
Example program running mbedClient over UbloxATCellularInterface or OnboardCellularInterface for the C030 platform.
Dependencies: ublox-cellular-base ublox-at-cellular-interface ublox-ppp-cellular-interface ublox-at-cellular-interface-n2xx ublox-cellular-base-n2xx
simpleclient.h
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __SIMPLECLIENT_H__ 00018 #define __SIMPLECLIENT_H__ 00019 00020 #include "mbed-client/m2minterfacefactory.h" 00021 #include "mbed-client/m2mdevice.h" 00022 #include "mbed-client/m2minterfaceobserver.h" 00023 #include "mbed-client/m2minterface.h" 00024 #include "mbed-client/m2mobject.h" 00025 #include "mbed-client/m2mobjectinstance.h" 00026 #include "mbed-client/m2mresource.h" 00027 #include "mbed-client/m2mconfig.h" 00028 #include "mbed-client/m2mblockmessage.h" 00029 #include "security.h" 00030 #include "mbed.h" 00031 00032 #define STRINGIFY(s) #s 00033 00034 M2MInterface::NetworkStack NETWORK_STACK = M2MInterface::LwIP_IPv4; 00035 00036 M2MInterface::BindingMode SOCKET_MODE = M2MInterface::UDP; 00037 00038 struct MbedClientDevice { 00039 const char* Manufacturer; 00040 const char* Type; 00041 const char* ModelNumber; 00042 const char* SerialNumber; 00043 }; 00044 00045 /* 00046 * Wrapper for mbed client stack that handles all callbacks, error handling, and 00047 * other shenanigans to make the mbed client stack easier to use. 00048 * 00049 * The end user should only have to care about configuring the parameters at the 00050 * top of this file and making sure they add the security.h file correctly. 00051 */ 00052 class MbedClient: public M2MInterfaceObserver { 00053 00054 public: 00055 00056 /* 00057 * Constructor for MbedClient object, initialize private variables 00058 */ 00059 MbedClient(struct MbedClientDevice device) 00060 { 00061 _interface = NULL; 00062 _bootstrapped = false; 00063 _error = false; 00064 _registered = false; 00065 _unregistered = false; 00066 _register_security = NULL; 00067 _value = 0; 00068 _object = NULL; 00069 _device = device; 00070 } 00071 00072 /* 00073 * Destructor for MbedClient object, you can ignore this 00074 */ 00075 ~MbedClient() 00076 { 00077 if (_interface) { 00078 delete _interface; 00079 } 00080 if (_register_security) { 00081 delete _register_security; 00082 } 00083 } 00084 00085 /* 00086 * Creates M2MInterface using which endpoint can 00087 * setup its name, resource type, life time, connection mode, 00088 * Currently only LwIPv4 is supported. 00089 */ 00090 void create_interface(const char *server_address, void *handler=NULL) 00091 { 00092 // Randomizing listening port for Certificate mode connectivity 00093 _server_address = server_address; 00094 uint16_t port = 0; // Network interface will randomize with port 0 00095 00096 // Create mDS interface object, this is the base object everything else attaches to 00097 _interface = M2MInterfaceFactory::create_interface(*this, 00098 MBED_ENDPOINT_NAME, // endpoint name string 00099 "test", // endpoint type string 00100 100, // lifetime 00101 port, // listen port 00102 MBED_DOMAIN, // domain string 00103 SOCKET_MODE, // binding mode 00104 NETWORK_STACK, // network stack 00105 ""); // context address string 00106 const char *binding_mode = (SOCKET_MODE == M2MInterface::UDP) ? "UDP" : "TCP"; 00107 printf("SOCKET_MODE : %s\n", binding_mode); 00108 printf("Connecting to %s\n", server_address); 00109 00110 if (_interface) { 00111 _interface->set_platform_network_handler(handler); 00112 } 00113 } 00114 00115 /* 00116 * Check private variable to see if the registration was sucessful or not 00117 */ 00118 bool register_successful() 00119 { 00120 return _registered; 00121 } 00122 00123 /* 00124 * Check private variable to see if un-registration was sucessful or not 00125 */ 00126 bool unregister_successful() 00127 { 00128 return _unregistered; 00129 } 00130 00131 /* 00132 * Creates register server object with mbed device server address and other parameters 00133 * required for client to connect to mbed device server. 00134 */ 00135 M2MSecurity* create_register_object() 00136 { 00137 // Create security object using the interface factory. 00138 // this will generate a security ObjectID and ObjectInstance 00139 M2MSecurity *security = M2MInterfaceFactory::create_security(M2MSecurity::M2MServer); 00140 00141 // Make sure security ObjectID/ObjectInstance was created successfully 00142 if (security) { 00143 // Add ResourceID's and values to the security ObjectID/ObjectInstance 00144 security->set_resource_value(M2MSecurity::M2MServerUri, _server_address); 00145 security->set_resource_value(M2MSecurity::SecurityMode, M2MSecurity::Certificate); 00146 security->set_resource_value(M2MSecurity::ServerPublicKey, SERVER_CERT, sizeof(SERVER_CERT) - 1); 00147 security->set_resource_value(M2MSecurity::PublicKey, CERT, sizeof(CERT) - 1); 00148 security->set_resource_value(M2MSecurity::Secretkey, KEY, sizeof(KEY) - 1); 00149 } 00150 return security; 00151 } 00152 00153 /* 00154 * Creates device object which contains mandatory resources linked with 00155 * device endpoint. 00156 */ 00157 M2MDevice* create_device_object() 00158 { 00159 // Create device objectID/ObjectInstance 00160 M2MDevice *device = M2MInterfaceFactory::create_device(); 00161 // Make sure device object was created successfully 00162 if (device) { 00163 // Add resourceID's to device objectID/ObjectInstance 00164 device->create_resource(M2MDevice::Manufacturer, _device.Manufacturer); 00165 device->create_resource(M2MDevice::DeviceType, _device.Type); 00166 device->create_resource(M2MDevice::ModelNumber, _device.ModelNumber); 00167 device->create_resource(M2MDevice::SerialNumber, _device.SerialNumber); 00168 } 00169 return device; 00170 } 00171 00172 /* 00173 * Register an object 00174 */ 00175 void test_register(M2MSecurity *register_object, M2MObjectList object_list) 00176 { 00177 if (_interface) { 00178 // Register function 00179 _interface->register_object(register_object, object_list); 00180 } 00181 } 00182 00183 /* 00184 * Unregister all objects 00185 */ 00186 void test_unregister() 00187 { 00188 if (_interface) { 00189 // Unregister function 00190 _interface->unregister_object(NULL); // NULL will unregister all objects 00191 } 00192 } 00193 00194 /* 00195 * Callback from mbed client stack when the bootstrap 00196 * is successful, it returns the mbed Device Server object 00197 * which will be used for registering the resources to 00198 * mbed Device server. 00199 */ 00200 void bootstrap_done(M2MSecurity *server_object) 00201 { 00202 if (server_object) { 00203 _bootstrapped = true; 00204 _error = false; 00205 printf("Bootstrapped\n"); 00206 } 00207 } 00208 00209 /* 00210 * Callback from mbed client stack when the registration 00211 * is successful, it returns the mbed Device Server object 00212 * to which the resources are registered and registered objects. 00213 */ 00214 void object_registered(M2MSecurity */*security_object*/, const M2MServer & /*server_object*/) 00215 { 00216 _registered = true; 00217 _unregistered = false; 00218 printf("Registered object successfully!\n"); 00219 } 00220 00221 /* 00222 * Callback from mbed client stack when the unregistration 00223 * is successful, it returns the mbed Device Server object 00224 * to which the resources were unregistered. 00225 */ 00226 void object_unregistered(M2MSecurity */*server_object*/) 00227 { 00228 printf("Unregistered object successfully\n"); 00229 _unregistered = true; 00230 _registered = false; 00231 } 00232 00233 /* 00234 * Callback from mbed client stack when registration is updated 00235 */ 00236 void registration_updated(M2MSecurity */*security_object*/, const M2MServer & /*server_object*/) 00237 { 00238 /* The registration is updated automatically and frequently by the 00239 * mbed client stack. This print statement is turned off because it 00240 * tends to happen a lot. 00241 */ 00242 //printf("Registration updated\n"); 00243 } 00244 00245 /* 00246 * Callback from mbed client stack if any error is encountered 00247 * during any of the LWM2M operations. Error type is passed in 00248 * the callback. 00249 */ 00250 void error(M2MInterface::Error error) 00251 { 00252 _error = true; 00253 switch (error){ 00254 case M2MInterface::AlreadyExists: 00255 printf("[ERROR:] M2MInterface::AlreadyExist\n"); 00256 break; 00257 case M2MInterface::BootstrapFailed: 00258 printf("[ERROR:] M2MInterface::BootstrapFailed\n"); 00259 break; 00260 case M2MInterface::InvalidParameters: 00261 printf("[ERROR:] M2MInterface::InvalidParameters\n"); 00262 break; 00263 case M2MInterface::NotRegistered: 00264 printf("[ERROR:] M2MInterface::NotRegistered\n"); 00265 break; 00266 case M2MInterface::Timeout: 00267 printf("[ERROR:] M2MInterface::Timeout\n"); 00268 break; 00269 case M2MInterface::NetworkError: 00270 printf("[ERROR:] M2MInterface::NetworkError\n"); 00271 break; 00272 case M2MInterface::ResponseParseFailed: 00273 printf("[ERROR:] M2MInterface::ResponseParseFailed\n"); 00274 break; 00275 case M2MInterface::UnknownError: 00276 printf("[ERROR:] M2MInterface::UnknownError\n"); 00277 break; 00278 case M2MInterface::MemoryFail: 00279 printf("[ERROR:] M2MInterface::MemoryFail\n"); 00280 break; 00281 case M2MInterface::NotAllowed: 00282 printf("[ERROR:] M2MInterface::NotAllowed\n"); 00283 break; 00284 case M2MInterface::SecureConnectionFailed: 00285 printf("[ERROR:] M2MInterface::SecureConnectionFailed\n"); 00286 break; 00287 case M2MInterface::DnsResolvingFailed: 00288 printf("[ERROR:] M2MInterface::DnsResolvingFailed\n"); 00289 break; 00290 default: 00291 break; 00292 } 00293 } 00294 00295 /* 00296 * Callback from mbed client stack if any value has changed 00297 * during PUT operation. Object and its type is passed in 00298 * the callback. 00299 * BaseType enum from m2mbase.h 00300 * Object = 0x0, Resource = 0x1, ObjectInstance = 0x2, ResourceInstance = 0x3 00301 */ 00302 void value_updated(M2MBase *base, M2MBase::BaseType type) 00303 { 00304 printf("PUT request received!"); 00305 printf("Name :'%s', \nPath : '%s', \nType : '%d' (0 for Object, 1 for Resource), \nType : '%s'\n", 00306 base->name(), 00307 base->uri_path(), 00308 type, 00309 base->resource_type()); 00310 } 00311 00312 /* 00313 * Update the registration period 00314 */ 00315 void test_update_register() 00316 { 00317 if (_registered) { 00318 _interface->update_registration(_register_security, 100); 00319 } 00320 } 00321 00322 /* 00323 * Manually configure the security object private variable 00324 */ 00325 void set_register_object(M2MSecurity *register_object) 00326 { 00327 if (_register_security == NULL) { 00328 _register_security = register_object; 00329 } 00330 } 00331 00332 private: 00333 00334 /* 00335 * Private variables used in class 00336 */ 00337 M2MInterface *_interface; 00338 M2MSecurity *_register_security; 00339 M2MObject *_object; 00340 volatile bool _bootstrapped; 00341 volatile bool _error; 00342 volatile bool _registered; 00343 volatile bool _unregistered; 00344 int _value; 00345 struct MbedClientDevice _device; 00346 String _server_address; 00347 }; 00348 00349 #endif // __SIMPLECLIENT_H__
Generated on Tue Jul 12 2022 21:01:19 by
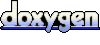