
Example for updating the MTi-1's firmware. Uses a platform independent, retargetable pure C implementation of the firmware updater protocol.
mtinterface_uart.cpp
00001 /*! 00002 * \file 00003 * \copyright Copyright (C) Xsens Technologies B.V., 2015. 00004 * 00005 * Licensed under the Apache License, Version 2.0 (the "License"); you may not 00006 * use this file except in compliance with the License. You may obtain a copy 00007 * of the License at 00008 * 00009 * http://www.apache.org/licenses/LICENSE-2.0 00010 * 00011 * Unless required by applicable law or agreed to in writing, software 00012 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00013 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the 00014 * License for the specific language governing permissions and limitations 00015 * under the License. 00016 */ 00017 #include "mtinterface_uart.h " 00018 #include <assert.h> 00019 #include "board.h " 00020 #include "mbed.h" 00021 00022 00023 /*! \class MtInterfaceUart 00024 \brief Implementation of MtInterface for communicating over UART. 00025 00026 The low level UART driver is provided by the mbed platform. 00027 */ 00028 00029 static MtInterfaceUart* thisPtr = 0; // required to give callback function access to class members 00030 00031 00032 /*! \brief Constructor 00033 \param baudrate Uart baud rate used to communicate with the module 00034 */ 00035 MtInterfaceUart::MtInterfaceUart(int baudrate) 00036 { 00037 assert(thisPtr == 0); //(only one instance is allowed) 00038 thisPtr = this; 00039 m_serial = new Serial(UART_TX_TO_MODULE, UART_RX_FROM_MODULE); 00040 m_serial->baud(baudrate); 00041 m_serial->format(8, Serial::None, 1); 00042 m_serial->attach(uart_rx_interrupt_handler, Serial::RxIrq); 00043 m_txBuffer = new uint8_t[300]; 00044 } 00045 00046 00047 /*! \brief Destructor 00048 */ 00049 MtInterfaceUart::~MtInterfaceUart() 00050 { 00051 delete m_serial; 00052 delete[] m_txBuffer; 00053 thisPtr = 0; 00054 } 00055 00056 00057 /*! \brief Sends an xbus message to the module via UART 00058 \param xbusMessage Xbus message to be send 00059 */ 00060 void MtInterfaceUart::sendXbusMessage(XbusMessage const* xbusMessage) 00061 { 00062 int size = XbusMessage_createRawMessage(m_txBuffer, xbusMessage, XBF_Uart); 00063 for (int n = 0; n < size; n++) 00064 { 00065 m_serial->putc(m_txBuffer[n]); 00066 } 00067 } 00068 00069 00070 /*! \brief Returns the low level bus format used by this interface 00071 */ 00072 XbusBusFormat MtInterfaceUart::busFormat() 00073 { 00074 return XBF_Uart; 00075 } 00076 00077 00078 /*! \brief Handles UART bytes received from the motion tracker 00079 */ 00080 void MtInterfaceUart::uart_rx_interrupt_handler() 00081 { 00082 while (thisPtr->m_serial->readable()) 00083 { 00084 uint8_t c = thisPtr->m_serial->getc(); 00085 XbusParser_parseBuffer(thisPtr->m_xbusParser, &c, 1); 00086 } 00087 } 00088 00089 00090 /*! \brief Set the baudrate 00091 \param baudrate The baudrate value which should be set 00092 */ 00093 void MtInterfaceUart::setBaudrate(int baudrate) 00094 { 00095 m_serial->baud(baudrate); 00096 } 00097
Generated on Wed Jul 13 2022 07:56:15 by
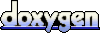