The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
MQTTClient< Network, Timer, MAX_MQTT_PACKET_SIZE, MAX_MESSAGE_HANDLERS > Class Template Reference
Client class for connecting to Cayenne via MQTT. More...
#include <CayenneMQTTClient.h>
Inherits Client< Network, Timer, MAX_MQTT_PACKET_SIZE, 1 >.
Public Member Functions | |
MQTTClient (Network &network, const char *username=NULL, const char *password=NULL, const char *clientID=NULL, unsigned int command_timeout_ms=30000) | |
Create an Cayenne MQTT client object. | |
void | init (const char *username, const char *password, const char *clientID) |
Initialize connection credentials. | |
void | setDefaultMessageHandler (CayenneMessageHandler handler) |
Set default handler function called when a message is received. | |
template<class T > | |
void | setDefaultMessageHandler (T *item, void(T::*handler)(MessageData &)) |
Set default handler function called when a message is received. | |
int | connect () |
Connect to the Cayenne server. | |
int | yield (unsigned long timeout_ms=1000L) |
Yield to allow MQTT message processing. | |
bool | connected () |
Check if the client is connected to the Cayenne server. | |
int | disconnect () |
Disconnect from the Cayenne server. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, int value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, unsigned int value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, long value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, unsigned long value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, double value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, float value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishData (CayenneTopic topic, unsigned int channel, const char *type, const char *unit, const char *value, const char *clientID=NULL) |
Send data to Cayenne. | |
int | publishResponse (const char *id, const char *error, const char *clientID=NULL) |
Send a response to a channel. | |
int | subscribe (CayenneTopic topic, unsigned int channel, CayenneMessageHandler handler=NULL, const char *clientID=NULL) |
Subscribe to a topic. | |
int | unsubscribe (CayenneTopic topic, unsigned int channel, const char *clientID=NULL) |
Unsubscribe from a topic. | |
void | mqttMessageArrived (MQTT::MessageData &md) |
Handler for incoming MQTT::Client messages. | |
Private Member Functions | |
void | setDefaultMessageHandler (T *item, void(T::*method)(MessageData &)) |
Set the default message handling callback - used for any message which does not match a subscription message handler. | |
int | connect (MQTTPacket_connectData &options) |
MQTT Connect - send an MQTT connect packet down the network and wait for a Connack The nework object must be connected to the network endpoint before calling this. | |
int | publish (const char *topicName, Message &message) |
MQTT Publish - send an MQTT publish packet and wait for all acks to complete for all QoSs. | |
int | publish (const char *topicName, void *payload, size_t payloadlen, enum QoS qos=QOS0, bool retained=false) |
MQTT Publish - send an MQTT publish packet and wait for all acks to complete for all QoSs. | |
int | publish (const char *topicName, void *payload, size_t payloadlen, unsigned short &id, enum QoS qos=QOS1, bool retained=false) |
MQTT Publish - send an MQTT publish packet and wait for all acks to complete for all QoSs. | |
int | subscribe (const char *topicFilter, enum QoS qos, messageHandler mh) |
MQTT Subscribe - send an MQTT subscribe packet and wait for the suback. | |
int | unsubscribe (const char *topicFilter) |
MQTT Unsubscribe - send an MQTT unsubscribe packet and wait for the unsuback. | |
bool | isConnected () |
Is the client connected? |
Detailed Description
template<class Network, class Timer, int MAX_MQTT_PACKET_SIZE = CAYENNE_MAX_MESSAGE_SIZE, int MAX_MESSAGE_HANDLERS = 5>
class CayenneMQTT::MQTTClient< Network, Timer, MAX_MQTT_PACKET_SIZE, MAX_MESSAGE_HANDLERS >
Client class for connecting to Cayenne via MQTT.
- Parameters:
-
Network A network class with the methods: read, write. See NetworkInterface.h for function definitions. Timer A timer class with the methods: countdown_ms, countdown, left_ms, expired. See TimerInterface.h for function definitions. MAX_MQTT_PACKET_SIZE Maximum size of an MQTT message, in bytes. MAX_MESSAGE_HANDLERS Maximum number of message handlers.
Definition at line 63 of file CayenneMQTTClient.h.
Constructor & Destructor Documentation
MQTTClient | ( | Network & | network, |
const char * | username = NULL , |
||
const char * | password = NULL , |
||
const char * | clientID = NULL , |
||
unsigned int | command_timeout_ms = 30000 |
||
) |
Create an Cayenne MQTT client object.
- Parameters:
-
[in] network Pointer to an instance of the Network class. Must be connected to the endpoint before calling MQTTClient connect. [in] username Cayenne username [in] password Cayenne password [in] clientID Cayennne client ID [in] command_timeout_ms Timeout for commands in milliseconds.
Definition at line 77 of file CayenneMQTTClient.h.
Member Function Documentation
int connect | ( | ) |
Connect to the Cayenne server.
Connection credentials must be initialized before calling this function.
- Parameters:
-
[in] username Cayenne username [in] password Cayenne password [in] clientID Cayennne client ID
- Returns:
- success code
Reimplemented from Client< Network, Timer, MAX_MQTT_PACKET_SIZE, 1 >.
Definition at line 123 of file CayenneMQTTClient.h.
bool connected | ( | ) |
Check if the client is connected to the Cayenne server.
- Returns:
- true if connected, false if not connected
Definition at line 145 of file CayenneMQTTClient.h.
int disconnect | ( | ) |
Disconnect from the Cayenne server.
- Returns:
- success code
Reimplemented from Client< Network, Timer, MAX_MQTT_PACKET_SIZE, 1 >.
Definition at line 153 of file CayenneMQTTClient.h.
void init | ( | const char * | username, |
const char * | password, | ||
const char * | clientID | ||
) |
Initialize connection credentials.
- Parameters:
-
[in] username Cayenne username [in] password Cayenne password [in] clientID Cayennne client ID
Definition at line 89 of file CayenneMQTTClient.h.
void mqttMessageArrived | ( | MQTT::MessageData & | md ) |
Handler for incoming MQTT::Client messages.
- Parameters:
-
[in] md Message data
Definition at line 383 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
const char * | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 287 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
int | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 167 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
long | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 207 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
unsigned long | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 227 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
double | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 247 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
float | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 267 of file CayenneMQTTClient.h.
int publishData | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | type, | ||
const char * | unit, | ||
unsigned int | value, | ||
const char * | clientID = NULL |
||
) |
Send data to Cayenne.
- Parameters:
-
[in] topic Cayenne topic [in] channel The channel to send data to, or CAYENNE_NO_CHANNEL if there is none [in] type Type to use for a type=value pair, can be NULL if sending to a topic that doesn't require type [in] unit Optional unit to use for a type,unit=value payload, can be NULL [in] value Data value [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 187 of file CayenneMQTTClient.h.
int publishResponse | ( | const char * | id, |
const char * | error, | ||
const char * | clientID = NULL |
||
) |
Send a response to a channel.
- Parameters:
-
[in] id ID of message the response is for [in] error Optional error message, NULL for success [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 309 of file CayenneMQTTClient.h.
void setDefaultMessageHandler | ( | CayenneMessageHandler | handler ) |
Set default handler function called when a message is received.
- Parameters:
-
[in] handler Function called when message is received, if no other handlers exist for the topic.
Reimplemented from Client< Network, Timer, MAX_MQTT_PACKET_SIZE, 1 >.
Definition at line 100 of file CayenneMQTTClient.h.
void setDefaultMessageHandler | ( | T * | item, |
void(T::*)(MessageData &) | handler | ||
) |
Set default handler function called when a message is received.
- Parameters:
-
item Address of initialized object handler Function called when message is received, if no other handlers exist for the topic.
Definition at line 111 of file CayenneMQTTClient.h.
int subscribe | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
CayenneMessageHandler | handler = NULL , |
||
const char * | clientID = NULL |
||
) |
Subscribe to a topic.
- Parameters:
-
[in] topic Cayenne topic [in] channel The topic channel, CAYENNE_NO_CHANNEL for none, CAYENNE_ALL_CHANNELS for all [in] handler The message handler, NULL to use default handler [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with. This string is not copied, so it must remain available for the life of the subscription.
- Returns:
- success code
Definition at line 332 of file CayenneMQTTClient.h.
int unsubscribe | ( | CayenneTopic | topic, |
unsigned int | channel, | ||
const char * | clientID = NULL |
||
) |
Unsubscribe from a topic.
- Parameters:
-
[in] topic Cayenne topic [in] channel The topic channel, CAYENNE_NO_CHANNEL for none, CAYENNE_ALL_CHANNELS for all [in] clientID The client ID to use in the topic, NULL to use the clientID the client was initialized with
- Returns:
- success code
Definition at line 359 of file CayenneMQTTClient.h.
int yield | ( | unsigned long | timeout_ms = 1000L ) |
Yield to allow MQTT message processing.
- Parameters:
-
[in] timeout_ms The time in milliseconds to yield for
- Returns:
- success code
Reimplemented from Client< Network, Timer, MAX_MQTT_PACKET_SIZE, 1 >.
Definition at line 137 of file CayenneMQTTClient.h.
Generated on Wed Jul 13 2022 15:49:29 by
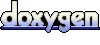