
Version of HelloMQTT with u-blox cellular (C027 and C030) boards added.
Dependencies: C12832 MQTT easy-connect ublox-at-cellular-interface-ext ublox-cellular-base ublox-cellular-driver-gen ublox-ppp-cellular-interface ublox-at-cellular-interface-n2xx ublox-cellular-base-n2xx
Fork of HelloMQTT by
main.cpp
00001 /******************************************************************************* 00002 * Copyright (c) 2014, 2015 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 * Ian Craggs - make sure QoS2 processing works, and add device headers 00016 *******************************************************************************/ 00017 00018 /** 00019 This is a sample program to illustrate the use of the MQTT Client library 00020 on the mbed platform. The Client class requires two classes which mediate 00021 access to system interfaces for networking and timing. As long as these two 00022 classes provide the required public programming interfaces, it does not matter 00023 what facilities they use underneath. In this program, they use the mbed 00024 system libraries. 00025 00026 */ 00027 00028 // change this to 0 to output messages to serial instead of LCD 00029 #define USE_LCD 0 00030 00031 #if USE_LCD 00032 #include "C12832.h" 00033 00034 // the actual pins are defined in mbed_app.json and can be overridden per target 00035 C12832 lcd(LCD_MOSI, LCD_SCK, LCD_MISO, LCD_A0, LCD_NCS); 00036 00037 #define logMessage lcd.cls();lcd.printf 00038 00039 #else 00040 00041 #define logMessage printf 00042 00043 #endif 00044 00045 #define MQTTCLIENT_QOS2 1 00046 00047 #include "easy-connect.h" 00048 #include "MQTTNetwork.h" 00049 #include "MQTTmbed.h" 00050 #include "MQTTClient.h" 00051 00052 int arrivedcount = 0; 00053 00054 00055 void messageArrived(MQTT::MessageData& md) 00056 { 00057 MQTT::Message &message = md.message; 00058 logMessage("Message arrived: qos %d, retained %d, dup %d, packetid %d\r\n", message.qos, message.retained, message.dup, message.id); 00059 logMessage("Payload %.*s\r\n", message.payloadlen, (char*)message.payload); 00060 ++arrivedcount; 00061 } 00062 00063 00064 int main(int argc, char* argv[]) 00065 { 00066 float version = 0.6; 00067 char* topic = "mbed-sample"; 00068 00069 logMessage("HelloMQTT: version is %.2f\r\n", version); 00070 00071 NetworkInterface* network = easy_connect(true); 00072 if (!network) { 00073 return -1; 00074 } 00075 00076 MQTTNetwork mqttNetwork(network); 00077 00078 MQTT::Client<MQTTNetwork, Countdown> client = MQTT::Client<MQTTNetwork, Countdown>(mqttNetwork); 00079 00080 const char* hostname = "m2m.eclipse.org"; 00081 int port = 1883; 00082 logMessage("Connecting to %s:%d\r\n", hostname, port); 00083 int rc = mqttNetwork.connect(hostname, port); 00084 if (rc != 0) 00085 logMessage("rc from TCP connect is %d\r\n", rc); 00086 00087 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00088 data.MQTTVersion = 3; 00089 data.clientID.cstring = "mbed-sample"; 00090 data.username.cstring = "testuser"; 00091 data.password.cstring = "testpassword"; 00092 if ((rc = client.connect(data)) != 0) 00093 logMessage("rc from MQTT connect is %d\r\n", rc); 00094 00095 if ((rc = client.subscribe(topic, MQTT::QOS2, messageArrived)) != 0) 00096 logMessage("rc from MQTT subscribe is %d\r\n", rc); 00097 00098 MQTT::Message message; 00099 00100 // QoS 0 00101 char buf[100]; 00102 sprintf(buf, "Hello World! QoS 0 message from app version %f\r\n", version); 00103 message.qos = MQTT::QOS0; 00104 message.retained = false; 00105 message.dup = false; 00106 message.payload = (void*)buf; 00107 message.payloadlen = strlen(buf)+1; 00108 rc = client.publish(topic, message); 00109 while (arrivedcount < 1) 00110 client.yield(100); 00111 00112 // QoS 1 00113 sprintf(buf, "Hello World! QoS 1 message from app version %f\r\n", version); 00114 message.qos = MQTT::QOS1; 00115 message.payloadlen = strlen(buf)+1; 00116 rc = client.publish(topic, message); 00117 while (arrivedcount < 2) 00118 client.yield(100); 00119 00120 // QoS 2 00121 sprintf(buf, "Hello World! QoS 2 message from app version %f\r\n", version); 00122 message.qos = MQTT::QOS2; 00123 message.payloadlen = strlen(buf)+1; 00124 rc = client.publish(topic, message); 00125 while (arrivedcount < 3) 00126 client.yield(100); 00127 00128 if ((rc = client.unsubscribe(topic)) != 0) 00129 logMessage("rc from unsubscribe was %d\r\n", rc); 00130 00131 if ((rc = client.disconnect()) != 0) 00132 logMessage("rc from disconnect was %d\r\n", rc); 00133 00134 mqttNetwork.disconnect(); 00135 00136 logMessage("Version %.2f: finish %d msgs\r\n", version, arrivedcount); 00137 00138 return 0; 00139 }
Generated on Tue Jul 12 2022 21:12:58 by
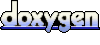