DW1000 UWB driver based on work of Matthias Grob & Manuel Stalder - ETH Zürich - 2015
DW1000 Class Reference
#include <DW1000.h>
Public Member Functions | |
DW1000 (PinName MOSI, PinName MISO, PinName SCLK, PinName CS, PinName IRQ) | |
Constructor. | |
uint32_t | getDeviceID () |
Read the device ID. | |
uint64_t | getEUI () |
Read the Extended Unique ID. | |
void | setEUI (uint64_t EUI) |
Set the Extended Unique ID. | |
float | getVoltage () |
Read voltage input. | |
float | getTemperature () |
Read on board temperature sensor. | |
uint64_t | getStatus () |
Get the status register. | |
void | setRxDelay (uint16_t ticks) |
Set receive antenna delay. | |
void | setTxDelay (uint16_t ticks) |
Set transmit antenna delay. | |
void | setRxDelayDistance (double errorDistance) |
Set receive antenna delay in meters. | |
void | setTxDelayDistance (double errorDistance) |
Set transmit antenna delay in meters. | |
uint32_t | readOTP (uint16_t word_address) |
Read a value from the OTP memory. | |
bool | writeOTP (uint16_t word_address, uint32_t data) |
Write a value to the OTP memory. | |
DW1000Setup * | getSetup () |
get the current radio configuration | |
uint32_t | getTxPower (float *power=NULL, float *boost500=NULL, float *boost250=NULL, float *boost125=NULL) |
Get the current Transmit gain settings. | |
void | setTxPower (float normalPowerdB, float boost500=0, float boost250=0, float boost125=0) |
Set Transmit gain. | |
void | getRxSignalPower (float *direct, float *total) |
Get the rx signal power for the last packet. | |
uint8_t | readXTALTune () |
Read the current crystal tuning value. | |
void | setXTALTune (uint8_t value) |
Set the crystal tuning value. | |
void | enterRFTestMode () |
Puts the device into CW test mode. | |
bool | applySetup (DW1000Setup *setup) |
apply a new radio setup to the UWB system | |
Protected Member Functions | |
void | setCallbacks (void(*callbackRX)(void), void(*callbackTX)(void)) |
Sets the callbacks on packet Rx and Tx. | |
template<typename T > | |
void | setCallbacks (T *tptr, void(T::*mptrRX)(void), void(T::*mptrTX)(void)) |
c++ version of setCallbacks() | |
uint64_t | getRXTimestamp () |
Get the last packet recieve time. | |
uint64_t | getTXTimestamp () |
Get the last packet transmit time. | |
void | sendFrame (uint8_t *message, uint16_t length) |
Send a packet. | |
void | sendDelayedFrame (uint8_t *message, uint16_t length, uint64_t TxTimestamp) |
Send a packet at a certain time. | |
void | setupSyncedFrame (uint8_t *message, uint16_t length) |
Set up data for a transmit on sync. | |
void | armSyncedFrame () |
Transmit on the next sync pulse. | |
uint16_t | getFramelength () |
Get last packet size. | |
void | readRxBuffer (uint8_t *buffer, int length) |
Get last recieved packet. | |
void | startRX () |
Enable reciever. | |
void | stopTRX () |
Disable radio link. | |
void | resetRX () |
Reset the reciever logic. | |
void | setInterrupt (bool RX, bool TX) |
Enable/Disable interrupts. | |
void | getFirstPath (uint16_t *fp_amp2, uint16_t *fp_amp3) |
Get the first path amplitude values. | |
uint16_t | getLDE_THRESH () |
Get the LDE threshold value. | |
uint16_t | getLDE_PPAMPL () |
Get the LDE Peak path amplitude. |
Detailed Description
A DW1000 driver.
It is expected that the protocol implimentation above this will inherit this object. If not using this structure then move the protected functions to being public.
Definition at line 25 of file DW1000.h.
Constructor & Destructor Documentation
DW1000 | ( | PinName | MOSI, |
PinName | MISO, | ||
PinName | SCLK, | ||
PinName | CS, | ||
PinName | IRQ | ||
) |
Constructor.
The radio will default to DW1000Setup::tunedDefault until you call applySetup() with a new configuration.
Definition at line 5 of file DW1000.cpp.
Member Function Documentation
bool applySetup | ( | DW1000Setup * | setup ) |
apply a new radio setup to the UWB system
- Parameters:
-
setup The new settings to use
- Returns:
- true if the setup was applied.
The setup object supplied is copied and can be disposed of after the call. If the supplied setup fails DW1000Setup::check() then it is ignored and the function returns false.
- Note:
- This will reset the radio. You must re-enable interupts, receiver etc. after calling it.
Definition at line 55 of file DW1000.cpp.
void armSyncedFrame | ( | ) | [protected] |
Transmit on the next sync pulse.
On the next rising edge of the sync line the transmitter will be activated. The packet must have previously been set up using setupSyncedFrame()
Rx is disabled until transmission is complete and then re-enabled.
Definition at line 834 of file DW1000.cpp.
void enterRFTestMode | ( | ) |
Puts the device into CW test mode.
The radio will be set to output a constant wave at carrier frequency. Transmit gain will be set to the maximum of 33.5dB but can be chaged by setting the TX power register To exit test mode the radio should be reset.
Definition at line 28 of file DW1000.cpp.
uint32_t getDeviceID | ( | ) |
uint64_t getEUI | ( | ) |
Read the Extended Unique ID.
- Returns:
- The device EUI as stored in the system registers
Definition at line 743 of file DW1000.cpp.
void getFirstPath | ( | uint16_t * | fp_amp2, |
uint16_t * | fp_amp3 | ||
) | [protected] |
Get the first path amplitude values.
- Parameters:
-
fp_amp2 Will be set to first path second peak amplitude fp_amp3 Will be set to first path third peak amplitude
Reads the two registers for the last packet recieved. Used for quality metrics.
Definition at line 704 of file DW1000.cpp.
uint16_t getFramelength | ( | ) | [protected] |
Get last packet size.
- Returns:
- The length in bytes of the last packet received
Definition at line 990 of file DW1000.cpp.
uint16_t getLDE_PPAMPL | ( | ) | [protected] |
uint16_t getLDE_THRESH | ( | ) | [protected] |
void getRxSignalPower | ( | float * | direct, |
float * | total | ||
) |
Get the rx signal power for the last packet.
- Parameters:
-
direct Is set to the direct path Rx power in dBm total Is set to the total Rx power in dBm
According to the DW1000 manual if the direct path power is within 6dB of the total then it was probably a LoS measurment. If there is more than 10dB difference then it's probably an indirect path.
Definition at line 660 of file DW1000.cpp.
uint64_t getRXTimestamp | ( | ) | [protected] |
Get the last packet recieve time.
- Returns:
- the internal time stamp for the last packet Rx
Time is counted on a clock running at 499.2MHz * 128 (~15.65ps) This value is raw time minus user set Rx antenna delay.
Definition at line 790 of file DW1000.cpp.
DW1000Setup * getSetup | ( | ) |
get the current radio configuration
- Returns:
- A pointer to a DW1000Setup object of the current setup.
Note to change the setup you must make a copy of the current setup and then pass that to applySetup().
Definition at line 50 of file DW1000.cpp.
uint64_t getStatus | ( | ) |
Get the status register.
- Returns:
- The system status register
See user manual section 7.2.17 for details
Definition at line 785 of file DW1000.cpp.
float getTemperature | ( | ) |
Read on board temperature sensor.
- Returns:
- The temperature in C
For accurate ranging the temperature of the device should be taken into account. User manual give variation as ~2.15mm / C
Definition at line 770 of file DW1000.cpp.
uint32_t getTxPower | ( | float * | power = NULL , |
float * | boost500 = NULL , |
||
float * | boost250 = NULL , |
||
float * | boost125 = NULL |
||
) |
Get the current Transmit gain settings.
- Parameters:
-
power Optional, is set to the first power in dBm boost500 Optional, is set to the second power in dBm boost250 Optional, is set to the third power in dBm boost125 Optional, is set to the forth power in dBm
- Returns:
- The raw transmit gain register value
If smart power is on then power represents the normal transmit power, boost500-boost125 indicates the power used for packets of that number of us or less.
If smart power is off then boost500 represents the gain for the PHY header, boost250 the gain for the main message. power and boost125 are not used.
Definition at line 471 of file DW1000.cpp.
uint64_t getTXTimestamp | ( | ) | [protected] |
Get the last packet transmit time.
- Returns:
- the internal time stamp for the last packet Tx
Time is counted on a clock running at 499.2MHz * 128 (~15.65ps) This value is raw time plus user set Tx antenna delay to give time at the antenna.
Definition at line 795 of file DW1000.cpp.
float getVoltage | ( | ) |
Read voltage input.
- Returns:
- the current device voltage
For accurate ranging the voltage of the device should be taken into account. User manual give variation as ~5.35cm / V
Definition at line 756 of file DW1000.cpp.
uint32_t readOTP | ( | uint16_t | word_address ) |
Read a value from the OTP memory.
- Parameters:
-
word_address The OTP memory address to read.
- Returns:
- The 32 bit value at that address.
See Section 6.3.1 of the user manual for the memory map.
Definition at line 960 of file DW1000.cpp.
void readRxBuffer | ( | uint8_t * | buffer, |
int | length | ||
) | [protected] |
uint8_t readXTALTune | ( | ) |
Read the current crystal tuning value.
Reads the current FX_XTALT value from 0 to 0x1f. See section 8.1 of the manual for details.
- Returns:
- The current value.
Definition at line 373 of file DW1000.cpp.
void resetRX | ( | ) | [protected] |
Reset the reciever logic.
This should be done after any receive errors
Definition at line 892 of file DW1000.cpp.
void sendDelayedFrame | ( | uint8_t * | message, |
uint16_t | length, | ||
uint64_t | TxTimestamp | ||
) | [protected] |
Send a packet at a certain time.
- Parameters:
-
message A buffer containing the data to send length The length of the data in bytes. TxTimestamp The timestamp to send the packet.
The supplied packet is transmitted once the internal clock reaches the specified timestamp. Maximum packet size is 125 bytes. Rx is disabled as soon as this command is issued and re-enabled once transmission is complete. Note - 9 LSBs are ignored so timings are only accurate to ~8ns. For more accurate timing check the tx timestamp after transmission is complete.
The receiver is re-activated as soon as packet transmission is complete.
Definition at line 840 of file DW1000.cpp.
void sendFrame | ( | uint8_t * | message, |
uint16_t | length | ||
) | [protected] |
Send a packet.
- Parameters:
-
message A buffer containing the data to send length The length of the data in bytes.
The supplied packet is transmitted as soon as possible and the reciever re-enabled once transmission is complete. Maximum packet size is 125 bytes.
The receiver is re-activated as soon as packet transmission is complete.
Definition at line 801 of file DW1000.cpp.
void setCallbacks | ( | void(*)(void) | callbackRX, |
void(*)(void) | callbackTX | ||
) | [protected] |
Sets the callbacks on packet Rx and Tx.
- Parameters:
-
callbackRX The function to call on packet Rx complete callbackTX The function to call on packet Tx complete
set either or both to null to disable the appropriate interupt
Definition at line 721 of file DW1000.cpp.
void setCallbacks | ( | T * | tptr, |
void(T::*)(void) | mptrRX, | ||
void(T::*)(void) | mptrTX | ||
) | [protected] |
c++ version of setCallbacks()
- Parameters:
-
tptr object for callbacks mptrRX method to call on packet Rx complete mptrTX method to call on packet Tx complete
void setEUI | ( | uint64_t | EUI ) |
Set the Extended Unique ID.
- Parameters:
-
EUI The EUID to use
- Note:
- ID is only valid until the next power cycle and overrides the value in the OTP memory. To set a value that is automatically loaded on startup set OTP memory addresses 0 and 1.
Definition at line 750 of file DW1000.cpp.
void setInterrupt | ( | bool | RX, |
bool | TX | ||
) | [protected] |
Enable/Disable interrupts.
- Parameters:
-
RX true to enable recieve interrupts TX true to enable transmit interrupts
For c style callbacks simply set the callback to null to disable it. When using c++ style callbacks both are enabled as default, this allows a method to disabled one or both.
Definition at line 970 of file DW1000.cpp.
void setRxDelay | ( | uint16_t | ticks ) |
Set receive antenna delay.
- Parameters:
-
ticks Delay in system clock cycles
Definition at line 712 of file DW1000.cpp.
void setRxDelayDistance | ( | double | errorDistance ) |
void setTxDelay | ( | uint16_t | ticks ) |
Set transmit antenna delay.
- Parameters:
-
ticks Delay in system clock cycles
Definition at line 716 of file DW1000.cpp.
void setTxDelayDistance | ( | double | errorDistance ) |
void setTxPower | ( | float | normalPowerdB, |
float | boost500 = 0 , |
||
float | boost250 = 0 , |
||
float | boost125 = 0 |
||
) |
Set Transmit gain.
- Parameters:
-
normalPowercB Normal transmit gain to use. boost500 Gain to use for 6.8Mb/s packets of under 500ms. boost250 Gain to use for 6.8Mb/s packets of under 250ms. boost125 Gain to use for 6.8Mb/s packets of under 125ms.
All gains are in dB. Gains can be between 0 and 33.5dB. Boost gains are optional, if not specified boost gains are set to the power for the lower rate (e.g. boost125 is set to the boost250 level). If smart power is disabled then the normal gain is used for all settings. The values in the internal DW1000Setup are updated to reflect the configured powers.
Definition at line 435 of file DW1000.cpp.
void setupSyncedFrame | ( | uint8_t * | message, |
uint16_t | length | ||
) | [protected] |
Set up data for a transmit on sync.
- Parameters:
-
message A buffer containing the data to send length The length of the data in bytes.
Data is loaded into the transmit buffer but the transmission is not started. Maximum packet size is 125 bytes.
Definition at line 822 of file DW1000.cpp.
void setXTALTune | ( | uint8_t | value ) |
Set the crystal tuning value.
Sets the value of the FX_XTALT register. See section 8.1 of the manual for details.
Values will be lost on reset, consider programming the final value into the OTP memory to store perminently.
The value to use from 0 to 0x1f
Definition at line 378 of file DW1000.cpp.
void startRX | ( | ) | [protected] |
Enable reciever.
This is automatically done after each Tx completes but can also be forced manually
Definition at line 857 of file DW1000.cpp.
void stopTRX | ( | ) | [protected] |
Disable radio link.
Disables both the recieve and transmit systems. Any transmissions waiting for a delayed time or sync pulse will be canceled.
Definition at line 862 of file DW1000.cpp.
bool writeOTP | ( | uint16_t | word_address, |
uint32_t | data | ||
) |
Write a value to the OTP memory.
After writes have been completed reset the device.
- Parameters:
-
word_address The OTP memory address to read. data The value to write
- Returns:
- True if the write was sucessful.
Writes the supplied data to the OTP memory and then reads it back to verify it was sucessfully programmed.
- Warning:
- This is a one time operation for each memory address. See Section 6.3.1 of the user manual for the memory map.
- Note:
- It is recommened that the device is reset or power cycled after programing.
Definition at line 913 of file DW1000.cpp.
Generated on Mon Jul 18 2022 07:51:56 by
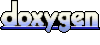