
For ESP8266 Wi Fi SOC from Sparkfun on the mbed LPC1768. Sets up a web page server that can control a few mbed pins. Nice IoT demo. Need to configure Wi Fi SSID and PASSWORD first with another program first (one time only). See https://developer.mbed.org/users/4180_1/notebook/using-the-esp8266-with-the-mbed-lpc1768/ for more info.
Fork of ESP8266-WEB-Mbed-Controller by
main.cpp
00001 // ESP8266 Static page WEB server to control Mbed 00002 00003 #include "mbed.h" 00004 //#include "DS18B20.h" 00005 00006 Serial pc(USBTX, USBRX); 00007 Serial esp(p28, p27); // tx, rx 00008 00009 //DS18B20 thermom(A0, DS18B20::RES_12_BIT); 00010 00011 // Standard Mbed LED definitions 00012 DigitalOut led1(LED1); // (PTB18) 00013 DigitalOut led2(LED2); // (PTB19) 00014 DigitalOut led3(LED3); // (PTD1) 00015 00016 // Digital Out and In pins, can be configured to any suitable pin depending on Platform 00017 DigitalOut Out1(p6); 00018 DigitalOut Out2(p7); 00019 DigitalOut Out3(p8); 00020 DigitalOut reset(p26); 00021 00022 DigitalIn In1(p9); 00023 DigitalIn In2(p10); 00024 DigitalIn In3(p11); 00025 00026 PwmOut speaker(p21); 00027 AnalogIn Ain1(p18); 00028 AnalogIn Ain2(p19); 00029 00030 Timer t1; 00031 Timer t2; 00032 00033 struct tm t; 00034 00035 int bufflen, DataRX, count, getcount, replycount, servreq, timeout; 00036 int bufl, ipdLen, linkID, weberror, webcounter; 00037 float temperature, AdcIn, Ht; 00038 float R1=100000, R2=10000; // resistor values to give a 10:1 reduction of measured AnalogIn voltage 00039 char Vcc[10]; 00040 char Temp[10]; 00041 char temp[10]; 00042 char webcount[8]; 00043 char lasthit[30]; 00044 char timebuf[30]; 00045 char type[16]; 00046 char type1[16]; 00047 char channel[2]; 00048 char cmdbuff[32]; 00049 char replybuff[1024]; 00050 char webdata[1024]; // This may need to be bigger depending on WEB browser used 00051 char webbuff[4096]; // Currently using 1986 characters, Increase this if more web page data added 00052 00053 void SendCMD(),getreply(),ReadWebData(),startserver(),sendpage(),SendWEB(),sendcheck(); 00054 void gettime(),gettemp(),getbattery(),setRTC(),beep(); 00055 00056 // manual set RTC values 00057 int minute =00; // 0-59 00058 int hour =12; // 2-23 00059 int dayofmonth =26; // 1-31 00060 int month =8; // 1-12 00061 int year =15; // last 2 digits 00062 00063 int port =80; // set server port 00064 int SERVtimeout =5; // set server timeout in seconds in case link breaks. 00065 00066 // Serial Interrupt read ESP data 00067 void callback() 00068 { 00069 led3=1; 00070 while (esp.readable()) { 00071 webbuff[count] = esp.getc(); 00072 count++; 00073 } 00074 if(strlen(webbuff)>bufflen) { 00075 DataRX=1; 00076 led3=0; 00077 } 00078 } 00079 00080 int main() 00081 { 00082 reset=0; 00083 pc.baud(115200); 00084 00085 pc.printf("\f\n\r------------ ESP8266 Hardware Reset --------------\n\r"); 00086 wait(0.5); 00087 reset=1; 00088 led1=1,led2=0,led3=0; 00089 timeout=6000; 00090 getcount=500; 00091 getreply(); 00092 esp.baud(115200); // ESP8266 baudrate. Maximum on KLxx' is 115200, 230400 works on K20 and K22F 00093 if (time(NULL) < 1420070400) { 00094 setRTC(); 00095 } 00096 beep(); 00097 startserver(); 00098 00099 while(1) { 00100 if(DataRX==1) { 00101 ReadWebData(); 00102 beep(); 00103 if (servreq == 1 && weberror == 0) { 00104 sendpage(); 00105 } 00106 esp.attach(&callback); 00107 pc.printf(" IPD Data:\r\n\n Link ID = %d,\r\n IPD Header Length = %d \r\n IPD Type = %s\r\n", linkID, ipdLen, type); 00108 pc.printf("\n\n HTTP Packet: \n\n%s\n", webdata); 00109 pc.printf(" Web Characters sent : %d\n\n", bufl); 00110 pc.printf(" -------------------------------------\n\n"); 00111 strcpy(lasthit, timebuf); 00112 servreq=0; 00113 } 00114 } 00115 } 00116 // Static WEB page 00117 void sendpage() 00118 { 00119 gettemp(); 00120 getbattery(); 00121 gettime(); 00122 00123 // WEB page data 00124 strcpy(webbuff, "<!DOCTYPE html>"); 00125 strcat(webbuff, "<html><head><title>ESP8266 Mbed LPC1768</title></head>"); 00126 strcat(webbuff, "<body>"); 00127 strcat(webbuff, "<div style=\"text-align:center; background-color:#F4F4F4; color:#00AEDB;\"><h1>ESP8266 Mbed IoT Web Controller</h1>"); 00128 strcat(webbuff, "Hit Count - "); 00129 strcat(webbuff, webcount); 00130 strcat(webbuff, "<br>Last Hit - "); 00131 strcat(webbuff, lasthit); 00132 strcat(webbuff, "</div><br /><hr>"); 00133 strcat(webbuff, "<h3>Mbed RTC Time -  "); 00134 strcat(webbuff, timebuf); 00135 strcat(webbuff, "</h3>\r\n"); 00136 strcat(webbuff, "<p><form method=\"POST\"><strong> Analog 1:  <input type=\"text\" size=6 value=\""); 00137 strcat(webbuff, Temp); 00138 strcat(webbuff, "\"> </sup>V <form method=\"POST\"> <strong>   Analog 2:  <input type=\"text\" size=4 value=\""); 00139 strcat(webbuff, Vcc); 00140 strcat(webbuff, "\"> </sup>V"); 00141 if(led1==0) { 00142 strcat(webbuff, "<p><input type=\"radio\" name=\"led1\" value=\"0\" checked> LED 1 off"); 00143 strcat(webbuff, "<br><input type=\"radio\" name=\"led1\" value=\"1\" > LED 1 on"); 00144 } else { 00145 strcat(webbuff, "<p><input type=\"radio\" name=\"led1\" value=\"0\" > LED 1 off"); 00146 strcat(webbuff, "<br><input type=\"radio\" name=\"led1\" value=\"1\" checked> LED 1 on"); 00147 } 00148 if(Out1==0) { 00149 strcat(webbuff, "<p><input type=\"radio\" name=\"Out1\" value=\"0\" checked> Digital Out 1 off"); 00150 strcat(webbuff, "<br><input type=\"radio\" name=\"Out1\" value=\"1\" > Digital Out 1 on"); 00151 } else { 00152 strcat(webbuff, "<p><input type=\"radio\" name=\"Out1\" value=\"0\" > Digital Out 1 off"); 00153 strcat(webbuff, "<br><input type=\"radio\" name=\"Out1\" value=\"1\" checked> Digital Out 1 on"); 00154 } 00155 if(Out2==0) { 00156 strcat(webbuff, "<p><input type=\"radio\" name=\"Out2\" value=\"0\" checked> Digital Out 2 off"); 00157 strcat(webbuff, "<br><input type=\"radio\" name=\"Out2\" value=\"1\" > Digital Out 2 on"); 00158 } else { 00159 strcat(webbuff, "<p><input type=\"radio\" name=\"Out2\" value=\"0\" > Digital Out 2 off"); 00160 strcat(webbuff, "<br><input type=\"radio\" name=\"Out2\" value=\"1\" checked> Digital Out 2 on"); 00161 } 00162 if(Out3==0) { 00163 strcat(webbuff, "<p><input type=\"radio\" name=\"Out3\" value=\"0\" checked> Digital Out 3 off"); 00164 strcat(webbuff, "<br><input type=\"radio\" name=\"Out3\" value=\"1\" > Digital Out 3 on"); 00165 } else { 00166 strcat(webbuff, "<p><input type=\"radio\" name=\"Out3\" value=\"0\" > Digital Out 3 off"); 00167 strcat(webbuff, "<br><input type=\"radio\" name=\"Out3\" value=\"1\" checked> Digital Out 3 on"); 00168 } 00169 if(In1==0) { 00170 strcat(webbuff, "<p><input type=\"radio\" name=\"In1\" value=\"0\" > Digital In 1"); 00171 } else { 00172 strcat(webbuff, "<p><input type=\"radio\" name=\"In1\" value=\"1\" checked> Digital In 1"); 00173 } 00174 if(In2==0) { 00175 strcat(webbuff, "<br><input type=\"radio\" name=\"In2\" value=\"0\" > Digital In 2"); 00176 } else { 00177 strcat(webbuff, "<br><input type=\"radio\" name=\"In2\" value=\"1\" checked> Digital In 2"); 00178 } 00179 if(In3==0) { 00180 strcat(webbuff, "<br><input type=\"radio\" name=\"In3\" value=\"0\" > Digital In 3"); 00181 } else { 00182 strcat(webbuff, "<br><input type=\"radio\" name=\"In3\" value=\"1\" checked> Digital In 3"); 00183 } 00184 strcat(webbuff, "</strong><p><input type=\"submit\" value=\"send-refresh\" style=\"background: #3498db;"); 00185 strcat(webbuff, "background-image:-webkit-linear-gradient(top, #3498db, #2980b9);"); 00186 strcat(webbuff, "background-image:linear-gradient(to bottom, #3498db, #2980b9);"); 00187 strcat(webbuff, "-webkit-border-radius:12;border-radius: 12px;font-family: Arial;color:#ffffff;font-size:20px;padding:"); 00188 strcat(webbuff, "10px 20px 10px 20px; border:solid #103c57 3px;text-decoration: none;"); 00189 strcat(webbuff, "background: #3cb0fd;"); 00190 strcat(webbuff, "background-image:-webkit-linear-gradient(top,#3cb0fd,#1a5f8a);"); 00191 strcat(webbuff, "background-image:linear-gradient(to bottom,#3cb0fd,#1a5f8a);"); 00192 strcat(webbuff, "text-decoration:none;\"></form></span>"); 00193 strcat(webbuff, "<p/><h2>How to use:</h2><ul>"); 00194 strcat(webbuff, "<li>Select the Radio buttons to control the digital out pins.</li>"); 00195 strcat(webbuff, "<li>Click 'Send-Refresh' to send.</li>"); 00196 strcat(webbuff, "<li>Use the 'Send-Refresh' button to refresh the data.</li>"); 00197 strcat(webbuff, "</ul>"); 00198 strcat(webbuff, "</body></html>"); 00199 // end of WEB page data 00200 bufl = strlen(webbuff); // get total page buffer length 00201 sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00202 timeout=200; 00203 getcount=7; 00204 SendCMD(); 00205 getreply(); 00206 SendWEB(); // send web page 00207 memset(webbuff, '\0', sizeof(webbuff)); 00208 sendcheck(); 00209 } 00210 00211 // wait for ESP "SEND OK" reply, then close IP to load web page 00212 void sendcheck() 00213 { 00214 weberror=1; 00215 timeout=500; 00216 getcount=24; 00217 t2.reset(); 00218 t2.start(); 00219 while(weberror==1 && t2.read() <5) { 00220 getreply(); 00221 if (strstr(replybuff, "SEND OK") != NULL) { 00222 weberror=0; // wait for valid SEND OK 00223 } 00224 } 00225 if(weberror==1) { // restart connection 00226 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); 00227 timeout=500; 00228 getcount=10; 00229 SendCMD(); 00230 getreply(); 00231 pc.printf(replybuff); 00232 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00233 timeout=500; 00234 getcount=10; 00235 SendCMD(); 00236 getreply(); 00237 pc.printf(replybuff); 00238 } else { 00239 sprintf(cmdbuff, "AT+CIPCLOSE=%s\r\n",channel); // close current connection 00240 SendCMD(); 00241 getreply(); 00242 pc.printf(replybuff); 00243 } 00244 t2.reset(); 00245 } 00246 00247 // Reads and processes GET and POST web data 00248 void ReadWebData() 00249 { 00250 wait_ms(200); 00251 esp.attach(NULL); 00252 count=0; 00253 DataRX=0; 00254 weberror=0; 00255 memset(webdata, '\0', sizeof(webdata)); 00256 int x = strcspn (webbuff,"+"); 00257 if(x) { 00258 strcpy(webdata, webbuff + x); 00259 weberror=0; 00260 int numMatched = sscanf(webdata,"+IPD,%d,%d:%s", &linkID, &ipdLen, type); 00261 if( strstr(webdata, "led1=1") != NULL ) { 00262 led1=1; 00263 } 00264 if( strstr(webdata, "led1=0") != NULL ) { 00265 led1=0; 00266 } 00267 if( strstr(webdata, "Out1=1") != NULL ) { 00268 Out1=1; 00269 } 00270 if( strstr(webdata, "Out1=0") != NULL ) { 00271 Out1=0; 00272 } 00273 if( strstr(webdata, "Out2=1") != NULL ) { 00274 Out2=1; 00275 } 00276 if( strstr(webdata, "Out2=0") != NULL ) { 00277 Out2=0; 00278 } 00279 if( strstr(webdata, "Out3=1") != NULL ) { 00280 Out3=1; 00281 } 00282 if( strstr(webdata, "Out3=0") != NULL ) { 00283 Out3=0; 00284 } 00285 sprintf(channel, "%d",linkID); 00286 if (strstr(webdata, "GET") != NULL) { 00287 servreq=1; 00288 } 00289 if (strstr(webdata, "POST") != NULL) { 00290 servreq=1; 00291 } 00292 webcounter++; 00293 sprintf(webcount, "%d",webcounter); 00294 } else { 00295 memset(webbuff, '\0', sizeof(webbuff)); 00296 esp.attach(&callback); 00297 weberror=1; 00298 } 00299 } 00300 // Starts and restarts webserver if errors detected. 00301 void startserver() 00302 { 00303 gettemp(); 00304 gettime(); 00305 pc.printf("\n\n RTC time %s\r\n\n",timebuf); 00306 pc.printf("++++++++++ Resetting ESP ++++++++++\r\n"); 00307 strcpy(cmdbuff,"AT+RST\r\n"); 00308 timeout=8000; 00309 getcount=1000; 00310 SendCMD(); 00311 getreply(); 00312 pc.printf(replybuff); 00313 pc.printf("%d",count); 00314 if (strstr(replybuff, "OK") != NULL) { 00315 pc.printf("\n++++++++++ Starting Server ++++++++++\r\n"); 00316 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); // set multiple connections. 00317 timeout=500; 00318 getcount=20; 00319 SendCMD(); 00320 getreply(); 00321 pc.printf(replybuff); 00322 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00323 timeout=500; 00324 getcount=20; 00325 SendCMD(); 00326 getreply(); 00327 pc.printf(replybuff); 00328 wait(1); 00329 sprintf(cmdbuff,"AT+CIPSTO=%d\r\n",SERVtimeout); 00330 timeout=500; 00331 getcount=50; 00332 SendCMD(); 00333 getreply(); 00334 pc.printf(replybuff); 00335 wait(5); 00336 pc.printf("\n Getting Server IP \r\n"); 00337 strcpy(cmdbuff, "AT+CIFSR\r\n"); 00338 timeout=2500; 00339 getcount=200; 00340 while(weberror==0) { 00341 SendCMD(); 00342 getreply(); 00343 if (strstr(replybuff, "0.0.0.0") == NULL) { 00344 weberror=1; // wait for valid IP 00345 } 00346 } 00347 pc.printf("\n Enter WEB address (IP) found below in your browser \r\n\n"); 00348 pc.printf("\n The MAC address is also shown below,if it is needed \r\n\n"); 00349 replybuff[strlen(replybuff)-1] = '\0'; 00350 //char* IP = replybuff + 5; 00351 sprintf(webdata,"%s", replybuff); 00352 pc.printf(webdata); 00353 led2=1; 00354 bufflen=200; 00355 count=0; 00356 pc.printf("\n\n++++++++++ Ready ++++++++++\r\n\n"); 00357 esp.attach(&callback); 00358 } else { 00359 pc.printf("\n++++++++++ ESP8266 error, check power/connections ++++++++++\r\n"); 00360 while(1) {} 00361 } 00362 t2.reset(); 00363 t2.start(); 00364 beep(); 00365 } 00366 // ESP Command data send 00367 void SendCMD() 00368 { 00369 esp.printf("%s", cmdbuff); 00370 } 00371 // Large WEB buffer data send 00372 void SendWEB() 00373 { 00374 int i=0; 00375 if(esp.writeable()) { 00376 while(webbuff[i]!='\0') { 00377 esp.putc(webbuff[i]); 00378 i++; 00379 } 00380 } 00381 } 00382 // Get Command and ESP status replies 00383 void getreply() 00384 { 00385 memset(replybuff, '\0', sizeof(replybuff)); 00386 t1.reset(); 00387 t1.start(); 00388 replycount=0; 00389 while(t1.read_ms()< timeout && replycount < getcount) { 00390 if(esp.readable()) { 00391 replybuff[replycount] = esp.getc(); 00392 replycount++; 00393 } 00394 } 00395 t1.stop(); 00396 } 00397 // Analog in example 00398 void getbattery() 00399 { 00400 AdcIn=Ain1.read(); 00401 Ht = (AdcIn*3.3); // set the numeric to the exact MCU analog reference voltage for greater accuracy 00402 sprintf(Vcc,"%2.3f",Ht); 00403 } 00404 // Temperature example 00405 void gettemp() 00406 { 00407 00408 AdcIn=Ain2.read(); 00409 Ht = (AdcIn*3.3); // set the numeric to the exact MCU analog reference voltage for greater accuracy 00410 sprintf(Temp,"%2.3f",Ht); 00411 } 00412 // Get RTC time 00413 void gettime() 00414 { 00415 time_t seconds = time(NULL); 00416 strftime(timebuf,50,"%H:%M:%S %a %d %b %y", localtime(&seconds)); 00417 } 00418 00419 void beep() 00420 { 00421 speaker.period(1.0/2000); // 2000hz period 00422 speaker = 0.5; //50% duty cycle - max volume 00423 wait_ms(60); 00424 speaker=0.0; // turn off audio 00425 } 00426 00427 void setRTC() 00428 { 00429 t.tm_sec = (0); // 0-59 00430 t.tm_min = (minute); // 0-59 00431 t.tm_hour = (hour); // 0-23 00432 t.tm_mday = (dayofmonth); // 1-31 00433 t.tm_mon = (month-1); // 0-11 "0" = Jan, -1 added for Mbed RCT clock format 00434 t.tm_year = ((year)+100); // year since 1900, current DCF year + 100 + 1900 = correct year 00435 set_time(mktime(&t)); // set RTC clock 00436 }
Generated on Sun Jul 17 2022 17:00:13 by
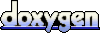