
Now with added rickrolling. A program to mess with people. Intended for small boards with built in USB sockets that connect direct to the CPU and can easily be hidden behind a computer (e.g. the mBuino).
main.cpp
00001 #include "mbed.h" 00002 #include "USBMouseKeyboard.h" 00003 #include "searchText.h" 00004 00005 00006 /* 00007 Magic key combos 00008 00009 r + logo - open run box 00010 m + logo - minimise all 00011 m + logo + shift - maximise all 00012 right arrow + logo + shift - move current window right one monitor 00013 l + logo - lock screen 00014 */ 00015 00016 #define KEY_ENTER 0x0a 00017 00018 // turn off all LEDs (some boards they glow a bit if you don't). 00019 // you may need to remove some depending on the board. 00020 //BusOut LEDs(LED1, LED2, LED3, LED4, LED5, LED6, LED7); 00021 int LEDs; 00022 USBMouseKeyboard keyboard; 00023 00024 00025 /// Run image searches at interval seconds for given number of repeats or -1 to run forever 00026 void startSearches(float interval, int number); 00027 00028 void runCommand(char *address); 00029 00030 // enter a keyboard string 00031 void sendString(const char *str); 00032 00033 // draw a mouse circle 00034 void drawCircle(float radius, float time, bool dir=true); 00035 00036 // draw a mouse line 00037 void drawLine(float length, float time, bool horizontal=true, bool direction=true); 00038 00039 // Turn caps lock on n seconds after they turn it off. 00040 void forceCaps(float delay, float checkPeriod=0.5); 00041 00042 void maxVolume(); 00043 void capsOn(); 00044 00045 main() 00046 { 00047 LEDs = 0; 00048 wait(10); 00049 while (true) { 00050 capsOn(); 00051 while ((keyboard.lockStatus() & 0x02) == 0x02) { 00052 wait(1); 00053 } 00054 maxVolume(); 00055 runCommand("https://www.youtube.com/watch?v=dQw4w9WgXcQ"); 00056 drawCircle(80,15); 00057 drawCircle(100,15); 00058 drawCircle(120,15); 00059 wait(60*5); 00060 } 00061 } 00062 00063 int searchCount; 00064 int searchStop; 00065 Ticker searchTicker; 00066 00067 void doNextSearch() 00068 { 00069 static int searchIndex = 0; 00070 keyboard.keyCode('r',KEY_LOGO); // windows key an R 00071 wait(0.1); // delay to give the run box a chance to come up. 00072 sendString(searchPrefix); 00073 sendString( searches[searchIndex++] ); 00074 sendString(searchSuffix); 00075 wait(0.05); // delay to give the run box a chance to come up. 00076 keyboard.putc(0x0a); // hit enter 00077 if (searchIndex == searchStringCount) 00078 searchIndex = 0; 00079 00080 searchCount++; 00081 if (searchCount == searchStop) 00082 searchTicker.attach(NULL,0); 00083 } 00084 00085 void startSearches(float interval, int number) 00086 { 00087 searchCount = 0; 00088 searchStop = number; 00089 searchTicker.attach(doNextSearch,interval); 00090 } 00091 00092 void runCommand(char *address) 00093 { 00094 keyboard.keyCode('r',KEY_LOGO); // windows key an R 00095 wait(0.1); // delay to give the run box a chance to come up. 00096 sendString(address); 00097 wait(0.05); 00098 keyboard.putc(0x0a); // hit enter 00099 } 00100 00101 void sendString(const char *str) 00102 { 00103 while (*str != 0) { 00104 keyboard.putc(*str); 00105 str++; 00106 } 00107 } 00108 00109 void maxVolume() 00110 { 00111 for (int i = 0; i<50; i++) { 00112 keyboard.mediaControl(KEY_VOLUME_UP); 00113 } 00114 } 00115 00116 void drawCircle(float radius, float time, bool dir) 00117 { 00118 00119 const float timeStep = 0.05; 00120 float currentx = 0; 00121 float currenty = radius; 00122 int steps = time/timeStep; 00123 float angleDelta = 2*3.14159265359 / steps; 00124 float xPos,yPos; 00125 00126 if (!dir) 00127 angleDelta = -angleDelta; 00128 00129 00130 for (int i = 0; i<steps; i++) { 00131 xPos = radius*sin(angleDelta*i); 00132 yPos = radius*cos(angleDelta*i); 00133 int xDelta = ((xPos - currentx) > 0)?(xPos - currentx) + 0.5 : (xPos - currentx) - 0.5 ; 00134 int yDelta = ((yPos - currenty) > 0)?(yPos - currenty) + 0.5 : (yPos - currenty) - 0.5 ; 00135 keyboard.move(xDelta,yDelta); 00136 currentx += xDelta; 00137 currenty += yDelta; 00138 wait(timeStep); 00139 } 00140 } 00141 00142 void drawLine(float length, float time, bool horizontal, bool direction) 00143 { 00144 00145 const float timeStep = 0.05; 00146 float currentPos = 0; 00147 int steps = time/timeStep; 00148 float stepSize = length / steps; 00149 float movement; 00150 00151 if (!direction) 00152 stepSize = -stepSize; 00153 00154 for (int i = 0; i<steps; i++) { 00155 00156 movement = stepSize*i - currentPos; 00157 int moveDelta; 00158 if (movement>0) 00159 moveDelta = movement+0.5; 00160 else 00161 moveDelta = movement-0.5; 00162 00163 if (horizontal) 00164 keyboard.move(moveDelta,0); 00165 else 00166 keyboard.move(0,moveDelta); 00167 00168 currentPos += moveDelta; 00169 wait(timeStep); 00170 } 00171 } 00172 00173 Ticker capLockTick; 00174 Timeout capLockTimer; 00175 bool capsTimerActive = false; 00176 float capsWaitPeriod = 60; 00177 00178 void capsOn(void) 00179 { 00180 capsTimerActive = false; 00181 if ((keyboard.lockStatus() & 0x02) == 0) 00182 keyboard.keyCode(KEY_CAPS_LOCK); 00183 } 00184 00185 void checkCapsLock(void) 00186 { 00187 LEDs = keyboard.lockStatus(); 00188 if ((keyboard.lockStatus() & 0x02) == 0) { 00189 if (!capsTimerActive) { 00190 capLockTimer.attach(capsOn,capsWaitPeriod); 00191 capsTimerActive = true; 00192 } 00193 } else { 00194 if (capsTimerActive) { 00195 capLockTimer.detach(); 00196 capsTimerActive = false; 00197 } 00198 } 00199 } 00200 00201 void forceCaps(float delay, float checkPeriod) 00202 { 00203 if (checkPeriod == 0) 00204 capLockTick.attach(NULL,0); 00205 else { 00206 capsWaitPeriod = delay; 00207 capLockTick.attach(checkCapsLock,checkPeriod); 00208 } 00209 }
Generated on Wed Jul 13 2022 19:17:59 by
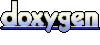