Yet another WS2812 driver, uses the BusrtSPI library. Less features than the PixelArray library but I felt like making my own version.
wsDrive.cpp
00001 #include "wsDrive.h" 00002 00003 wsDrive::wsDrive(PinName mosi, PinName miso, PinName clk) : BurstSPI(mosi,miso,clk) 00004 { 00005 frequency(2400000); 00006 format(12); 00007 pixArray = NULL; 00008 pixelLen = 0; 00009 pixArray16 = NULL; 00010 } 00011 00012 void wsDrive::setData(pixelInfo *dataStart, uint16_t dataLen) 00013 { 00014 pixArray = dataStart; 00015 pixelLen = dataLen; 00016 pixArray16 = NULL; 00017 } 00018 00019 void wsDrive::setData(pixelInfo16 *dataStart, uint16_t dataLen) 00020 { 00021 pixArray16 = dataStart; 00022 pixelLen = dataLen; 00023 pixArray = NULL; 00024 } 00025 00026 void wsDrive::sendData() 00027 { 00028 frequency(2400000); 00029 format(12); 00030 setFormat(); 00031 00032 uint16_t pixIndex = 0; 00033 if (pixArray) 00034 while (pixIndex < pixelLen) { 00035 sendPixel(pixArray + pixIndex++); 00036 } 00037 else 00038 while (pixIndex < pixelLen) { 00039 sendPixel(pixArray16 + pixIndex++); 00040 } 00041 00042 } 00043 00044 // each bytes sent as two 12 bit messages (3 bits of data per LED bit). 00045 void wsDrive::sendByte(unsigned char value) 00046 { 00047 00048 uint16_t dataToSend = 0; 00049 00050 uint8_t mask = 0x80; 00051 while (mask) { 00052 dataToSend += (value & mask)?0x06:0x4; // 100 for a 0 or 110 for a 1 00053 if (mask & 0x11) { // trans 00054 fastWrite(dataToSend); 00055 dataToSend = 0; 00056 } 00057 dataToSend = dataToSend << 3; 00058 mask = mask >> 1; 00059 } 00060 } 00061 00062 void wsDrive::sendPixel(pixelInfo *pixToSend) 00063 { 00064 sendByte(pixToSend->G); 00065 sendByte(pixToSend->R); 00066 sendByte(pixToSend->B); 00067 } 00068 00069 void wsDrive::sendPixel(pixelInfo16 *pixToSend) 00070 { 00071 sendByte(pixToSend->G<255?(unsigned char)pixToSend->G:255); 00072 sendByte(pixToSend->R<255?(unsigned char)pixToSend->R:255); 00073 sendByte(pixToSend->B<255?(unsigned char)pixToSend->B:255); 00074 } 00075 00076
Generated on Fri Jul 15 2022 00:11:46 by
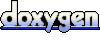