
This example establishes a transparent link between the mbed USB CDC port and the modem (LISA) on the C027. You can use it to use the standard u-blox tools such as m-center or any terminal program. These tools can then connect to the serial port and talk directly to the modem. Baudrate should be set to 115200 baud and is fixed. m-center can be downloaded from u-blox website following this link: http://www.u-blox.com/en/evaluation-tools-a-software/u-center/m-center.html
Dependencies: C027 UbloxUSBModem mbed
Fork of C027_ModemTransparentSerial by
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 #include "WANDongle.h" 00004 #include "USBSerialStream.h" 00005 #include "UbloxCDMAModemInitializer.h" 00006 #include "UbloxGSMModemInitializer.h" 00007 00008 int main() 00009 { 00010 C027 c027; 00011 c027.mdmUsbEnable(true); 00012 c027.mdmPower(true); 00013 00014 // open the mdm serial port 00015 WANDongle mdmDongle; 00016 USBSerialStream mdmStream(mdmDongle.getSerial(1/* the CDC usually 0 or 1, LISA-C requires CDC1*/)); 00017 USBHost* host = USBHost::getHostInst(); 00018 mdmDongle.addInitializer(new UbloxCDMAModemInitializer(host)); 00019 mdmDongle.addInitializer(new UbloxGSMModemInitializer(host)); 00020 00021 // open the PC serial port and (use the same baudrate) 00022 Serial pc(USBTX, USBRX); 00023 pc.baud(MDMBAUD); 00024 00025 while (1) 00026 { 00027 uint8_t buf[64]; 00028 size_t len; 00029 int i; 00030 if (!mdmDongle.connected()) 00031 { 00032 mdmDongle.tryConnect(); 00033 } 00034 else 00035 { 00036 // transfer data from pc to modem 00037 len = mdmStream.space(); 00038 if (len>0) 00039 { 00040 if (len > sizeof(buf)) 00041 len = sizeof(buf); 00042 for (i = 0; (i < len) && pc.readable(); ) 00043 buf[i++] = pc.getc(); 00044 if (OK == mdmStream.write(buf, i)) 00045 /* what to do here ?*/; 00046 } 00047 // transfer data from modem to pc 00048 len = mdmStream.available(); 00049 if ((len>0) && pc.writeable()) 00050 { 00051 if (len > sizeof(buf)) 00052 len = sizeof(buf); 00053 if (OK == mdmStream.read(buf, &len, len)) 00054 { 00055 for (i = 0; (i < len); ) 00056 pc.putc(buf[i++]); 00057 } 00058 } 00059 } 00060 } 00061 }
Generated on Thu Jul 14 2022 14:01:55 by
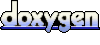