
Servo file under construction.
Embed:
(wiki syntax)
Show/hide line numbers
Servo.h
00001 #include "stdint.h" 00002 #include "mbed.h" 00003 00004 class Servo 00005 { 00006 private: 00007 PwmOut SignalLine; 00008 00009 float Period; 00010 float UpperRange; 00011 float Center; 00012 float LowerRange; 00013 float Position; 00014 00015 public: 00016 Servo(PinName sgnl); 00017 //constructor sets common servo defaults 00018 void position(float ratio); 00019 //set normalized servo position (-1 to 1), default = 0 00020 float position(); 00021 //get normalized servo position (-1 to 1) 00022 void maximum(float seconds); 00023 void maximum_ms(int milliseconds); 00024 void maximum_us(int microseconds); 00025 //set maximum deflection pulse width, default = 2400 us 00026 void center(float seconds); 00027 void center_ms(int milliseconds); 00028 void center_us(int microseconds); 00029 //set center deflection pulse width, default = 1500 us 00030 void minimum(float seconds); 00031 void minimum_ms(int milliseconds); 00032 void minimum_us(int microseconds); 00033 //set minimum deflection pulse width, default = 600 us 00034 void period(float seconds); 00035 void period_ms(int milliseconds); 00036 void period_us(int microseconds); 00037 //set the period, default = 20000 us 00038 void pulsewidth(float seconds); 00039 void pulsewidth_ms(int milliseconds); 00040 void pulsewidth_us(int microseconds); 00041 //set the pulse width, default = 1500 us 00042 float operator =(float assignment); 00043 //shorthand for position setting; 00044 //ex: "ServoObj = 0.5;" will set servo deflection to +0.5 00045 operator float(); 00046 //shorthand for position reading; 00047 // ex: "float check = ServoObj;" will get the current servo deflection 00048 };
Generated on Fri Jul 22 2022 19:30:52 by
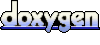