something else now 00054 while(1) 00055 { 00056 Thread::wait(20); 00057 led4= !led4; 00058 } 00059 } 00060 00061 #endif 00062
Generated on Tue Jul 12 2022 15:12:29 by
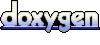