Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <algorithm> 00002 #include "mbed.h" 00003 #include "ESP8266Interface.h" 00004 #include "TCPSocket.h" 00005 #include "greentea-client/test_env.h" 00006 #include "unity/unity.h" 00007 #include "utest.h" 00008 00009 using namespace utest::v1; 00010 00011 #ifndef MBED_CFG_ESP8266_TX 00012 #define MBED_CFG_ESP8266_TX D1 00013 #endif 00014 00015 #ifndef MBED_CFG_ESP8266_RX 00016 #define MBED_CFG_ESP8266_RX D0 00017 #endif 00018 00019 #ifndef MBED_CFG_ESP8266_DEBUG 00020 #define MBED_CFG_ESP8266_DEBUG false 00021 #endif 00022 00023 #define STRINGIZE(x) STRINGIZE2(x) 00024 #define STRINGIZE2(x) #x 00025 00026 00027 namespace { 00028 // Test connection information 00029 const char *HTTP_SERVER_NAME = "developer.mbed.org"; 00030 const char *HTTP_SERVER_FILE_PATH = "/media/uploads/mbed_official/hello.txt"; 00031 const int HTTP_SERVER_PORT = 80; 00032 #if defined(TARGET_VK_RZ_A1H) 00033 const int RECV_BUFFER_SIZE = 300; 00034 #else 00035 const int RECV_BUFFER_SIZE = 512; 00036 #endif 00037 // Test related data 00038 const char *HTTP_OK_STR = "200 OK"; 00039 const char *HTTP_HELLO_STR = "Hello world!"; 00040 00041 // Test buffers 00042 char buffer[RECV_BUFFER_SIZE] = {0}; 00043 } 00044 00045 bool find_substring(const char *first, const char *last, const char *s_first, const char *s_last) { 00046 const char *f = std::search(first, last, s_first, s_last); 00047 return (f != last); 00048 } 00049 00050 void test_tcp_hello_world() { 00051 bool result = false; 00052 ESP8266Interface net(MBED_CFG_ESP8266_TX, MBED_CFG_ESP8266_RX, MBED_CFG_ESP8266_DEBUG); 00053 net.connect(STRINGIZE(MBED_CFG_ESP8266_SSID), STRINGIZE(MBED_CFG_ESP8266_PASS)); 00054 printf("TCP client IP Address is %s\r\n", net.get_ip_address()); 00055 00056 TCPSocket sock(&net); 00057 printf("HTTP: Connection to %s:%d\r\n", HTTP_SERVER_NAME, HTTP_SERVER_PORT); 00058 if (sock.connect(HTTP_SERVER_NAME, HTTP_SERVER_PORT) == 0) { 00059 printf("HTTP: OK\r\n"); 00060 00061 // We are constructing GET command like this: 00062 // GET http://developer.mbed.org/media/uploads/mbed_official/hello.txt HTTP/1.0\n\n 00063 strcpy(buffer, "GET http://"); 00064 strcat(buffer, HTTP_SERVER_NAME); 00065 strcat(buffer, HTTP_SERVER_FILE_PATH); 00066 strcat(buffer, " HTTP/1.0\n\n"); 00067 // Send GET command 00068 sock.send(buffer, strlen(buffer)); 00069 00070 // Server will respond with HTTP GET's success code 00071 const int ret = sock.recv(buffer, sizeof(buffer) - 1); 00072 buffer[ret] = '\0'; 00073 00074 // Find 200 OK HTTP status in reply 00075 bool found_200_ok = find_substring(buffer, buffer + ret, HTTP_OK_STR, HTTP_OK_STR + strlen(HTTP_OK_STR)); 00076 // Find "Hello World!" string in reply 00077 bool found_hello = find_substring(buffer, buffer + ret, HTTP_HELLO_STR, HTTP_HELLO_STR + strlen(HTTP_HELLO_STR)); 00078 00079 TEST_ASSERT_TRUE(found_200_ok); 00080 TEST_ASSERT_TRUE(found_hello); 00081 00082 if (found_200_ok && found_hello) result = true; 00083 00084 printf("HTTP: Received %d chars from server\r\n", ret); 00085 printf("HTTP: Received 200 OK status ... %s\r\n", found_200_ok ? "[OK]" : "[FAIL]"); 00086 printf("HTTP: Received '%s' status ... %s\r\n", HTTP_HELLO_STR, found_hello ? "[OK]" : "[FAIL]"); 00087 printf("HTTP: Received message:\r\n"); 00088 printf("%s", buffer); 00089 sock.close(); 00090 } else { 00091 printf("HTTP: ERROR\r\n"); 00092 } 00093 00094 net.disconnect(); 00095 TEST_ASSERT_EQUAL(true, result); 00096 } 00097 00098 00099 // Test setup 00100 utest::v1::status_t test_setup(const size_t number_of_cases) { 00101 GREENTEA_SETUP(120, "default_auto"); 00102 return verbose_test_setup_handler(number_of_cases); 00103 } 00104 00105 Case cases[] = { 00106 Case("TCP hello world", test_tcp_hello_world), 00107 }; 00108 00109 Specification specification(test_setup, cases); 00110 00111 int main() { 00112 return !Harness::run(specification); 00113 } 00114
Generated on Tue Jul 12 2022 15:14:29 by
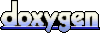