
Use a Sparkfun Blackberry Trackball Breakout Board as a mouse.
Dependencies: mbed BBTrackball
main.cpp
00001 /* Copyright 2011 Adam Green (http://mbed.org/users/AdamGreen/) 00002 00003 Licensed under the Apache License, Version 2.0 (the "License"); 00004 you may not use this file except in compliance with the License. 00005 You may obtain a copy of the License at 00006 00007 http://www.apache.org/licenses/LICENSE-2.0 00008 00009 Unless required by applicable law or agreed to in writing, software 00010 distributed under the License is distributed on an "AS IS" BASIS, 00011 WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00012 See the License for the specific language governing permissions and 00013 limitations under the License. 00014 */ 00015 /* Interfaces to a Sparkfun Blackberry Trackball Breakout Board and uses mbed's 00016 USBMouse class to make it look like a mouse to a PC. Uses the trackball 00017 motion to move the mouse pointer, pushing down on the trackball will trigger 00018 a microswitch which will act as a left button click, and the LEDs under the 00019 trackball will be lit different colours depending on what the user is 00020 currently doing with the device (red for no motion, green when the trackball 00021 is being moved, and blue during button clicks). It does use some other 00022 utility classes from acceleration.h to provide acceleration to the trackball 00023 motion. 00024 */ 00025 #include <mbed.h> 00026 #include <USBMouse.h> 00027 #include "acceleration.h" 00028 00029 00030 00031 int main() 00032 { 00033 static USBMouse Mouse; 00034 static CAcceleratedTrackball Trackball(p20, // BLU 00035 p25, // RED 00036 p26, // GRN 00037 p10, // WHT 00038 p5, // UP 00039 p6, // DWN 00040 p7, // LFT 00041 p8, // RHT 00042 p9); // BTN 00043 00044 for(;;) 00045 { 00046 int DeltaX; 00047 int DeltaY; 00048 int ButtonPressed; 00049 00050 Trackball.GetState(DeltaX, DeltaY, ButtonPressed); 00051 00052 Mouse.update(DeltaX, 00053 DeltaY, 00054 ButtonPressed ? MOUSE_LEFT : 0, 00055 0); 00056 } 00057 }
Generated on Fri Jul 15 2022 14:34:39 by
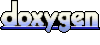