The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
FP< retT, argT > Class Template Reference
API for managing Function Pointers. More...
#include <FP.h>
Public Member Functions | |
FP () | |
Create the FP object - only one callback can be attached to the object, that is a member function or a global function, not both at the same time. | |
template<class T > | |
void | attach (T *item, retT(T::*method)(argT)) |
Add a callback function to the object. | |
void | attach (retT(*function)(argT)) |
Add a callback function to the object. | |
retT | operator() (argT arg) const |
Invoke the function attached to the class. | |
bool | attached () |
Determine if an callback is currently hooked. | |
void | detach () |
Release a function from the callback hook. |
Detailed Description
template<class retT, class argT>
class FP< retT, argT >
API for managing Function Pointers.
Example using the FP Class with global functions
#include "mbed.h" #include "FP.h" FP<void,bool>fp; DigitalOut myled(LED1); void handler(bool value) { myled = value; return; } int main() { fp.attach(&handler); while(1) { fp(1); wait(0.2); fp(0); wait(0.2); } }
Example using the FP Class with different class member functions
#include "mbed.h" #include "FP.h" FP<void,bool>fp; DigitalOut myled(LED4); class Wrapper { public: Wrapper(){} void handler(bool value) { myled = value; return; } }; int main() { Wrapper wrapped; fp.attach(&wrapped, &Wrapper::handler); while(1) { fp(1); wait(0.2); fp(0); wait(0.2); } }
Example using the FP Class with member FP and member function
#include "mbed.h" #include "FP.h" DigitalOut myled(LED2); class Wrapper { public: Wrapper() { fp.attach(this, &Wrapper::handler); } void handler(bool value) { myled = value; return; } FP<void,bool>fp; }; int main() { Wrapper wrapped; while(1) { wrapped.fp(1); wait(0.2); wrapped.fp(0); wait(0.2); } }
Definition at line 128 of file FP.h.
Constructor & Destructor Documentation
FP | ( | ) |
Member Function Documentation
void attach | ( | T * | item, |
retT(T::*)(argT) | method | ||
) |
void attach | ( | retT(*)(argT) | function ) |
bool attached | ( | ) |
retT operator() | ( | argT | arg ) | const |
Field Documentation
retT(* c_callback)(argT) |
retT(FPtrDummy::* method_callback)(argT) |
Generated on Wed Jul 13 2022 15:49:28 by
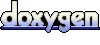