
the fish that looks like a jet
Dependencies: ADXL345 ADXL345_I2C IMUfilter ITG3200 mbed Servo
PwmIn.h
00001 00002 #ifndef MBED_PWMIN_H 00003 #define MBED_PWMIN_H 00004 00005 #include "mbed.h" 00006 00007 /** PwmIn class to read PWM inputs 00008 * 00009 * Uses InterruptIn to measure the changes on the input 00010 * and record the time they occur 00011 * 00012 * @note uses InterruptIn, so not available on p19/p20 00013 */ 00014 class PwmIn { 00015 public: 00016 /** Create a PwmIn 00017 * 00018 * @param p The pwm input pin (must support InterruptIn) 00019 */ 00020 PwmIn(PinName p, float dutyMin, float dutyMax) ; 00021 00022 /** Read the current period 00023 * 00024 * @returns the period in seconds 00025 */ 00026 float period(); 00027 00028 /** Read the current pulsewidth 00029 * 00030 * @returns the pulsewidth in seconds 00031 */ 00032 float pulsewidth(); 00033 00034 /** Read the current dutycycle 00035 * 00036 * @returns the dutycycle as a percentage, represented between 0.0-1.0 00037 */ 00038 float dutycycle(); 00039 00040 float dutycyclescaledup(); 00041 00042 protected: 00043 void rise(); 00044 void fall(); 00045 00046 InterruptIn _p; 00047 Timer _t; 00048 int _pulsewidth, _period; 00049 int _tmp; 00050 float _dutyMin, _dutyMax, _dutyDelta; 00051 00052 bool _risen; 00053 }; 00054 00055 #endif
Generated on Mon Jul 25 2022 21:18:46 by
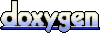