
Example program running mbedClient over UbloxATCellularInterface or OnboardCellularInterface for the C030 platform.
Dependencies: ublox-cellular-base ublox-at-cellular-interface ublox-ppp-cellular-interface ublox-at-cellular-interface-n2xx ublox-cellular-base-n2xx
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #define __STDC_FORMAT_MACROS 00018 #include <inttypes.h> 00019 #include <string> 00020 #include <sstream> 00021 #include <vector> 00022 00023 #include "mbed.h" 00024 #include "mbedtls/entropy_poll.h" 00025 #include "UbloxATCellularInterface.h" 00026 #include "OnboardCellularInterface.h" 00027 #include "UbloxATCellularInterfaceN2xx.h" 00028 #include "simpleclient.h" 00029 #include "security.h" 00030 #include "mbed_trace.h" 00031 #include "mbed.h" 00032 00033 // You must select the correct interface library for your board, by 00034 // uncommenting the correct line below. Supported combinations are 00035 // indicated with a "Y" in the table below. 00036 // 00037 // C030_U201 C030_N211 00038 // UbloxATCellularInterface Y - 00039 // OnboardCellularInterface Y - 00040 // UbloxATCellularInterfaceN2xx - Y 00041 // Note: the N211 module supports only UDP, not TCP 00042 00043 // OnboardCellularInterface uses LWIP and the PPP cellular interface 00044 // on the mbed MCU, while using UbloxATCellularInterface and 00045 // UbloxATCellularInterfaceN2xx uses an IP stack on the cellular 00046 // module and hence uses less RAM. This also allows other AT command 00047 // operations (e.g. sending an SMS) to happen during a data transfer 00048 // (for which you should replace the UbloxATCellularInterface library with 00049 // the UbloxATCellularInterfaceExt library). However, it is slower than 00050 // using the LWIP/PPP on the mbed MCU interface since more string parsing 00051 // is required. 00052 #define INTERFACE_CLASS UbloxATCellularInterface 00053 //#define INTERFACE_CLASS OnboardCellularInterface 00054 //#define INTERFACE_CLASS UbloxATCellularInterfaceN2xx 00055 00056 // The credentials of the SIM in the board. If PIN checking is enabled 00057 // for your SIM card you must set this to the required PIN. 00058 #define PIN "0000" 00059 00060 // Network credentials. You should set this according to your 00061 // network/SIM card. For C030 non-N2xx boards, leave the parameters as NULL 00062 // otherwise, if you do not know the APN for your network, you may 00063 // either try the fairly common "internet" for the APN (and leave the 00064 // username and password NULL), or you may leave all three as NULL and then 00065 // a lookup will be attempted for a small number of known networks 00066 // (see APN_db.h in mbed-os/features/netsocket/cellular/utils). 00067 #define APN NULL 00068 #define USERNAME NULL 00069 #define PASSWORD NULL 00070 00071 // LEDs 00072 DigitalOut ledRed(LED1, 1); 00073 DigitalOut ledGreen(LED2, 1); 00074 DigitalOut ledBlue(LED3, 1); 00075 00076 // The user button 00077 volatile bool buttonPressed = false; 00078 00079 static void good() { 00080 ledGreen = 0; 00081 ledBlue = 1; 00082 ledRed = 1; 00083 } 00084 00085 static void bad() { 00086 ledRed = 0; 00087 ledGreen = 1; 00088 ledBlue = 1; 00089 } 00090 00091 static void event() { 00092 ledBlue = 0; 00093 ledRed = 1; 00094 ledGreen = 1; 00095 } 00096 00097 static void pulseEvent() { 00098 event(); 00099 wait_ms(500); 00100 good(); 00101 } 00102 00103 static void ledOff() { 00104 ledBlue = 1; 00105 ledRed = 1; 00106 ledGreen = 1; 00107 } 00108 00109 // Resource values for the Device Object 00110 struct MbedClientDevice device = { 00111 "Manufacturer", // Manufacturer 00112 "Type", // Type 00113 "ModelNumber", // ModelNumber 00114 "SerialNumber" // SerialNumber 00115 }; 00116 00117 class LedResource { 00118 public: 00119 LedResource() { 00120 ledObject = M2MInterfaceFactory::create_object("3311"); 00121 M2MObjectInstance *inst = ledObject->create_object_instance(); 00122 00123 // An observable resource 00124 M2MResource *onResource = inst->create_dynamic_resource("5850", "On/Off", M2MResourceInstance::BOOLEAN, true); 00125 onResource->set_operation(M2MBase::GET_PUT_ALLOWED); 00126 onResource->set_value(false); 00127 00128 // An multi-valued resource 00129 M2MResource *dimmerResource = inst->create_dynamic_resource("5851", "Dimmer", M2MResourceInstance::BOOLEAN, false); 00130 dimmerResource->set_operation(M2MBase::GET_PUT_ALLOWED); 00131 dimmerResource->set_value(false); 00132 } 00133 00134 ~LedResource() { 00135 } 00136 00137 M2MObject *get_object() { 00138 return ledObject; 00139 } 00140 00141 private: 00142 M2MObject *ledObject; 00143 }; 00144 00145 static void cbButton() 00146 { 00147 buttonPressed = true; 00148 pulseEvent(); 00149 } 00150 00151 /* This example program for the u-blox C030 board instantiates mbedClient 00152 * and runs it over a UbloxATCellularInterface or a OnboardCellularInterface to 00153 * the mbed connector. 00154 * Progress may be monitored with a serial terminal running at 9600 baud. 00155 * The LED on the C030 board will turn green when this program is 00156 * operating correctly, pulse blue when an mbedClient operation is completed 00157 * and turn red if there is a failure. 00158 * 00159 * Note: mbedClient malloc's around 34 kbytes of RAM, more than is available on 00160 * the C027 platform, hence this example will not run on the C027 platform. 00161 * 00162 * IMPORTANT to use this example you must first register with the mbed Connector: 00163 * 00164 * https://connector.mbed.com/ 00165 * 00166 * ...using your mbed developer credentials and generate your own copy of the file 00167 * security.h, replacing the empty one in this directory with yours and 00168 * recompiling/downloading the code to your board. 00169 */ 00170 00171 int main() 00172 { 00173 INTERFACE_CLASS *interface = new INTERFACE_CLASS(); 00174 // If you need to debug the cellular interface, comment out the 00175 // instantiation above and uncomment the one below. 00176 // For the N2xx interface, change xxx to MBED_CONF_UBLOX_CELL_N2XX_BAUD_RATE, 00177 // while for the non-N2xx interface change it to MBED_CONF_UBLOX_CELL_BAUD_RATE. 00178 // INTERFACE_CLASS *interface = new INTERFACE_CLASS(MDMTXD, MDMRXD, 00179 // xxx, 00180 // true); 00181 MbedClient *mbedClient = new MbedClient(device); 00182 M2MObjectList objectList; 00183 M2MSecurity *registerObject; 00184 M2MDevice *deviceObject; 00185 LedResource ledResource; 00186 unsigned int seed; 00187 size_t len; 00188 InterruptIn userButton(SW0); 00189 00190 // Attach a function to the user button 00191 userButton.rise(&cbButton); 00192 00193 #if MBED_CONF_MBED_TRACE_ENABLE 00194 mbed_trace_init(); 00195 #endif 00196 srand(seed); 00197 00198 // Randomize source port 00199 mbedtls_hardware_poll(NULL, (unsigned char *) &seed, sizeof seed, &len); 00200 00201 good(); 00202 printf("Starting up, please wait up to 180 seconds for network registration to complete...\n"); 00203 pulseEvent(); 00204 00205 // Create endpoint interface to manage register and unregister 00206 mbedClient->create_interface("coap://api.connector.mbed.com:5684", interface); 00207 00208 // Create objects of varying types, see simpleclient.h for more details on implementation. 00209 registerObject = mbedClient->create_register_object(); // Server object specifying connector info 00210 deviceObject = mbedClient->create_device_object(); // Device resources object 00211 00212 // Add objects to list 00213 objectList.push_back(deviceObject); 00214 objectList.push_back(ledResource.get_object()); 00215 00216 // Set endpoint registration object 00217 mbedClient->set_register_object(registerObject); 00218 00219 printf("Updating object registration in a loop (with a 30 second refresh period) until the user button is presed...\n"); 00220 interface->set_credentials(APN, USERNAME, PASSWORD); 00221 while (!buttonPressed) { 00222 // Make sure cellular is connected 00223 if (interface->connect(PIN) == 0) { 00224 pulseEvent(); 00225 printf("[Still] connected to packet network.\n"); 00226 if (mbedClient->register_successful()) { 00227 printf("Updating registration (follow progress at https://connector.mbed.com/#home)...\n"); 00228 mbedClient->test_update_register(); 00229 } else { 00230 printf("Registering with connector (follow progress at https://connector.mbed.com/#home)...\n"); 00231 mbedClient->test_register(registerObject, objectList); 00232 } 00233 } else { 00234 bad(); 00235 printf("Failed to connect, will retry (have you checked that an antenna is plugged in and your APN is correct?)...\n"); 00236 } 00237 Thread::wait(30000); 00238 printf("[Checking if user button has been pressed]\n"); 00239 } 00240 00241 pulseEvent(); 00242 printf("User button was pressed, stopping...\n"); 00243 mbedClient->test_unregister(); 00244 interface->disconnect(); 00245 ledOff(); 00246 printf("Stopped.\n"); 00247 M2MDevice::delete_instance(); 00248 } 00249 00250 // End Of File
Generated on Tue Jul 12 2022 21:01:19 by
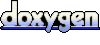