
WiFi AP example for Odin-W2
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* WiFi AP Example 00002 * Copyright (c) 2018 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "OdinWiFiInterface.h" 00019 #include "TCPSocket.h" 00020 #include <string> 00021 00022 #ifdef DEVICE_WIFI_AP 00023 static const char *wifi_ssid = MBED_CONF_APP_WIFI_SSID; 00024 static const char *wifi_password = MBED_CONF_APP_WIFI_PASSWORD; 00025 static const char *ap_ip = MBED_CONF_APP_AP_IP; 00026 static const char *ap_netmask = MBED_CONF_APP_AP_NETMASK; 00027 static const char *ap_gateway = MBED_CONF_APP_AP_GATEWAY; 00028 #endif 00029 00030 #define ECHO_SERVER_PORT 5050 00031 00032 OdinWiFiInterface *_wifi; 00033 00034 00035 static void start_ap(nsapi_security_t security = NSAPI_SECURITY_WPA_WPA2) 00036 { 00037 nsapi_error_t error_code; 00038 00039 printf("\nStarting AP\n"); 00040 00041 // AP Configure and start 00042 error_code = _wifi->set_ap_network(ap_ip, ap_netmask, ap_gateway); 00043 MBED_ASSERT(error_code == NSAPI_ERROR_OK); 00044 00045 //DHCP not available 00046 error_code = _wifi->set_ap_dhcp(false); 00047 MBED_ASSERT(error_code == NSAPI_ERROR_OK); 00048 00049 //Set beacon interval to default value 00050 _wifi->set_ap_beacon_interval(100); 00051 00052 //Set ap ssid, password and channel 00053 error_code = _wifi->ap_start(wifi_ssid, wifi_password, security, cbWLAN_CHANNEL_01); 00054 MBED_ASSERT(error_code == NSAPI_ERROR_OK); 00055 00056 printf("\nAP started successfully\n"); 00057 } 00058 00059 00060 static void stop_ap() 00061 { 00062 nsapi_error_t error_code; 00063 00064 error_code = _wifi->ap_stop(); 00065 MBED_ASSERT(error_code == NSAPI_ERROR_OK); 00066 00067 printf("\nAP stopped\n"); 00068 00069 } 00070 00071 int main() 00072 { 00073 nsapi_size_or_error_t errcode; 00074 nsapi_error_t *err; 00075 TCPSocket sock, *sock_data; 00076 int n = 0; 00077 char recv_buf[1024]; 00078 00079 /*Start AP*/ 00080 00081 _wifi = new OdinWiFiInterface(true); 00082 00083 start_ap(); 00084 00085 /*Socket initialization*/ 00086 00087 errcode = sock.open(_wifi); 00088 if (errcode != NSAPI_ERROR_OK) { 00089 printf("TCPSocket.open() fails, code: %d\n", errcode); 00090 return -1; 00091 } 00092 00093 errcode = sock.bind("10.0.0.1", ECHO_SERVER_PORT); 00094 if (errcode < 0) { 00095 printf("TCPSocket.connect() fails, code: %d\n", errcode); 00096 return -1; 00097 } 00098 else { 00099 printf("TCP: connected with %s server\n", "10.0.0.1"); 00100 } 00101 00102 /*Echo server*/ 00103 if (sock.listen() == 0) 00104 { 00105 sock_data = sock.accept(err = NULL); 00106 00107 if (sock_data != NULL) 00108 { 00109 while (true) 00110 { 00111 n = sock_data->recv((void*) recv_buf, sizeof(recv_buf)); 00112 if (n > 0) 00113 { 00114 printf("\n Received from client %d bytes: %s \n", n, recv_buf); 00115 00116 errcode = sock_data->send((void*) recv_buf, n); 00117 if (errcode < 0) 00118 { 00119 printf("\n TCPSocket.send() fails, code: %d\n", errcode); 00120 return -1; 00121 } 00122 else 00123 { 00124 printf("\n TCP: Sent %d Bytes to client\n", n); 00125 } 00126 } 00127 else 00128 { 00129 printf("\n TCPSocket.recv() failed"); 00130 return -1; 00131 } 00132 00133 } 00134 00135 } 00136 00137 } 00138 00139 sock_data->close(); 00140 sock.close(); 00141 00142 stop_ap(); 00143 00144 return 0; 00145 }
Generated on Wed Jul 13 2022 02:14:15 by
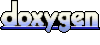