
Re-up
Dependencies: BLE_API mbed nRF51822
main.cpp
00001 // ヘッダのインクルード 00002 #include "mbed.h" 00003 #include "BLEDevice.h" 00004 00005 #define CHARACTERISTIC_LEN 100 00006 00007 // デバイス名の登録 00008 const static char DEVICE_NAME[] = "mbed_HRM1017"; 00009 00010 // UUIDの登録 00011 static const uint8_t UUID_BRIL_SERVICE[] = {0x4d,0x92,0x37,0xc0,0xbd,0x5b,0x45,0x93,0xad,0x55,0xd8,0xf5,0x95,0xcf,0xe2,0xea}; 00012 static const uint8_t UUID_CHAR_DATA[] = {0xe5,0xc1,0xcf,0x6e,0xe0,0x57,0x40,0x08,0x98,0x21,0x17,0x71,0x10,0x24,0xe8,0x85}; 00013 00014 // 00015 uint8_t gRwData[CHARACTERISTIC_LEN] = {0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15}; 00016 int g_conflg = 0; 00017 int g_test = 0; 00018 int g_receiveflg = 0; 00019 uint8_t g_txdata[100]; 00020 uint8_t g_rxdata[100]; 00021 00022 BLEDevice ble; 00023 Serial pc(USBTX, USBRX); 00024 00025 uint8_t thermTempPayload[5] = { 1, 2, 3, 4, 5 }; 00026 00027 GattCharacteristic gDataCharacteristic ( UUID_CHAR_DATA, gRwData, sizeof(gRwData), sizeof(gRwData), 00028 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE); 00029 00030 GattCharacteristic tempChar( GattCharacteristic::UUID_TEMPERATURE_MEASUREMENT_CHAR, 00031 thermTempPayload, 100, 100, 00032 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE | 00033 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00034 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE ); 00035 00036 // バッテリーレベル用変数 00037 uint8_t batt = 100; // Battery level 00038 uint8_t read_batt = 0; // Variable to hold battery level reads 00039 00040 // バッテリーレベル用GATT 00041 GattCharacteristic battLevel ( GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, 00042 (uint8_t *)batt, 1, 1, 00043 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY | 00044 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | 00045 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE ); 00046 00047 // 00048 GattCharacteristic *htmChars[] = {&tempChar, }; 00049 GattCharacteristic *battChars[] = {&battLevel, }; 00050 GattCharacteristic *RwDataChars[] = {&gDataCharacteristic}; 00051 00052 GattService htmService( GattService::UUID_HEALTH_THERMOMETER_SERVICE, htmChars, 00053 sizeof(htmChars) / sizeof(GattCharacteristic * ) ); 00054 GattService battService( GattService::UUID_BATTERY_SERVICE, battChars, 00055 sizeof(battChars) / sizeof(GattCharacteristic * ) ); 00056 GattService gBrilService = GattService(UUID_BRIL_SERVICE, RwDataChars, sizeof(RwDataChars) / sizeof(GattCharacteristic *)); 00057 00058 uint16_t uuid16_list[] = {GattService::UUID_HEALTH_THERMOMETER_SERVICE, 00059 GattService::UUID_BATTERY_SERVICE}; 00060 00061 static Gap::ConnectionParams_t connectionParams; 00062 00063 void onDisconnectionCallback( Gap::Handle_t handle, Gap::DisconnectionReason_t reason ){ 00064 00065 g_conflg = 0; 00066 ble.startAdvertising(); 00067 pc.printf( "Disconnect!!\r\n" ); 00068 00069 } 00070 00071 void onConnectionCallback( Gap::Handle_t handle, Gap::addr_type_t type, const Gap::address_t addr, 00072 Gap::addr_type_t addr_type_townAddrType, const Gap::address_t ownAddr, 00073 const Gap::ConnectionParams_t *params ){ 00074 00075 g_conflg = 1; 00076 pc.printf( "Connect!!\r\n" ); 00077 00078 } 00079 00080 void onDataWrittenCallback( const GattCharacteristicWriteCBParams *params ){ 00081 int i; 00082 00083 uint16_t bytesread = params->len; 00084 00085 batt--; 00086 if( batt < 0 ){ 00087 batt = 100; 00088 } 00089 00090 pc.printf( "Written %d Bytes.\r\n", bytesread ); 00091 for( i = 0; i < bytesread; i++ ){ 00092 pc.printf( "%02x",params->data[i] ); 00093 if( ( i + 1 ) < bytesread ){ 00094 pc.printf( ", " ); 00095 } 00096 } 00097 pc.printf( "\r\n" ); 00098 00099 if( ( params->data[0] == 0x24 ) && ( params->data[1] == 0x02 ) ){ 00100 g_receiveflg = 1; 00101 } 00102 00103 ble.updateCharacteristicValue( tempChar.getValueAttribute().getHandle(), thermTempPayload, sizeof( thermTempPayload ) ); 00104 // ble.updateCharacteristicValue(battLevel.getHandle(), (uint8_t *)&batt, sizeof(batt)); 00105 } 00106 00107 void create_data(){ 00108 00109 int i; 00110 00111 } 00112 00113 int main( void ) 00114 { 00115 00116 // 初期設定 00117 ble.init(); 00118 // コールバック関数の設定 00119 ble.onDisconnection( onDisconnectionCallback ); 00120 ble.onConnection( onConnectionCallback ); 00121 ble.onDataWritten(onDataWrittenCallback); 00122 00123 ble.getPreferredConnectionParams( &connectionParams ); 00124 00125 ble.accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00126 // ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t*)uuid16_list, sizeof(uuid16_list)); 00127 // ble.accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_THERMOMETER); 00128 ble.accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00129 ble.setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00130 ble.setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00131 ble.setAdvertisingTimeout(0); // アドバタイジングモードのタイムアウト無効 00132 00133 // アドバタイズモード開始 00134 ble.startAdvertising(); 00135 00136 // サービスの追加 00137 ble.addService(htmService); 00138 ble.addService(battService); 00139 ble.addService(gBrilService); 00140 00141 while(1){ 00142 00143 ble.waitForEvent(); 00144 if( g_receiveflg ){ 00145 00146 } 00147 00148 } 00149 }
Generated on Mon Jul 18 2022 15:36:54 by
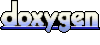