This is my quadcopter prototype software, still in development!
Embed:
(wiki syntax)
Show/hide line numbers
ITG3200.cpp
00001 /** 00002 * @author Aaron Berk 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2010 ARM Limited 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * 00028 * ITG-3200 triple axis, digital interface, gyroscope. 00029 * 00030 * Datasheet: 00031 * 00032 * http://invensense.com/mems/gyro/documents/PS-ITG-3200-00-01.4.pdf 00033 */ 00034 00035 /** 00036 * Includes 00037 */ 00038 00039 00040 #include "ITG3200.h" 00041 00042 ITG3200::ITG3200(PinName sda, PinName scl) : i2c_(sda, scl) { 00043 00044 //400kHz, fast mode. 00045 i2c_.frequency(400000); 00046 //Set FS_SEL to 0x03 for proper operation. 00047 //See datasheet for details. 00048 char tx[2]; 00049 tx[0] = DLPF_FS_REG; 00050 //FS_SEL bits sit in bits 4 and 3 of DLPF_FS register. 00051 tx[1] = 0x03 << 3; 00052 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00053 } 00054 char ITG3200::getWhoAmI(void){ 00055 //WhoAmI Register address. 00056 char tx = WHO_AM_I_REG; 00057 char rx; 00058 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00059 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00060 return rx; 00061 } 00062 void ITG3200::setWhoAmI(char address){ 00063 char tx[2]; 00064 tx[0] = WHO_AM_I_REG; 00065 tx[1] = address; 00066 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00067 } 00068 char ITG3200::getSampleRateDivider(void){ 00069 char tx = SMPLRT_DIV_REG; 00070 char rx; 00071 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00072 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00073 return rx; 00074 } 00075 void ITG3200::setSampleRateDivider(char divider){ 00076 char tx[2]; 00077 tx[0] = SMPLRT_DIV_REG; 00078 tx[1] = divider; 00079 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00080 } 00081 int ITG3200::getInternalSampleRate(void){ 00082 char tx = DLPF_FS_REG; 00083 char rx; 00084 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00085 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00086 //DLPF_CFG == 0 -> sample rate = 8kHz. 00087 if(rx == 0){ 00088 return 8; 00089 } 00090 //DLPF_CFG = 1..7 -> sample rate = 1kHz. 00091 else if(rx >= 1 && rx <= 7){ 00092 return 1; 00093 } 00094 //DLPF_CFG = anything else -> something's wrong! 00095 else{ 00096 return -1; 00097 } 00098 } 00099 void ITG3200::setLpBandwidth(char bandwidth){ 00100 char tx[2]; 00101 tx[0] = DLPF_FS_REG; 00102 //Bits 4,3 are required to be 0x03 for proper operation. 00103 tx[1] = bandwidth | (0x03 << 3); 00104 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00105 } 00106 char ITG3200::getInterruptConfiguration(void){ 00107 char tx = INT_CFG_REG; 00108 char rx; 00109 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00110 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00111 return rx; 00112 } 00113 void ITG3200::setInterruptConfiguration(char config){ 00114 char tx[2]; 00115 tx[0] = INT_CFG_REG; 00116 tx[1] = config; 00117 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00118 } 00119 bool ITG3200::isPllReady(void){ 00120 char tx = INT_STATUS; 00121 char rx; 00122 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00123 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00124 //ITG_RDY bit is bit 4 of INT_STATUS register. 00125 if(rx & 0x04){ 00126 return true; 00127 } 00128 else{ 00129 return false; 00130 } 00131 } 00132 bool ITG3200::isRawDataReady(void){ 00133 char tx = INT_STATUS; 00134 char rx; 00135 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00136 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00137 //RAW_DATA_RDY bit is bit 1 of INT_STATUS register. 00138 if(rx & 0x01){ 00139 return true; 00140 } 00141 else{ 00142 return false; 00143 } 00144 } 00145 float ITG3200::getTemperature(void){ 00146 char tx = TEMP_OUT_H_REG; 00147 char rx[2]; 00148 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00149 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00150 int16_t temperature = ((int) rx[0] << 8) | ((int) rx[1]); 00151 //Offset = -35 degrees, 13200 counts. 280 counts/degrees C. 00152 return 35.0 + ((temperature + 13200)/280.0); 00153 } 00154 int ITG3200::getGyroX(void){ 00155 char tx = GYRO_XOUT_H_REG; 00156 char rx[2]; 00157 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00158 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00159 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00160 return output; 00161 } 00162 int ITG3200::getGyroY(void){ 00163 char tx = GYRO_YOUT_H_REG; 00164 char rx[2]; 00165 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00166 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00167 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00168 return output; 00169 } 00170 int ITG3200::getGyroZ(void){ 00171 char tx = GYRO_ZOUT_H_REG; 00172 char rx[2]; 00173 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00174 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, rx, 2); 00175 int16_t output = ((int) rx[0] << 8) | ((int) rx[1]); 00176 return output; 00177 } 00178 char ITG3200::getPowerManagement(void){ 00179 char tx = PWR_MGM_REG; 00180 char rx; 00181 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, &tx, 1); 00182 i2c_.read((ITG3200_I2C_ADDRESS << 1) | 0x01, &rx, 1); 00183 return rx; 00184 } 00185 void ITG3200::setPowerManagement(char config){ 00186 char tx[2]; 00187 tx[0] = PWR_MGM_REG; 00188 tx[1] = config; 00189 i2c_.write((ITG3200_I2C_ADDRESS << 1) & 0xFE, tx, 2); 00190 } 00191
Generated on Wed Jul 13 2022 23:20:56 by
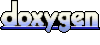