
This is a sample example code to get started using ESP8266NodeMCU library. Please make necessary changes.
Dependencies: ESP8266NodeMCUInterface mbed
main.cpp
00001 #include "mbed.h" 00002 #include "ESP8266.h" 00003 #include "TCPSocketConnection.h" 00004 #include <string> 00005 using namespace std; 00006 00007 DigitalOut myled(LED1); 00008 ESP8266 ark(PA_9,PA_10,D3,115200); 00009 Serial pc(SERIAL_TX, SERIAL_RX); 00010 TCPSocketConnection gm; 00011 00012 int main() 00013 { 00014 pc.baud(115200); 00015 int i=0; 00016 while(1) { 00017 if(i==0) { 00018 hardwareInit: 00019 pc.printf("intializing hardware,...\r\n"); 00020 if(ark.init()) { 00021 pc.printf("hardware intialized!\r\n"); 00022 i=1; 00023 nustConnection: 00024 pc.printf("connecting to NUST,...\r\n"); 00025 if(ark.connect("NUST","nust008tech")) { 00026 00027 pc.printf("NUST Connected!\r\n"); 00028 if(ark.is_connected()) { 00029 pc.printf("connection confirmed!\r\n"); 00030 pc.printf("IP Adress: %s\r\n",ark.getIPAddress()); 00031 hostConnection: 00032 pc.printf("connecting to Host,...\r\n"); 00033 if(gm.connect("207.58.139.247",80)==0) { 00034 pc.printf("Host connected.\r\n"); 00035 00036 char getRequest[] = "GET /testwifi/index.html HTTP/1.1\r\nHost: www.adafruit.com\r\nConnection: keep-alive\r\nAccept: */*\r\n\r\n"; 00037 sendGETRequest: 00038 pc.printf("sending GET request,...\r\n"); 00039 if(gm.send_all(getRequest,strlen(getRequest))>0) { 00040 pc.printf("GET requested!\r\n"); 00041 00042 char getResponce[500]={}; 00043 pc.printf("reading responce of GET request!\r\n"); 00044 int resBytes = gm.receive_all(getResponce,500); 00045 if(resBytes > 0) { 00046 pc.printf("Responce Received!\r\n"); 00047 pc.printf("Responce:\r\n%s\r\n",getResponce); 00048 } else if(resBytes==0) { 00049 pc.printf("Empty Responce!\r\n"); 00050 goto sendGETRequest; 00051 } else { 00052 pc.printf("Resopnce NOT Received!\r\n"); 00053 goto sendGETRequest; 00054 } 00055 00056 } else { 00057 pc.printf("GET request failed!\r\n"); 00058 goto sendGETRequest; 00059 } 00060 } else { 00061 pc.printf("Host Connection Failed\r\n"); 00062 goto hostConnection; 00063 } 00064 } else { 00065 pc.printf("sonething went wrong with connection. not connected \r\n"); 00066 goto nustConnection; 00067 } 00068 } else { 00069 pc.printf("conenction fail!\r\n"); 00070 goto nustConnection; 00071 } 00072 } else { 00073 pc.printf("hardware not intialized!\r\n"); 00074 goto hardwareInit; 00075 } 00076 } 00077 wait(1); 00078 } 00079 }
Generated on Wed Jul 13 2022 16:02:05 by
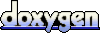