
Demo of 4DGL library for the uLCD-144-G2 128 by 128 color display. See https://mbed.org/users/4180_1/notebook/ulcd-144-g2-128-by-128-color-lcd/ for instructions
Dependencies: 4DGL-uLCD-SE mbed
Fork of uVGAII_demo by
main.cpp
00001 // uLCD-144-G2 demo program for uLCD-4GL LCD driver library 00002 // 00003 #include "mbed.h" 00004 #include "uLCD_4DGL.h" 00005 00006 uLCD_4DGL uLCD(p9,p10,p11); // serial tx, serial rx, reset pin; 00007 00008 int main() 00009 { 00010 // basic printf demo = 16 by 18 characters on screen 00011 uLCD.printf("\nHello uLCD World\n"); //Default Green on black text 00012 uLCD.printf("\n Starting Demo..."); 00013 uLCD.text_width(4); //4X size text 00014 uLCD.text_height(4); 00015 uLCD.color(RED); 00016 for (int i=10; i>=0; --i) { 00017 uLCD.locate(1,2); 00018 uLCD.printf("%2D",i); 00019 wait(.5); 00020 } 00021 uLCD.cls(); 00022 uLCD.printf("Change baudrate......"); 00023 uLCD.baudrate(3000000); //jack up baud rate to max for fast display 00024 //if demo hangs here - try lower baud rates 00025 // 00026 // printf text only full screen mode demo 00027 uLCD.background_color(BLUE); 00028 uLCD.cls(); 00029 uLCD.locate(0,0); 00030 uLCD.color(WHITE); 00031 uLCD.textbackground_color(BLUE); 00032 uLCD.set_font(FONT_7X8); 00033 uLCD.text_mode(OPAQUE); 00034 int i=0; 00035 while(i<64) { 00036 if(i%16==0) uLCD.cls(); 00037 uLCD.printf("TxtLine %2D Page %D\n",i%16,i/16 ); 00038 i++; //16 lines with 18 charaters per line 00039 } 00040 wait(0.5); 00041 //demo graphics commands 00042 uLCD.background_color(BLACK); 00043 uLCD.cls(); 00044 uLCD.background_color(DGREY); 00045 uLCD.filled_circle(60, 50, 30, 0xFF00FF); 00046 uLCD.triangle(120, 100, 40, 40, 10, 100, 0x0000FF); 00047 uLCD.line(0, 0, 80, 60, 0xFF0000); 00048 uLCD.filled_rectangle(50, 50, 100, 90, 0x00FF00); 00049 uLCD.pixel(60, 60, BLACK); 00050 uLCD.read_pixel(120, 70); 00051 uLCD.circle(120, 60, 10, BLACK); 00052 uLCD.set_font(FONT_7X8); 00053 uLCD.text_mode(TRANSPARENT); 00054 uLCD.text_bold(ON); 00055 uLCD.text_char('B', 9, 8, BLACK); 00056 uLCD.text_char('I',10, 8, BLACK); 00057 uLCD.text_char('G',11, 8, BLACK); 00058 uLCD.text_italic(ON); 00059 uLCD.text_string("This is a test of string", 1, 4, FONT_7X8, WHITE); 00060 wait(2); 00061 00062 //Bouncing Ball Demo 00063 float fx=50.0,fy=21.0,vx=1.0,vy=0.4; 00064 int x=50,y=21,radius=4; 00065 uLCD.background_color(BLACK); 00066 uLCD.cls(); 00067 //draw walls 00068 uLCD.line(0, 0, 127, 0, WHITE); 00069 uLCD.line(127, 0, 127, 127, WHITE); 00070 uLCD.line(127, 127, 0, 127, WHITE); 00071 uLCD.line(0, 127, 0, 0, WHITE); 00072 for (int i=0; i<1500; i++) { 00073 //draw ball 00074 uLCD.filled_circle(x, y, radius, RED); 00075 //bounce off edge walls and slow down a bit? 00076 if ((x<=radius+1) || (x>=126-radius)) vx = -.90*vx; 00077 if ((y<=radius+1) || (y>=126-radius)) vy = -.90*vy; 00078 //erase old ball location 00079 uLCD.filled_circle(x, y, radius, BLACK); 00080 //move ball 00081 fx=fx+vx; 00082 fy=fy+vy; 00083 x=(int)fx; 00084 y=(int)fy; 00085 } 00086 wait(0.5); 00087 //draw an image pixel by pixel 00088 int pixelcolors[50][50]; 00089 uLCD.background_color(BLACK); 00090 uLCD.cls(); 00091 //compute Mandelbrot set image for display 00092 //image size in pixels 00093 const unsigned ImageHeight=128; 00094 const unsigned ImageWidth=128; 00095 //"c" region to display 00096 double MinRe = -0.75104; 00097 double MaxRe = -0.7408; 00098 double MinIm = 0.10511; 00099 double MaxIm = MinIm+(MaxRe-MinRe)*ImageHeight/ImageWidth; 00100 double Re_factor = (MaxRe-MinRe)/(ImageWidth-1); 00101 double Im_factor = (MaxIm-MinIm)/(ImageHeight-1); 00102 unsigned MaxIterations = 2048; 00103 for(unsigned y=0; y<ImageHeight; ++y) { 00104 double c_im = MaxIm - y*Im_factor; 00105 for(unsigned x=0; x<ImageWidth; ++x) { 00106 double c_re = MinRe + x*Re_factor; 00107 double Z_re = c_re, Z_im = c_im; 00108 int niterations=0; 00109 for(unsigned n=0; n<MaxIterations; ++n) { 00110 double Z_re2 = Z_re*Z_re, Z_im2 = Z_im*Z_im; 00111 if(Z_re2 + Z_im2 > 4) { 00112 niterations = n; 00113 break; 00114 } 00115 Z_im = 2*Z_re*Z_im + c_im; 00116 Z_re = Z_re2 - Z_im2 + c_re; 00117 } 00118 if (niterations!=(MaxIterations-1)) 00119 uLCD.pixel(x,y,((niterations & 0xF00)<<12)+((niterations & 0xF0)<<8)+((niterations & 0x0F)<<4) ); 00120 } 00121 } 00122 wait(5); 00123 // PLASMA wave BLIT animation 00124 //draw an image using BLIT (Block Image Transfer) fastest way to transfer pixel data 00125 uLCD.cls(); 00126 int num_cols=50; 00127 int num_rows=50; 00128 int frame=0; 00129 double a,b,c=0.0; 00130 while(frame<75) { 00131 for (int k=0; k<num_cols; k++) { 00132 b= (1+sin(3.14159*k*0.75/(num_cols-1.0)+c))*0.5; 00133 for (int i=0; i<num_rows; i++) { 00134 a= (1+sin(3.14159*i*0.75/(num_rows-1.0)+c))*0.5; 00135 // a and b will be a sine wave output between 0 and 1 00136 // sine wave was scaled for nice effect across array 00137 // uses a and b to compute pixel colors based on rol and col location in array 00138 // also keeps colors at the same brightness level 00139 if ((a+b) <.667) 00140 pixelcolors[i][k] = (255-(int(254.0*((a+b)/0.667)))<<16) | (int(254.0*((a+b)/0.667))<<8) | 0; 00141 else if ((a+b)<1.333) 00142 pixelcolors[i][k] = (0 <<16) | (255-(int (254.0*((a+b-0.667)/0.667)))<<8) | int(254.0*((a+b-0.667)/0.667)); 00143 else 00144 pixelcolors[i][k] = (int(255*((a+b-1.333)/0.667))<<16) | (0<<8) | (255-(int (254.0*((a+b-1.333)/0.667)))); 00145 } 00146 } 00147 uLCD.BLIT(39, 39, 50, 50, &pixelcolors[0][0]); 00148 c = c + 0.0314159*3.0; 00149 if (c > 6.2831) c = 0.0; 00150 frame++; 00151 } 00152 //Load Image Demo 00153 uLCD.cls(); 00154 //SD card needed with image and video files for last two demos 00155 uLCD.cls(); 00156 uLCD.media_init(); 00157 uLCD.printf("\n\nAn SD card is needed for image and video data"); 00158 uLCD.set_sector_address(0x001D, 0x4C01); 00159 uLCD.display_image(0,0); 00160 wait(10); 00161 //Play video demo 00162 while(1) { 00163 uLCD.cls(); 00164 uLCD.media_init(); 00165 uLCD.set_sector_address(0x001D, 0x4C42); 00166 uLCD.display_video(0,0); 00167 } 00168 } 00169 00170 00171
Generated on Tue Jul 12 2022 23:20:38 by
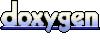