mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ecjpake.h
Go to the documentation of this file.
00001 /** 00002 * \file ecjpake.h 00003 * 00004 * \brief Elliptic curve J-PAKE 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ECJPAKE_H 00024 #define MBEDTLS_ECJPAKE_H 00025 00026 /* 00027 * J-PAKE is a password-authenticated key exchange that allows deriving a 00028 * strong shared secret from a (potentially low entropy) pre-shared 00029 * passphrase, with forward secrecy and mutual authentication. 00030 * https://en.wikipedia.org/wiki/Password_Authenticated_Key_Exchange_by_Juggling 00031 * 00032 * This file implements the Elliptic Curve variant of J-PAKE, 00033 * as defined in Chapter 7.4 of the Thread v1.0 Specification, 00034 * available to members of the Thread Group http://threadgroup.org/ 00035 * 00036 * As the J-PAKE algorithm is inherently symmetric, so is our API. 00037 * Each party needs to send its first round message, in any order, to the 00038 * other party, then each sends its second round message, in any order. 00039 * The payloads are serialized in a way suitable for use in TLS, but could 00040 * also be use outside TLS. 00041 */ 00042 00043 #include "ecp.h" 00044 #include "md.h" 00045 00046 #ifdef __cplusplus 00047 extern "C" { 00048 #endif 00049 00050 /** 00051 * Roles in the EC J-PAKE exchange 00052 */ 00053 typedef enum { 00054 MBEDTLS_ECJPAKE_CLIENT = 0, /**< Client */ 00055 MBEDTLS_ECJPAKE_SERVER, /**< Server */ 00056 } mbedtls_ecjpake_role; 00057 00058 /** 00059 * EC J-PAKE context structure. 00060 * 00061 * J-PAKE is a symmetric protocol, except for the identifiers used in 00062 * Zero-Knowledge Proofs, and the serialization of the second message 00063 * (KeyExchange) as defined by the Thread spec. 00064 * 00065 * In order to benefit from this symmetry, we choose a different naming 00066 * convetion from the Thread v1.0 spec. Correspondance is indicated in the 00067 * description as a pair C: <client name>, S: <server name> 00068 */ 00069 typedef struct 00070 { 00071 const mbedtls_md_info_t *md_info; /**< Hash to use */ 00072 mbedtls_ecp_group grp; /**< Elliptic curve */ 00073 mbedtls_ecjpake_role role; /**< Are we client or server? */ 00074 int point_format; /**< Format for point export */ 00075 00076 mbedtls_ecp_point Xm1; /**< My public key 1 C: X1, S: X3 */ 00077 mbedtls_ecp_point Xm2; /**< My public key 2 C: X2, S: X4 */ 00078 mbedtls_ecp_point Xp1; /**< Peer public key 1 C: X3, S: X1 */ 00079 mbedtls_ecp_point Xp2; /**< Peer public key 2 C: X4, S: X2 */ 00080 mbedtls_ecp_point Xp; /**< Peer public key C: Xs, S: Xc */ 00081 00082 mbedtls_mpi xm1; /**< My private key 1 C: x1, S: x3 */ 00083 mbedtls_mpi xm2; /**< My private key 2 C: x2, S: x4 */ 00084 00085 mbedtls_mpi s; /**< Pre-shared secret (passphrase) */ 00086 } mbedtls_ecjpake_context; 00087 00088 /** 00089 * \brief Initialize a context 00090 * (just makes it ready for setup() or free()). 00091 * 00092 * \param ctx context to initialize 00093 */ 00094 void mbedtls_ecjpake_init( mbedtls_ecjpake_context *ctx ); 00095 00096 /** 00097 * \brief Set up a context for use 00098 * 00099 * \note Currently the only values for hash/curve allowed by the 00100 * standard are MBEDTLS_MD_SHA256/MBEDTLS_ECP_DP_SECP256R1. 00101 * 00102 * \param ctx context to set up 00103 * \param role Our role: client or server 00104 * \param hash hash function to use (MBEDTLS_MD_XXX) 00105 * \param curve elliptic curve identifier (MBEDTLS_ECP_DP_XXX) 00106 * \param secret pre-shared secret (passphrase) 00107 * \param len length of the shared secret 00108 * 00109 * \return 0 if successfull, 00110 * a negative error code otherwise 00111 */ 00112 int mbedtls_ecjpake_setup( mbedtls_ecjpake_context *ctx, 00113 mbedtls_ecjpake_role role, 00114 mbedtls_md_type_t hash, 00115 mbedtls_ecp_group_id curve, 00116 const unsigned char *secret, 00117 size_t len ); 00118 00119 /* 00120 * \brief Check if a context is ready for use 00121 * 00122 * \param ctx Context to check 00123 * 00124 * \return 0 if the context is ready for use, 00125 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA otherwise 00126 */ 00127 int mbedtls_ecjpake_check( const mbedtls_ecjpake_context *ctx ); 00128 00129 /** 00130 * \brief Generate and write the first round message 00131 * (TLS: contents of the Client/ServerHello extension, 00132 * excluding extension type and length bytes) 00133 * 00134 * \param ctx Context to use 00135 * \param buf Buffer to write the contents to 00136 * \param len Buffer size 00137 * \param olen Will be updated with the number of bytes written 00138 * \param f_rng RNG function 00139 * \param p_rng RNG parameter 00140 * 00141 * \return 0 if successfull, 00142 * a negative error code otherwise 00143 */ 00144 int mbedtls_ecjpake_write_round_one( mbedtls_ecjpake_context *ctx, 00145 unsigned char *buf, size_t len, size_t *olen, 00146 int (*f_rng)(void *, unsigned char *, size_t), 00147 void *p_rng ); 00148 00149 /** 00150 * \brief Read and process the first round message 00151 * (TLS: contents of the Client/ServerHello extension, 00152 * excluding extension type and length bytes) 00153 * 00154 * \param ctx Context to use 00155 * \param buf Pointer to extension contents 00156 * \param len Extension length 00157 * 00158 * \return 0 if successfull, 00159 * a negative error code otherwise 00160 */ 00161 int mbedtls_ecjpake_read_round_one( mbedtls_ecjpake_context *ctx, 00162 const unsigned char *buf, 00163 size_t len ); 00164 00165 /** 00166 * \brief Generate and write the second round message 00167 * (TLS: contents of the Client/ServerKeyExchange) 00168 * 00169 * \param ctx Context to use 00170 * \param buf Buffer to write the contents to 00171 * \param len Buffer size 00172 * \param olen Will be updated with the number of bytes written 00173 * \param f_rng RNG function 00174 * \param p_rng RNG parameter 00175 * 00176 * \return 0 if successfull, 00177 * a negative error code otherwise 00178 */ 00179 int mbedtls_ecjpake_write_round_two( mbedtls_ecjpake_context *ctx, 00180 unsigned char *buf, size_t len, size_t *olen, 00181 int (*f_rng)(void *, unsigned char *, size_t), 00182 void *p_rng ); 00183 00184 /** 00185 * \brief Read and process the second round message 00186 * (TLS: contents of the Client/ServerKeyExchange) 00187 * 00188 * \param ctx Context to use 00189 * \param buf Pointer to the message 00190 * \param len Message length 00191 * 00192 * \return 0 if successfull, 00193 * a negative error code otherwise 00194 */ 00195 int mbedtls_ecjpake_read_round_two( mbedtls_ecjpake_context *ctx, 00196 const unsigned char *buf, 00197 size_t len ); 00198 00199 /** 00200 * \brief Derive the shared secret 00201 * (TLS: Pre-Master Secret) 00202 * 00203 * \param ctx Context to use 00204 * \param buf Buffer to write the contents to 00205 * \param len Buffer size 00206 * \param olen Will be updated with the number of bytes written 00207 * \param f_rng RNG function 00208 * \param p_rng RNG parameter 00209 * 00210 * \return 0 if successfull, 00211 * a negative error code otherwise 00212 */ 00213 int mbedtls_ecjpake_derive_secret( mbedtls_ecjpake_context *ctx, 00214 unsigned char *buf, size_t len, size_t *olen, 00215 int (*f_rng)(void *, unsigned char *, size_t), 00216 void *p_rng ); 00217 00218 /** 00219 * \brief Free a context's content 00220 * 00221 * \param ctx context to free 00222 */ 00223 void mbedtls_ecjpake_free( mbedtls_ecjpake_context *ctx ); 00224 00225 #if defined(MBEDTLS_SELF_TEST) 00226 /** 00227 * \brief Checkup routine 00228 * 00229 * \return 0 if successful, or 1 if a test failed 00230 */ 00231 int mbedtls_ecjpake_self_test( int verbose ); 00232 #endif 00233 00234 #ifdef __cplusplus 00235 } 00236 #endif 00237 00238 #endif /* ecjpake.h */
Generated on Tue Jul 12 2022 12:52:43 by
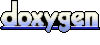