
Web server based weather station using Sparkfun Weather Meters.
Dependencies: FatFileSystem mbed WeatherMeters SDFileSystem
IHTTPRequestHandlerContext Class Reference
Interface to be returned from an application to provide callbacks for a specific HTTP request. More...
#include <HTTPServer.h>
Inherited by CFileRequestHandlerContext, CHTTPContext [protected]
, and CPrintfGetRequestHandlerContext.
Public Member Functions | |
virtual int | BeginRequestHeaders ()=0 |
Called by the CHTTPServer object before any WriteRequestHeader() calls to let the application to know to expect subsequent WriteRequestHeader() calls. | |
virtual void | WriteRequestHeader (const char *pHeaderName, const char *pHeaderValue)=0 |
Called by CHTTPServer for each HTTP request header sent by the HTTP client. | |
virtual void | EndRequestHeaders ()=0 |
Called by the CHTTPServer object after it has completed all of the necessary WriteRequestHeader() calls to enumerate the headers of the HTTP client's request. | |
virtual int | BeginRequestContent (size_t ContentSize)=0 |
Called by CHTTPServer to let the application know that it should expect subsequent WriteRequestContentBlock() calls for processing of the request content as it arrives on the TCP/IP socket. | |
virtual void | WriteRequestContentBlock (const void *pBlockBuffer, size_t BlockBufferSize)=0 |
Called for each block of request data received from the HTTP client. | |
virtual void | EndRequestContent ()=0 |
Called by CHTTPServer after it has completed all of the WriteRequestContentBlock() calls for processing of the request content data sent from the HTTP client. | |
virtual const char * | GetStatusLine (size_t *pStatusLineLength)=0 |
Called by CHTTPServer object to get the status line from the application to be returned to the HTTP client for this request. | |
virtual const char * | GetResponseHeaders (size_t *pResponseHeaderLength)=0 |
Called by the CHTTPServer object to get all of the response headers that the application would like to be returned to the HTTP client. | |
virtual int | BeginResponseContent ()=0 |
Called by CHTTPServer to let application know that there will be subsequent ReadResponseContentBlock() calls to obtain the content data from the application to be sent back to the HTTP client in the response. | |
virtual size_t | ReadResponseContentBlock (char *pBuffer, size_t BytesToRead)=0 |
Called by CHTTPServer to get the next block of response data to be sent back to the HTTP client. | |
virtual void | EndResponseContent ()=0 |
Called by CHTTPServer to let the application know that it has finished sending all of the response data to the HTTP client. | |
virtual void | Release ()=0 |
Called by the CHTTPServer object when it is completely done processing this HTTP response request so that the application can free up any resources it has associated with this HTTP client request, including the IHTTPRequestHandlerContext derived object servicing these callbacks. |
Detailed Description
Interface to be returned from an application to provide callbacks for a specific HTTP request.
Definition at line 47 of file HTTPServer.h.
Member Function Documentation
virtual int BeginRequestContent | ( | size_t | ContentSize ) | [pure virtual] |
Called by CHTTPServer to let the application know that it should expect subsequent WriteRequestContentBlock() calls for processing of the request content as it arrives on the TCP/IP socket.
Only POST requests from a client should include such content data.
- Parameters:
-
ContentSize is the size of the request content to be sent from the HTTP client. This value indicates the total number of bytes that should result from all of the WriteRequestContentBlock() calls to follow.
- Returns:
- 0 if the application isn't interested in receiving subsequent WriteRequestContentBlock() and EndRequestContent() callbacks. Returns non-zero value to receive request content data.
virtual int BeginRequestHeaders | ( | ) | [pure virtual] |
Called by the CHTTPServer object before any WriteRequestHeader() calls to let the application to know to expect subsequent WriteRequestHeader() calls.
- Returns:
- 0 if the application isn't interested in receiving subsequent WriteRequestHeader() and EndRequestHeaders() callbacks. Returns non-zero value to receive headers.
virtual int BeginResponseContent | ( | ) | [pure virtual] |
Called by CHTTPServer to let application know that there will be subsequent ReadResponseContentBlock() calls to obtain the content data from the application to be sent back to the HTTP client in the response.
- Returns:
- 0 if the application has no content to be sent back in the HTTP response.
virtual void EndRequestContent | ( | ) | [pure virtual] |
Called by CHTTPServer after it has completed all of the WriteRequestContentBlock() calls for processing of the request content data sent from the HTTP client.
virtual void EndRequestHeaders | ( | ) | [pure virtual] |
Called by the CHTTPServer object after it has completed all of the necessary WriteRequestHeader() calls to enumerate the headers of the HTTP client's request.
virtual void EndResponseContent | ( | ) | [pure virtual] |
Called by CHTTPServer to let the application know that it has finished sending all of the response data to the HTTP client.
The application can use such a call to close any files that it has open for satsifying this request.
virtual const char* GetResponseHeaders | ( | size_t * | pResponseHeaderLength ) | [pure virtual] |
Called by the CHTTPServer object to get all of the response headers that the application would like to be returned to the HTTP client.
The CHTTPServer will append this header string to the "Server: ServerName\r\n" header that it always sends.
Example: "Content-Length: 100\r\n"
- Parameters:
-
pResponseHeaderLength points to the length to be filled in by the application indicating the length, in character, of the returned header string. It should not include the NULL terminator and can be 0 if the returned value is NULL.
- Returns:
- a pointer to the extra headers to be returned to the HTTP client for this request. It can be NULL if the application has no special headers to be returned.
virtual const char* GetStatusLine | ( | size_t * | pStatusLineLength ) | [pure virtual] |
Called by CHTTPServer object to get the status line from the application to be returned to the HTTP client for this request.
Examples: "HTTP/1.0 200 OK\r\n" -or- "HTTP/1.0 404 File no found\r\n"
- Parameters:
-
pStatusLineLength points to the length to be filled in by the application indicating the length, in character, of the returned status line. It should not include the NULL terminator. It must have a non-zero value.
- Returns:
- a pointer to the status line to be returned to the HTTP client for this request. It should start with the "HTTP/1.0" string and end with a newline designator of "\r\n". The return value can't be NULL.
virtual size_t ReadResponseContentBlock | ( | char * | pBuffer, |
size_t | BytesToRead | ||
) | [pure virtual] |
Called by CHTTPServer to get the next block of response data to be sent back to the HTTP client.
- Parameters:
-
pBuffer is a pointer to the buffer to be filled in by the application with data to be sent back to the HTTP client in the response. BytesToRead indicates the number of bytes that should be placed into pBuffer by the application. The application should only place fewer than this requested amount on the last block of data to be sent back to the HTTP client.
- Returns:
- The number of bytes that were actually placed in pBuffer for this call. It can only be smaller than BytesToRead on the last block to be sent since the server uses such truncated reads to know when it has sent the last block of response data.
virtual void Release | ( | ) | [pure virtual] |
Called by the CHTTPServer object when it is completely done processing this HTTP response request so that the application can free up any resources it has associated with this HTTP client request, including the IHTTPRequestHandlerContext derived object servicing these callbacks.
virtual void WriteRequestContentBlock | ( | const void * | pBlockBuffer, |
size_t | BlockBufferSize | ||
) | [pure virtual] |
Called for each block of request data received from the HTTP client.
The server won't issue this callback if the application returned 0 from the BeginRequestContent() method.
- Parameters:
-
pBlockBuffer is a pointer to the block of request content data most recently received from the HTTP client. BlockBufferSize is the number of bytes contained in pBlockBuffer.
virtual void WriteRequestHeader | ( | const char * | pHeaderName, |
const char * | pHeaderValue | ||
) | [pure virtual] |
Called by CHTTPServer for each HTTP request header sent by the HTTP client.
The server won't issue this callback if 0 was previously returned by the application from the BeginRequestHeaders() method.
- Parameters:
-
pHeaderName is the name of this header field. If this string is empty then this header line is a continuation of the previous header line which did have a field name. The string pointed to by this parameter has a short lifetime and is only valid during the duration of this call. pHeaderValue is the string value of this header field. The string pointed to by this parameter has a short lifetime and is only valid during the duration of this call.
Generated on Tue Jul 12 2022 20:48:16 by
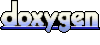