This class adds HTTP, FTP and CellLocate client support for u-blox modules for the C027 and C030 boards (excepting the C030 N2xx flavour) from mbed 5.5 onwards. The HTTP, FTP and CellLocate operations are all hosted on the module, minimizing RAM consumption in the mbed MCU. It also sub-classes ublox-cellular-driver-gen to bring in SMS, USSD and modem file system support if you need to use these functions at the same time as the cellular interface.
Dependencies: ublox-at-cellular-interface
Dependents: example-ublox-at-cellular-interface-ext HelloMQTT ublox_new_driver_test example-ublox-at-cellular-interface-ext ... more
main.cpp
00001 #include "mbed.h" 00002 #include "greentea-client/test_env.h" 00003 #include "unity.h" 00004 #include "utest.h" 00005 #include "UbloxATCellularInterfaceExt.h" 00006 #include "UDPSocket.h" 00007 #ifdef FEATURE_COMMON_PAL 00008 #include "mbed_trace.h" 00009 #define TRACE_GROUP "TEST" 00010 #else 00011 #define tr_debug(format, ...) debug(format "\n", ## __VA_ARGS__) 00012 #define tr_info(format, ...) debug(format "\n", ## __VA_ARGS__) 00013 #define tr_warn(format, ...) debug(format "\n", ## __VA_ARGS__) 00014 #define tr_error(format, ...) debug(format "\n", ## __VA_ARGS__) 00015 #endif 00016 00017 using namespace utest::v1; 00018 00019 // ---------------------------------------------------------------- 00020 // COMPILE-TIME MACROS 00021 // ---------------------------------------------------------------- 00022 00023 // These macros can be overridden with an mbed_app.json file and 00024 // contents of the following form: 00025 // 00026 //{ 00027 // "config": { 00028 // "apn": { 00029 // "value": "\"my_apn\"" 00030 // }, 00031 // "ftp-server": { 00032 // "value": "\"test.rebex.net\"" 00033 // }, 00034 // "ftp-username": { 00035 // "value": "\"demo\"" 00036 // }, 00037 // "ftp-password": { 00038 // "value": "\"password\"" 00039 // }, 00040 // "ftp-use-passive": { 00041 // "value": true 00042 // }, 00043 // "ftp-server-supports-write": { 00044 // "value": false 00045 // }, 00046 // "ftp-filename": { 00047 // "value": "\"readme.txt\"" 00048 // }, 00049 // "ftp-dirname": { 00050 // "value": "\"pub\"" 00051 // } 00052 //} 00053 00054 // Whether debug trace is on 00055 #ifndef MBED_CONF_APP_DEBUG_ON 00056 # define MBED_CONF_APP_DEBUG_ON false 00057 #endif 00058 00059 // The credentials of the SIM in the board. 00060 #ifndef MBED_CONF_APP_DEFAULT_PIN 00061 // Note: if PIN is enabled on your SIM, you must define the PIN 00062 // for your SIM jere (e.g. using mbed_app.json to do so). 00063 # define MBED_CONF_APP_DEFAULT_PIN "0000" 00064 #endif 00065 00066 // Network credentials. 00067 #ifndef MBED_CONF_APP_APN 00068 # define MBED_CONF_APP_APN NULL 00069 #endif 00070 #ifndef MBED_CONF_APP_USERNAME 00071 # define MBED_CONF_APP_USERNAME NULL 00072 #endif 00073 #ifndef MBED_CONF_APP_PASSWORD 00074 # define MBED_CONF_APP_PASSWORD NULL 00075 #endif 00076 00077 // FTP server name 00078 #ifndef MBED_CONF_APP_FTP_SERVER 00079 # error "Must define an FTP server name to run these tests" 00080 #endif 00081 00082 // User name on the FTP server 00083 #ifndef MBED_CONF_APP_FTP_USERNAME 00084 # define MBED_CONF_APP_FTP_SERVER_USERNAME "" 00085 #endif 00086 00087 // Password on the FTP server 00088 #ifndef MBED_CONF_APP_FTP_PASSWORD 00089 # define MBED_CONF_APP_FTP_SERVER_PASSWORD "" 00090 #endif 00091 00092 // Whether to use SFTP or not 00093 #ifndef MBED_CONF_APP_FTP_SECURE 00094 # define MBED_CONF_APP_FTP_SECURE false 00095 #endif 00096 00097 // Port to use on the remote server 00098 #ifndef MBED_CONF_APP_FTP_SERVER_PORT 00099 # if MBED_CONF_APP_FTP_SECURE 00100 # define MBED_CONF_APP_FTP_SERVER_PORT 22 00101 # else 00102 # define MBED_CONF_APP_FTP_SERVER_PORT 21 00103 # endif 00104 #endif 00105 00106 // Whether to use passive or active mode 00107 // default to true as many servers/networks 00108 // require this 00109 #ifndef MBED_CONF_APP_FTP_USE_PASSIVE 00110 # define MBED_CONF_APP_FTP_USE_PASSIVE true 00111 #endif 00112 00113 // Whether the server supports FTP write operations 00114 #ifndef MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00115 # define MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE false 00116 #endif 00117 00118 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00119 // The name of the file to PUT, GET and then delete 00120 # ifndef MBED_CONF_APP_FTP_FILENAME 00121 # define MBED_CONF_APP_FTP_FILENAME "test_file_delete_me" 00122 # endif 00123 // The name of the directory to create, CD to and then remove 00124 // Must not be a substring of MBED_CONF_APP_FTP_FILENAME. 00125 # ifndef MBED_CONF_APP_FTP_DIRNAME 00126 # define MBED_CONF_APP_FTP_DIRNAME "test_dir_delete_me" 00127 # endif 00128 #else 00129 // The name of the file to GET 00130 # ifndef MBED_CONF_APP_FTP_FILENAME 00131 # error "Must define the name of a file you know exists on the FTP server" 00132 # endif 00133 // The name of the directory to CD to 00134 # ifndef MBED_CONF_APP_FTP_DIRNAME 00135 # error "Must define the name of a directory you know exists on the FTP server" 00136 # endif 00137 #endif 00138 00139 // The size of file when testing PUT/GET 00140 #ifndef MBED_CONF_APP_FTP_FILE_SIZE 00141 # define MBED_CONF_APP_FTP_FILE_SIZE 1200 00142 #endif 00143 00144 // ---------------------------------------------------------------- 00145 // PRIVATE VARIABLES 00146 // ---------------------------------------------------------------- 00147 00148 #ifdef FEATURE_COMMON_PAL 00149 // Lock for debug prints 00150 static Mutex mtx; 00151 #endif 00152 00153 // An instance of the cellular interface 00154 static UbloxATCellularInterfaceExt *pDriver = 00155 new UbloxATCellularInterfaceExt(MDMTXD, MDMRXD, 00156 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00157 MBED_CONF_APP_DEBUG_ON); 00158 // A buffer for general use 00159 static char buf[MBED_CONF_APP_FTP_FILE_SIZE]; 00160 00161 // ---------------------------------------------------------------- 00162 // PRIVATE FUNCTIONS 00163 // ---------------------------------------------------------------- 00164 00165 #ifdef FEATURE_COMMON_PAL 00166 // Locks for debug prints 00167 static void lock() 00168 { 00169 mtx.lock(); 00170 } 00171 00172 static void unlock() 00173 { 00174 mtx.unlock(); 00175 } 00176 #endif 00177 00178 // Write a file to the module's file system with known contents 00179 void createFile(const char * filename) { 00180 00181 for (unsigned int x = 0; x < sizeof (buf); x++) { 00182 buf[x] = (char) x; 00183 } 00184 00185 TEST_ASSERT(pDriver->writeFile(filename, buf, sizeof (buf)) == sizeof (buf)); 00186 tr_debug("%d bytes written to file \"%s\"", sizeof (buf), filename); 00187 } 00188 00189 // Read a file back from the module's file system and check the contents 00190 void checkFile(const char * filename) { 00191 memset(buf, 0, sizeof (buf)); 00192 00193 int x = pDriver->readFile(filename, buf, sizeof (buf)); 00194 tr_debug ("File is %d bytes big", x); 00195 TEST_ASSERT(x == sizeof (buf)); 00196 00197 tr_debug("%d bytes read from file \"%s\"", sizeof (buf), filename); 00198 00199 for (unsigned int x = 0; x < sizeof (buf); x++) { 00200 TEST_ASSERT(buf[x] == (char) x); 00201 } 00202 } 00203 00204 // ---------------------------------------------------------------- 00205 // TESTS 00206 // ---------------------------------------------------------------- 00207 00208 // Test the setting up of parameters, connection and login to an FTP session 00209 void test_ftp_login() { 00210 SocketAddress address; 00211 char portString[10]; 00212 00213 sprintf(portString, "%d", MBED_CONF_APP_FTP_SERVER_PORT); 00214 #ifdef TARGET_UBLOX_C030_R41XM 00215 int mno_profile; 00216 if (pDriver->init(MBED_CONF_APP_DEFAULT_PIN) == false) //init can return false if profile set is SW_DEFAULT 00217 { 00218 TEST_ASSERT(pDriver->get_mno_profile(&mno_profile)); 00219 if (mno_profile == UbloxATCellularInterface::SW_DEFAULT) { 00220 TEST_ASSERT(pDriver->set_mno_profile(UbloxATCellularInterface::STANDARD_EU)); 00221 TEST_ASSERT(pDriver->reboot_modem()); 00222 tr_debug("Reboot successful\n"); 00223 ThisThread::sleep_for(5000); 00224 } 00225 } 00226 TEST_ASSERT(pDriver->init(MBED_CONF_APP_DEFAULT_PIN)); 00227 TEST_ASSERT(pDriver->disable_power_saving_mode()); 00228 #else 00229 TEST_ASSERT(pDriver->init(MBED_CONF_APP_DEFAULT_PIN)); 00230 #endif 00231 00232 // Reset parameters to default to begin with 00233 pDriver->ftpResetPar(); 00234 00235 // Set a timeout for FTP commands 00236 TEST_ASSERT(pDriver->ftpSetTimeout(60000)); 00237 00238 // Set up the FTP server parameters 00239 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SERVER_NAME, 00240 MBED_CONF_APP_FTP_SERVER)); 00241 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SERVER_PORT, 00242 portString)); 00243 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_USER_NAME, 00244 MBED_CONF_APP_FTP_USERNAME)); 00245 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_PASSWORD, 00246 MBED_CONF_APP_FTP_PASSWORD)); 00247 #ifdef MBED_CONF_APP_FTP_ACCOUNT 00248 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_ACCOUNT, 00249 MBED_CONF_APP_FTP_ACCOUNT)); 00250 #endif 00251 #if MBED_CONF_APP_FTP_SECURE 00252 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SECURE, "1")); 00253 #endif 00254 #if MBED_CONF_APP_FTP_USE_PASSIVE 00255 TEST_ASSERT(pDriver->ftpSetPar(UbloxATCellularInterfaceExt::FTP_MODE, "1")); 00256 #endif 00257 00258 // Now connect to the network 00259 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00260 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00261 00262 // Get the server IP address, purely to make sure it's there 00263 TEST_ASSERT(pDriver->gethostbyname(MBED_CONF_APP_FTP_SERVER, &address) == 0); 00264 tr_debug ("Using FTP \"%s\", which is at %s", MBED_CONF_APP_FTP_SERVER, 00265 address.get_ip_address()); 00266 00267 // Log into the FTP server 00268 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LOGIN) == NULL); 00269 } 00270 00271 // Test FTP directory listing 00272 void test_ftp_dir() { 00273 // Get a directory listing 00274 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00275 NULL, NULL, buf, sizeof (buf)) == NULL); 00276 tr_debug("Listing:\n%s", buf); 00277 00278 // The file we will GET should appear in the directory listing 00279 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME)); 00280 // As should the directory name we will change to 00281 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME)); 00282 } 00283 00284 // Test FTP file information 00285 void test_ftp_fileinfo() { 00286 // Get the info 00287 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_FILE_INFO, 00288 MBED_CONF_APP_FTP_FILENAME, NULL, 00289 buf, sizeof (buf)) == NULL); 00290 tr_debug("File info:\n%s", buf); 00291 00292 // The file info string should at least include the file name 00293 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME)); 00294 } 00295 00296 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00297 00298 // In case a previous test failed half way, do some cleaning up first 00299 // Note: don't check return values as these operations will fail 00300 // if there's nothing to clean up 00301 void test_ftp_write_cleanup() { 00302 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00303 MBED_CONF_APP_FTP_FILENAME); 00304 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00305 MBED_CONF_APP_FTP_FILENAME "_2"); 00306 pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RMDIR, 00307 MBED_CONF_APP_FTP_DIRNAME); 00308 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME); 00309 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME "_1"); 00310 } 00311 00312 // Test FTP put and then get 00313 void test_ftp_put_get() { 00314 // Create the file 00315 createFile(MBED_CONF_APP_FTP_FILENAME); 00316 00317 // Put the file 00318 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_PUT_FILE, 00319 MBED_CONF_APP_FTP_FILENAME) == NULL); 00320 00321 // Get the file 00322 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_GET_FILE, 00323 MBED_CONF_APP_FTP_FILENAME, 00324 MBED_CONF_APP_FTP_FILENAME "_1") == NULL); 00325 00326 // Check that it is the same as we sent 00327 checkFile(MBED_CONF_APP_FTP_FILENAME "_1"); 00328 } 00329 00330 // Test FTP rename file 00331 void test_ftp_rename() { 00332 // Get a directory listing 00333 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00334 NULL, NULL, buf, sizeof (buf)) == NULL); 00335 tr_debug("Listing:\n%s", buf); 00336 00337 // The file we are renaming to should not appear 00338 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00339 00340 // Rename the file 00341 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RENAME_FILE, 00342 MBED_CONF_APP_FTP_FILENAME, 00343 MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00344 00345 // Get a directory listing 00346 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00347 NULL, NULL, buf, sizeof (buf)) == NULL); 00348 tr_debug("Listing:\n%s", buf); 00349 00350 // The new file should now exist 00351 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") > NULL); 00352 00353 } 00354 00355 // Test FTP delete file 00356 void test_ftp_delete() { 00357 // Get a directory listing 00358 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00359 NULL, NULL, buf, sizeof (buf)) == NULL); 00360 tr_debug("Listing:\n%s", buf); 00361 00362 // The file we are to delete should appear in the list 00363 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") > NULL); 00364 00365 // Delete the file 00366 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_DELETE_FILE, 00367 MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00368 00369 // Get a directory listing 00370 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00371 NULL, NULL, buf, sizeof (buf)) == NULL); 00372 tr_debug("Listing:\n%s", buf); 00373 00374 // The file we deleted should no longer appear in the list 00375 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_FILENAME "_2") == NULL); 00376 } 00377 00378 // Test FTP MKDIR 00379 void test_ftp_mkdir() { 00380 // Get a directory listing 00381 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00382 NULL, NULL, buf, sizeof (buf)) == NULL); 00383 tr_debug("Listing:\n%s", buf); 00384 00385 // The directory we are to create should not appear in the list 00386 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00387 00388 // Create the directory 00389 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_MKDIR, 00390 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00391 00392 // Get a directory listing 00393 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00394 NULL, NULL, buf, sizeof (buf)) == NULL); 00395 tr_debug("Listing:\n%s", buf); 00396 00397 // The directory we created should now appear in the list 00398 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00399 } 00400 00401 // Test FTP RMDIR 00402 void test_ftp_rmdir() { 00403 // Get a directory listing 00404 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00405 NULL, NULL, buf, sizeof (buf)) == NULL); 00406 tr_debug("Listing:\n%s", buf); 00407 00408 // The directory we are to remove should appear in the list 00409 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) > NULL); 00410 00411 // Remove the directory 00412 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_RMDIR, 00413 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00414 00415 // Get a directory listing 00416 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00417 NULL, NULL, buf, sizeof (buf)) == NULL); 00418 tr_debug("Listing:\n%s", buf); 00419 00420 // The directory we removed should no longer appear in the list 00421 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00422 } 00423 00424 #endif // MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00425 00426 // Test FTP get 00427 void test_ftp_get() { 00428 // Make sure that the 'get' filename we're going to use 00429 // isn't already here (but don't assert on this one 00430 // as, if the file isn't there, we will get an error) 00431 pDriver->delFile(MBED_CONF_APP_FTP_FILENAME); 00432 00433 // Get the file 00434 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_GET_FILE, 00435 MBED_CONF_APP_FTP_FILENAME) == NULL); 00436 00437 // Check that it has arrived 00438 TEST_ASSERT(pDriver->fileSize(MBED_CONF_APP_FTP_FILENAME) > 0); 00439 } 00440 00441 // Test FTP change directory 00442 void test_ftp_cd() { 00443 // Get a directory listing 00444 *buf = 0; 00445 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00446 NULL, NULL, buf, sizeof (buf)) == NULL); 00447 00448 tr_debug("Listing:\n%s", buf); 00449 00450 // The listing should include the directory name we are going to move to 00451 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME)); 00452 00453 // Change directories 00454 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_CD, 00455 MBED_CONF_APP_FTP_DIRNAME) == NULL); 00456 // Get a directory listing 00457 *buf = 0; 00458 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00459 NULL, NULL, buf, sizeof (buf)) == NULL); 00460 tr_debug("Listing:\n%s", buf); 00461 00462 // The listing should no longer include the directory name we have moved 00463 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME) == NULL); 00464 00465 // Go back to where we were 00466 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_CD, "..") 00467 == NULL); 00468 00469 // Get a directory listing 00470 *buf = 0; 00471 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00472 NULL, NULL, buf, sizeof (buf)) == NULL); 00473 tr_debug("Listing:\n%s", buf); 00474 00475 // The listing should include the directory name we went to once more 00476 TEST_ASSERT(strstr(buf, MBED_CONF_APP_FTP_DIRNAME)); 00477 } 00478 00479 #ifdef MBED_CONF_APP_FTP_FOTA_FILENAME 00480 // Test FTP FOTA 00481 // TODO: test not tested as I don't have a module that supports the FTP FOTA operation 00482 void test_ftp_fota() { 00483 *buf = 0; 00484 // Do FOTA on a file 00485 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_FOTA_FILE, 00486 MBED_CONF_APP_FTP_FOTA_FILENAME, NULL, 00487 buf, sizeof (buf)) == NULL); 00488 tr_debug("MD5 sum: %s\n", buf); 00489 00490 // Check that the 128 bit MD5 sum is now there 00491 TEST_ASSERT(strlen (buf) == 32); 00492 } 00493 #endif 00494 00495 // Test logout and disconnect from an FTP session 00496 void test_ftp_logout() { 00497 // Log out from the FTP server 00498 TEST_ASSERT(pDriver->ftpCommand(UbloxATCellularInterfaceExt::FTP_LOGOUT) == NULL); 00499 00500 TEST_ASSERT(pDriver->disconnect() == 0); 00501 00502 // Wait for printfs to leave the building or the test result string gets messed up 00503 ThisThread::sleep_for(500); 00504 } 00505 00506 // ---------------------------------------------------------------- 00507 // TEST ENVIRONMENT 00508 // ---------------------------------------------------------------- 00509 00510 // Setup the test environment 00511 utest::v1::status_t test_setup(const size_t number_of_cases) { 00512 // Setup Greentea with a timeout 00513 GREENTEA_SETUP(540, "default_auto"); 00514 return verbose_test_setup_handler(number_of_cases); 00515 } 00516 00517 // Test cases 00518 Case cases[] = { 00519 Case("FTP log in", test_ftp_login), 00520 #if MBED_CONF_APP_FTP_SERVER_SUPPORTS_WRITE 00521 Case("Clean-up", test_ftp_write_cleanup), 00522 Case("FTP put and get", test_ftp_put_get), 00523 Case("FTP file info", test_ftp_fileinfo), 00524 Case("FTP rename", test_ftp_rename), 00525 Case("FTP make directory", test_ftp_mkdir), 00526 Case("FTP directory list", test_ftp_dir), Case("FTP delete", test_ftp_delete), 00527 Case("FTP change directory", test_ftp_cd), 00528 Case("FTP delete directory", test_ftp_rmdir), 00529 #else 00530 Case("FTP directory list", test_ftp_dir), 00531 Case("FTP file info", test_ftp_fileinfo), 00532 Case("FTP get", test_ftp_get), 00533 Case("FTP change directory", test_ftp_cd), 00534 #endif 00535 #ifdef MBED_CONF_APP_FTP_FOTA_FILENAME 00536 Case("FTP FOTA", test_ftp_fota), 00537 #endif 00538 Case("FTP log out", test_ftp_logout) 00539 }; 00540 00541 Specification specification(test_setup, cases); 00542 00543 // ---------------------------------------------------------------- 00544 // MAIN 00545 // ---------------------------------------------------------------- 00546 00547 int main() { 00548 00549 #ifdef FEATURE_COMMON_PAL 00550 mbed_trace_init(); 00551 00552 mbed_trace_mutex_wait_function_set(lock); 00553 mbed_trace_mutex_release_function_set(unlock); 00554 #endif 00555 00556 // Run tests 00557 return !Harness::run(specification); 00558 } 00559 00560 // End Of File 00561
Generated on Tue Jul 12 2022 21:43:58 by
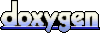