Provides an API software interface to TIMER2 to control upto four stepper motors.
Embed:
(wiki syntax)
Show/hide line numbers
SimpleStepperOutput.h
00001 /**************************************************************************** 00002 * Product: LIB17 Open Source Library 00003 * 00004 * Steller Technologies Limited 00005 * ---------------------------- 00006 * 00007 * Copyright (C) 2002-2011 Steller Technologies Limited. All rights reserved. 00008 * 00009 * This software may be distributed and modified under the terms of the GNU 00010 * General Public License version 2 (GPL) as published by the Free Software 00011 * Foundation and appearing in the file GPL.TXT included in the packaging of 00012 * this file. Please note that GPL Section 2[b] requires that all works based 00013 * on this software must also be made publicly available under the terms of 00014 * the GPL ("Copyleft"). 00015 * 00016 * Alternatively, this software may be distributed and modified under the 00017 * terms of Steller Technologies Limited commercial licenses, which expressly 00018 * supersede the GPL and are specifically designed for licensees interested in 00019 * retaining the proprietary status of their code. 00020 * 00021 * $Id:$ 00022 * 00023 ***************************************************************************/ 00024 00025 /** 00026 * @defgroup SimpleStepperOutput SimpleStepperOutput functions 00027 */ 00028 00029 #ifndef AJK_SIMPLESTEPPEROUTPUT_H 00030 #define AJK_SIMPLESTEPPEROUTPUT_H 00031 00032 #ifndef __ARMCC_VERSION 00033 #include "SimpleStepperMbed.h" 00034 #else 00035 #include "mbed.h" 00036 #endif 00037 00038 00039 namespace AjK { 00040 00041 /** SimpleStepperOutput - Adds pin output objects. 00042 * 00043 * The Mbed library supplies the DigitalIn and DigitalOut objects to allow you 00044 * to specify ins and outs. 00045 * 00046 * SimpleStepperOutput allows library objects to implement pins without the requirement 00047 * to link against the Mbed library. This increase portability when using 00048 * alternate compilers (such as the Code Red GCC C++ compiler for LPCXpresso). 00049 * 00050 * @ingroup SimpleStepperOutput 00051 */ 00052 class SimpleStepperOutput { 00053 public: 00054 enum Direction { 00055 Out = 0 00056 , In 00057 }; 00058 00059 protected: 00060 PinName pin; 00061 uint32_t mask; 00062 uint32_t fiodir; 00063 uint32_t fiomask; 00064 uint32_t fiopin; 00065 uint32_t fioset; 00066 uint32_t fioclr; 00067 00068 inline void setpin(PinName p) { pin = p; } 00069 inline void setmask(PinName p) { mask = (uint32_t)(1UL << ((uint32_t)p & 0x1F)); } 00070 inline void setDir(PinName p) { fiodir = (uint32_t)(p & ~0x1F) + 0x00; } 00071 inline void setMask(PinName p) { fiomask = (uint32_t)(p & ~0x1F) + 0x10; } 00072 inline void setPin(PinName p) { fiopin = (uint32_t)(p & ~0x1F) + 0x14; } 00073 inline void setSet(PinName p) { fioset = (uint32_t)(p & ~0x1F) + 0x18; } 00074 inline void setClr(PinName p) { fioclr = (uint32_t)(p & ~0x1F) + 0x1C; } 00075 00076 inline void pinUp() { *((volatile uint32_t *)fioset) = mask; } 00077 inline void pinDn() { *((volatile uint32_t *)fioclr) = mask; } 00078 inline uint32_t pinIs() { return *((volatile uint32_t *)(fiopin)) & mask; } 00079 00080 public: 00081 00082 /** Constructor 00083 * @ingroup SimpleStepperOutput 00084 */ 00085 SimpleStepperOutput(PinName p, Direction d = Out, PinMode m = PullDown) { init(p, d, m); }; 00086 00087 /** write 00088 * 00089 * Writes a value to the pin. 00090 * 00091 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00092 * @ingroup SimpleStepperOutput 00093 * @param i Zero makes the pin 0v, non-zero makes the pin 1. 00094 */ 00095 void write(int i) { if (i!=0) { pinUp(); } else { pinDn(); } } 00096 00097 /** read 00098 * 00099 * Reads the value on the pin. 00100 * 00101 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00102 * @ingroup SimpleStepperOutput 00103 * @return int 0v returns zero, otherwise returns 1. 00104 */ 00105 int read(void) { return pinIs() ? 1 : 0; }; 00106 00107 /** output 00108 * 00109 * Setup the pin to be an output. 00110 * 00111 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00112 * @ingroup SimpleStepperOutput 00113 * @ingroup API 00114 * @return int 0v returns zero, otherwise returns 1. 00115 */ 00116 void output(void) { *((volatile uint32_t *)fiodir) |= mask; } 00117 00118 /** input 00119 * 00120 * Setup the pin to be an input. 00121 * 00122 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00123 * @ingroup SimpleStepperOutput 00124 * @return int 0v returns zero, otherwise returns 1. 00125 */ 00126 void input(void) { *((volatile uint32_t *)fiodir) &= ~mask; } 00127 00128 /** getPin 00129 * 00130 * Get the PinName this object is operating on. 00131 * 00132 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00133 * @ingroup SimpleStepperOutput 00134 * @return int 0v returns zero, otherwise returns 1. 00135 */ 00136 PinName getPin(void) { return pin; } 00137 00138 /** getDirection 00139 * 00140 * Get the operational direction this pin is setup for. 00141 * 00142 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00143 * @ingroup SimpleStepperOutput 00144 * @return int 0v returns zero, otherwise returns 1. 00145 */ 00146 int getDirection(void) { return *((volatile uint32_t *)fiomask) & mask ? 1 : 0; } 00147 00148 /** operator int() 00149 * 00150 * Reads the value on the pin. 00151 * 00152 * @see read 00153 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00154 * @ingroup SimpleStepperOutput 00155 * @return int 0v returns zero, otherwise returns 1. 00156 */ 00157 operator int() { return read(); } 00158 00159 /** operator= 00160 * 00161 * Writes a value to the pin. 00162 * 00163 * @see write 00164 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00165 * @ingroup SimpleStepperOutput 00166 */ 00167 SimpleStepperOutput& operator= (int value) { write(value); return *this; } 00168 00169 /** operator= 00170 * 00171 * Writes a value to the pin. 00172 * 00173 * @see write 00174 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00175 * @ingroup SimpleStepperOutput 00176 */ 00177 SimpleStepperOutput& operator= (SimpleStepperOutput& rhs) { write(rhs.read()); return *this; } 00178 00179 /** getMask 00180 * 00181 * Get the mask value for this pin. 00182 * 00183 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00184 * @ingroup SimpleStepperOutput 00185 * @return uint32_t The mask value used by this pin. 00186 */ 00187 uint32_t getMask(void) { return mask; } 00188 00189 /** getFiodir 00190 * 00191 * Get the FIODIR register for the port the pin is on. 00192 * 00193 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00194 * @ingroup SimpleStepperOutput 00195 * @return uint32_t The register value. 00196 */ 00197 uint32_t getFiodir(void) { return fiodir; } 00198 00199 /** getFiomask 00200 * 00201 * Get the FIOMASK register for the port the pin is on. 00202 * 00203 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00204 * @ingroup SimpleStepperOutput 00205 * @return uint32_t The register value. 00206 */ 00207 uint32_t getFiomask(void) { return fiomask; } 00208 00209 /** getFiopin 00210 * 00211 * Get the FIOPIN register for the port the pin is on. 00212 * 00213 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00214 * @ingroup SimpleStepperOutput 00215 * @return uint32_t The register value. 00216 */ 00217 uint32_t getFiopin(void) { return fiopin; } 00218 00219 /** getFioset 00220 * 00221 * Get the FIOSET register for the port the pin is on. 00222 * 00223 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00224 * @ingroup SimpleStepperOutput 00225 * @return uint32_t The register value. 00226 */ 00227 uint32_t getFioset(void) { return fioset; } 00228 00229 /** getFioclr 00230 * 00231 * Get the FIOCLR register for the port the pin is on. 00232 * 00233 * @see http://cornflakes.wikidot.com/lib17:core:lib17-dio 00234 * @ingroup SimpleStepperOutput 00235 * @return uint32_t The register value. 00236 */ 00237 uint32_t getFioclr(void) { return fioclr; } 00238 00239 00240 protected: 00241 void init(PinName p, Direction d, PinMode m) 00242 { 00243 // We rely on the fact that by default the LPC1768 00244 // sets all pins to be GPIO. The user will change 00245 // that if they need to. So we don't bother trying 00246 // to setup PINSELx 00247 00248 // psel(); // Not used, see above. 00249 00250 setpin(p); 00251 setmask(p); 00252 setDir(p); 00253 setMask(p); 00254 setPin(p); 00255 setSet(p); 00256 setClr(p); 00257 00258 if (d == Out) output(); 00259 else mode( m ); 00260 } 00261 00262 protected: 00263 void psel(void) 00264 { 00265 uint32_t ppsel, pumask; 00266 00267 if (pin >= P0_0 && pin <= P0_15) ppsel = (uint32_t)(&LPC_PINCON->PINSEL0); 00268 else if (pin >= P0_16 && pin <= P0_31) ppsel = (uint32_t)(&LPC_PINCON->PINSEL1); 00269 else if (pin >= P1_0 && pin <= P1_15) ppsel = (uint32_t)(&LPC_PINCON->PINSEL2); 00270 else if (pin >= P1_16 && pin <= P1_31) ppsel = (uint32_t)(&LPC_PINCON->PINSEL3); 00271 else if (pin >= P2_0 && pin <= P2_15) ppsel = (uint32_t)(&LPC_PINCON->PINSEL4); 00272 else if (pin >= P3_16 && pin <= P3_31) ppsel = (uint32_t)(&LPC_PINCON->PINSEL7); 00273 else if (pin >= P4_16 && pin <= P4_31) ppsel = (uint32_t)(&LPC_PINCON->PINSEL9); 00274 else return; 00275 00276 pumask = ~(3UL << ((pin & 0x1F)>>1)); 00277 *((volatile uint32_t *)ppsel) &= pumask; 00278 } 00279 00280 public: 00281 void mode(PinMode m) 00282 { 00283 uint32_t ppmod, pumask; 00284 00285 if (m == OpenDrain) { 00286 openDrain(1); 00287 } 00288 else { 00289 if (pin >= P0_0 && pin <= P0_15) { 00290 ppmod = (uint32_t)(&LPC_PINCON->PINMODE0); 00291 pumask = ((m & 0x3) << ( ((pin & 0x1F)-0)*2) ); 00292 } 00293 else if (pin >= P0_16 && pin <= P0_31) { 00294 ppmod = (uint32_t)(&LPC_PINCON->PINMODE1); 00295 pumask = ((m & 0x3) << ( ((pin & 0x1F)-16)*2) ); 00296 } 00297 else if (pin >= P1_0 && pin <= P1_15) { 00298 ppmod = (uint32_t)(&LPC_PINCON->PINMODE2); 00299 pumask = ((m & 0x3) << ( ((pin & 0x1F)-0)*2) ); 00300 } 00301 else if (pin >= P1_16 && pin <= P1_31) { 00302 ppmod = (uint32_t)(&LPC_PINCON->PINMODE3); 00303 pumask = ((m & 0x3) << ( ((pin & 0x1F)-16)*2) ); 00304 } 00305 else if (pin >= P2_0 && pin <= P2_15) { 00306 ppmod = (uint32_t)(&LPC_PINCON->PINMODE4); 00307 pumask = ((m & 0x3) << ( ((pin & 0x1F)-0)*2) ); 00308 } 00309 else if (pin >= P3_16 && pin <= P3_31) { 00310 ppmod = (uint32_t)(&LPC_PINCON->PINMODE7); 00311 pumask = ((m & 0x3) << ( ((pin & 0x1F)-16)*2) ); 00312 } 00313 else if (pin >= P4_16 && pin <= P4_31) { 00314 ppmod = (uint32_t)(&LPC_PINCON->PINMODE9); 00315 pumask = ((m & 0x3) << ( ((pin & 0x1F)-16)*2) ); 00316 } 00317 else return; 00318 00319 *((volatile uint32_t *)ppmod) |= pumask; 00320 } 00321 } 00322 00323 public: 00324 void openDrain(int i = 1) 00325 { 00326 if (pin >= P0_0 && pin <= P0_31) { if (i) LPC_PINCON->PINMODE_OD0 |= mask; else LPC_PINCON->PINMODE_OD0 &= ~mask; } 00327 else if (pin >= P1_0 && pin <= P1_31) { if (i) LPC_PINCON->PINMODE_OD1 |= mask; else LPC_PINCON->PINMODE_OD1 &= ~mask; } 00328 else if (pin >= P2_0 && pin <= P2_31) { if (i) LPC_PINCON->PINMODE_OD2 |= mask; else LPC_PINCON->PINMODE_OD2 &= ~mask; } 00329 else if (pin >= P3_16 && pin <= P3_31) { if (i) LPC_PINCON->PINMODE_OD3 |= mask; else LPC_PINCON->PINMODE_OD3 &= ~mask; } 00330 else if (pin >= P4_16 && pin <= P4_31) { if (i) LPC_PINCON->PINMODE_OD4 |= mask; else LPC_PINCON->PINMODE_OD4 &= ~mask; } 00331 } 00332 00333 }; 00334 00335 }; /* namespace AjK ends. */ 00336 00337 using namespace AjK; 00338 00339 #endif /* AJK_SIMPLESTEPPEROUTPUT_H */
Generated on Thu Jul 14 2022 17:06:02 by
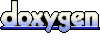