
portable version of the cumulocity demo
Dependencies: C027_Support C12832 LM75B MMA7660 MbedSmartRest mbed-rtos mbed
Fork of MbedSmartRestMain by
LocationUpdate.cpp
00001 #include "LocationUpdate.h" 00002 #include "Aggregator.h" 00003 #include "ComposedRecord.h" 00004 #include "CharValue.h" 00005 #include "IntegerValue.h" 00006 #include "FloatValue.h" 00007 00008 LocationUpdate::LocationUpdate(SmartRest& client, SmartRestTemplate& tpl, long& deviceId, GPSTracker& gpsTracker) : 00009 _client(client), 00010 _tpl(tpl), 00011 _deviceId(deviceId), 00012 _gpsTracker(gpsTracker) 00013 { 00014 _init = false; 00015 } 00016 00017 bool LocationUpdate::init() 00018 { 00019 if (_init) 00020 return false; 00021 00022 // Update device position 00023 // USAGE: 108,<DEVICE/ID>,<ALTITUDE>,<LATITUDE>,<LONGITUDE> 00024 if (!_tpl.add("10,108,PUT,/inventory/managedObjects/%%,application/vnd.com.nsn.cumulocity.managedObject+json,application/vnd.com.nsn.cumulocity.managedObject+json,%%,UNSIGNED NUMBER NUMBER NUMBER,\"{\"\"c8y_Position\"\":{\"\"alt\"\":%%,\"\"lat\"\":%%,\"\"lng\"\":%%},\"\"c8y_MotionTracking\"\":{\"\"active\"\":true}}\"\r\n")) 00025 return false; 00026 00027 // Insert measurement 00028 // USAGE: 109,<DEVICE/ID>,<ALTITUDE>,<LATITUDE>,<LONGITUDE> 00029 if (!_tpl.add("10,109,POST,/event/events,application/vnd.com.nsn.cumulocity.event+json,application/vnd.com.nsn.cumulocity.event+json,%%,NOW UNSIGNED NUMBER NUMBER NUMBER,\"{\"\"time\"\":\"\"%%\"\",\"\"source\"\":{\"\"id\"\":\"\"%%\"\"},\"\"type\"\":\"\"c8y_LocationUpdate\"\",\"\"text\"\":\"\"Mbed location update\"\",\"\"c8y_Position\"\":{\"\"alt\"\":%%,\"\"lat\"\":%%,\"\"lng\"\":%%}}\"")) 00030 return false; 00031 00032 _init = true; 00033 return true; 00034 } 00035 00036 bool LocationUpdate::run() 00037 { 00038 GPSTracker::Position position; 00039 00040 if (!_gpsTracker.position(&position)) { 00041 puts("No GPS data available."); 00042 return true; 00043 } 00044 00045 puts("Starting measurement sending."); 00046 00047 Aggregator aggregator; 00048 ComposedRecord record1, record2; 00049 IntegerValue msgId1(108); 00050 IntegerValue msgId2(109); 00051 IntegerValue devId(_deviceId); 00052 FloatValue altitude(position.altitude, 2); 00053 FloatValue latitude(position.latitude, 6); 00054 FloatValue longitude(position.longitude, 6); 00055 if ((!record1.add(msgId1)) || (!record1.add(devId)) || (!record1.add(altitude)) || (!record1.add(latitude)) || (!record1.add(longitude))) 00056 return false; 00057 if ((!record2.add(msgId2)) || (!record2.add(devId)) || (!record2.add(altitude)) || (!record2.add(latitude)) || (!record2.add(longitude))) 00058 return false; 00059 if ((!aggregator.add(record1)) || (!aggregator.add(record2))) 00060 return false; 00061 00062 puts("Sending GPS measurement."); 00063 if (_client.send(aggregator) != SMARTREST_SUCCESS) { 00064 puts("Signal measurement failed."); 00065 _client.stop(); 00066 return false; 00067 } 00068 00069 _client.stop(); 00070 return true; 00071 }
Generated on Tue Jul 12 2022 21:10:53 by
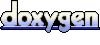