Very simple cooperative round-robin task scheduler. See examples.
Embed:
(wiki syntax)
Show/hide line numbers
STcallback.h
00001 /* 00002 Copyright (c) 2011 Andy Kirkham 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef AJK_STCALLBACK_H 00024 #define AJK_STCALLBACK_H 00025 00026 namespace AjK { 00027 00028 class SimpleTask; 00029 class STcallbackDummy; 00030 00031 /** STcallback - Adds callbacks that take SimpleTask * pointer data type. 00032 * 00033 * The Mbed library supplies a callback using the FunctionPointer object as 00034 * defined in FunctionPointer.h However, this callback system does not allow 00035 * the caller to pass a value to the callback. Likewise, the callback itself 00036 * cannot return a value. 00037 * 00038 * Note, when passing pointers to variables to the callback, if the callback 00039 * function/method changes that variable's value then it will also change the 00040 * value the caller sees. If C pointers are new to you, you are strongly 00041 * advised to read up on the subject. It's pointers that often get beginners 00042 * into trouble when mis-used. 00043 * 00044 * @see http://mbed.org/handbook/C-Data-Types 00045 * @see http://mbed.org/projects/libraries/svn/mbed/trunk/FunctionPointer.h 00046 * @see http://mbed.org/cookbook/FunctionPointer 00047 * @see http://mbed.org/cookbook/FPointer 00048 */ 00049 class STcallback { 00050 00051 protected: 00052 00053 //! C callback function pointer. 00054 void (*c_callback)(SimpleTask *); 00055 00056 //! C++ callback object/method pointer (the object part). 00057 STcallbackDummy *obj_callback; 00058 00059 //! C++ callback object/method pointer (the method part). 00060 void (STcallbackDummy::*method_callback)(SimpleTask *); 00061 00062 public: 00063 00064 /** Constructor 00065 */ 00066 STcallback() { 00067 c_callback = NULL; 00068 obj_callback = NULL; 00069 method_callback = NULL; 00070 } 00071 00072 /** attach - Overloaded attachment function. 00073 * 00074 * Attach a C type function pointer as the callback. 00075 * 00076 * Note, the callback function prototype must be:- 00077 * @code 00078 * void myCallbackFunction(SimpleTask *p); 00079 * @endcode 00080 * @param A C function pointer to call. 00081 */ 00082 void attach(void (*function)(SimpleTask *) = 0) { c_callback = function; } 00083 00084 /** attach - Overloaded attachment function. 00085 * 00086 * Attach a C++ type object/method pointer as the callback. 00087 * 00088 * Note, the callback method prototype must be:- 00089 * @code 00090 * public: 00091 * void myCallbackFunction(SimpleTask *p); 00092 * @endcode 00093 * @param A C++ object pointer. 00094 * @param A C++ method within the object to call. 00095 */ 00096 template<class T> 00097 void attach(T* item = 0, void (T::*method)(SimpleTask *) = 0) { 00098 obj_callback = (STcallbackDummy *)item; 00099 method_callback = (void (STcallbackDummy::*)(SimpleTask *))method; 00100 } 00101 00102 /** call - Overloaded callback initiator. 00103 * 00104 * call the callback function. 00105 * 00106 * @param SimpleTask * pointer. 00107 */ 00108 void call(SimpleTask *arg) { 00109 if (c_callback != 0) { 00110 (*c_callback)(arg); 00111 } 00112 else { 00113 if (obj_callback != 0 && method_callback != 0) { 00114 (obj_callback->*method_callback)(arg); 00115 } 00116 } 00117 } 00118 }; 00119 00120 }; // namespace AjK ends 00121 00122 using namespace AjK; 00123 00124 #endif
Generated on Fri Jul 15 2022 08:21:53 by
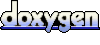