
for interfacing the sparkfun boards
Dependencies: ADXL345_I2C HMC5883L IMUfilter ITG3200_HelloWorld mbed
ADXL345HL.h
00001 #pragma once 00002 #include "ADXL345_I2C.h" 00003 00004 00005 //Gravity at Earth's surface in m/s/s 00006 #define g0 9.812865328 00007 //Number of samples to average. 00008 #define SAMPLES 4 00009 //Convert from radians to degrees. 00010 #define toDegrees(x) (x * 57.2957795) 00011 //Convert from degrees to radians. 00012 #define toRadians(x) (x * 0.01745329252) 00013 //Full scale resolution on the ADXL345 is 4mg/LSB. 00014 #define ACCELEROMETER_GAIN (0.004 * g0) 00015 //Sampling accelerometer at 200Hz. 00016 #define ACC_RATE 0.005 00017 00018 class ADXL345HL 00019 { 00020 private: 00021 ADXL345_I2C* accelerometer; 00022 int readings[3]; 00023 double xBias; 00024 double yBias; 00025 double zBias; 00026 double* output; //x,y,z 00027 char address; //i^2c device address 00028 int calibrationsamples; 00029 int readsamples; 00030 float samplerate; 00031 public: 00032 void init(int calibsamples, int readsampls, float samplrate); 00033 void calibrateAccelerometer(void); 00034 double* sampleAccelerometer(void); 00035 ADXL345HL(); 00036 ~ADXL345HL(); 00037 };
Generated on Tue Jul 12 2022 19:05:53 by
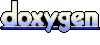